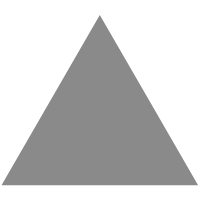
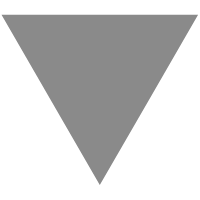
How To Use @HostBinding and @HostListener in Custom Angular Directives
source link: https://www.digitalocean.com/community/tutorials/angular-hostbinding-hostlistener
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
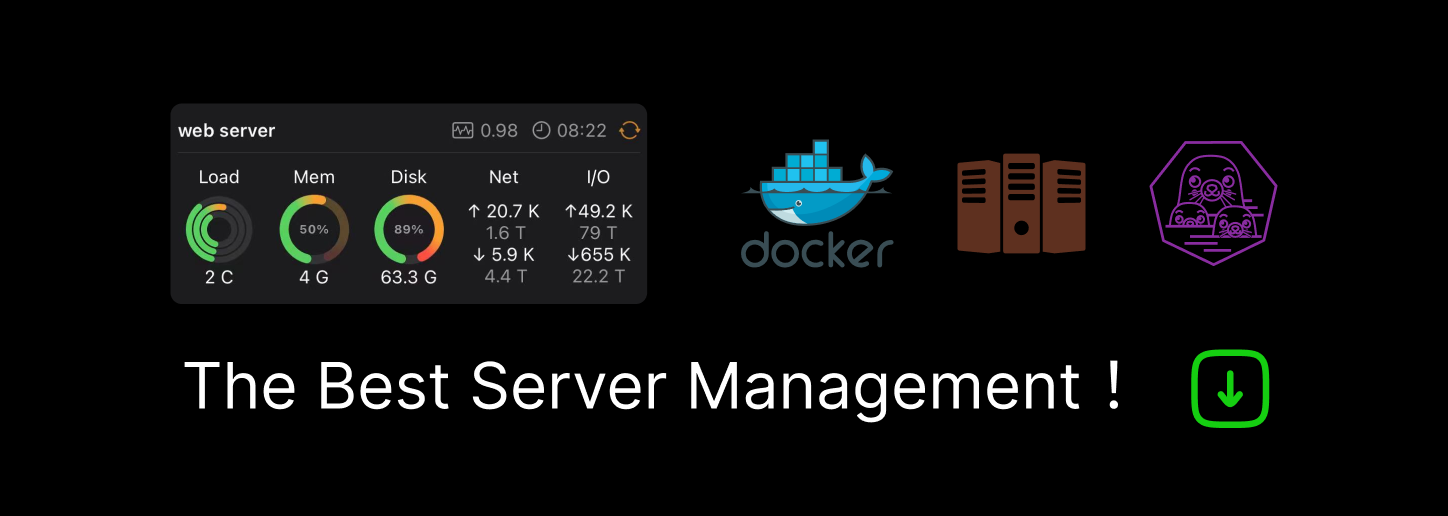
Introduction
@HostBinding
and @HostListener
are two decorators in Angular that can be really useful in custom directives. @HostBinding
lets you set properties on the element or component that hosts the directive, and @HostListener
lets you listen for events on the host element or component.
In this article, you will use @HostBinding
and @HostListener
in an example directive that listens for a keydown
event on the host.
It will set its text and border color to a random color from a set of a few available colors.
Prerequisites
To complete this tutorial, you will need:
This tutorial was verified with Node v16.4.2, npm
v7.18.1, angular
v12.1.1.
Using @HostBinding
and @HostListener
First, create a new RainbowDirective
.
This can be accomplished with @angular/cli
:
ng generate directive rainbow --skip-tests
This will add the new component to the app declarations
and produce a rainbow.directive.ts
file:
import { Directive } from '@angular/core';
@Directive({
selector: '[appRainbow]'
})
export class RainbowDirective {
constructor() { }
}
Add @HostBinding
and @HostListener
:
import { Directive, HostBinding, HostListener } from '@angular/core';
@Directive({
selector: '[appRainbow]'
})
export class RainbowDirective {
possibleColors = [
'darksalmon',
'hotpink',
'lightskyblue',
'goldenrod',
'peachpuff',
'mediumspringgreen',
'cornflowerblue',
'blanchedalmond',
'lightslategrey'
];
@HostBinding('style.color') color!: string;
@HostBinding('style.border-color') borderColor!: string;
@HostListener('keydown') newColor() {
const colorPick = Math.floor(Math.random() * this.possibleColors.length);
this.color = this.borderColor = this.possibleColors[colorPick];
}
}
And the directive can be used on elements like this:
<input type="text" appRainbow />
Our Rainbow
directive uses two @HostBinding
decorators to define two class members, one that’s attached to the host’s style.color
binding and the other to style.border-color
.
You can also bind to any class, property, or attribute of the host.
Here are a few more examples of possible bindings:
@HostBinding('class.active')
@HostBinding('disabled')
@HostBinding('attr.role')
The @HostListener
with the 'keydown'
argument listens for the keydown event on the host. We define a function attached to this decorator that changes the value of color
and borderColor
, and our changes get reflected on the host automatically.
Conclusion
In this article, you used @HostBinding
and @HostListener
in an example directive that listens for a keydown
event on the host.
If you’d like to learn more about Angular, check out our Angular topic page for exercises and programming projects.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK