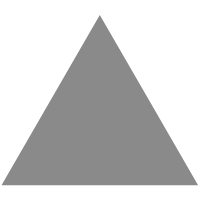
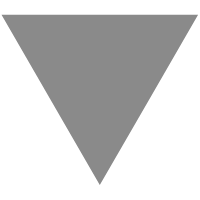
How to generate 6 different random numbers in java
source link: https://www.codesd.com/item/how-to-generate-6-different-random-numbers-in-java.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
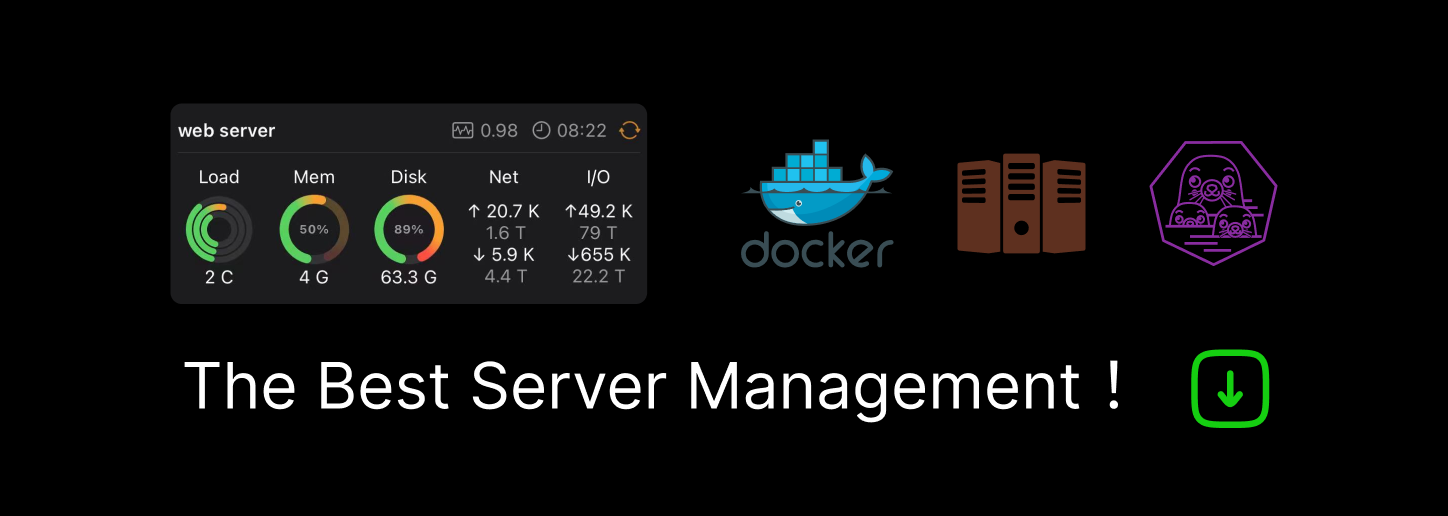
How to generate 6 different random numbers in java
I want to generate 6 different random numbers by using Math.random and store them into an array. How can I make sure that they are different? I know I need to use for-loop to check the array but how...
This is the range. I only need numbers between 1 and 49. ( 1 + (int) (Math.random() * 49) )
In Java 8:
final int[] ints = new Random().ints(1, 50).distinct().limit(6).toArray();
In Java 7:
public static void main(final String[] args) throws Exception {
final Random random = new Random();
final Set<Integer> intSet = new HashSet<>();
while (intSet.size() < 6) {
intSet.add(random.nextInt(49) + 1);
}
final int[] ints = new int[intSet.size()];
final Iterator<Integer> iter = intSet.iterator();
for (int i = 0; iter.hasNext(); ++i) {
ints[i] = iter.next();
}
System.out.println(Arrays.toString(ints));
}
Just a little messier. Not helped by the fact that it's pretty tedious to unbox the Set<Integer>
into an int[]
.
It should be noted that this solution should be fine of the number of required values is significantly smaller than the range. As 1..49
is quite a lot larger than 6
you're fine. Otherwise performance rapidly degrades.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK