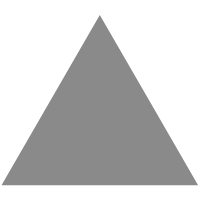
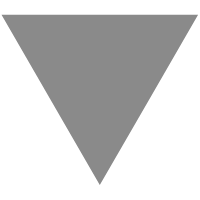
Alternative to AJAX async: false option for form send button click on event hand...
source link: https://www.codesd.com/item/alternative-to-ajax-async-false-option-for-form-send-button-click-on-event-handler.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
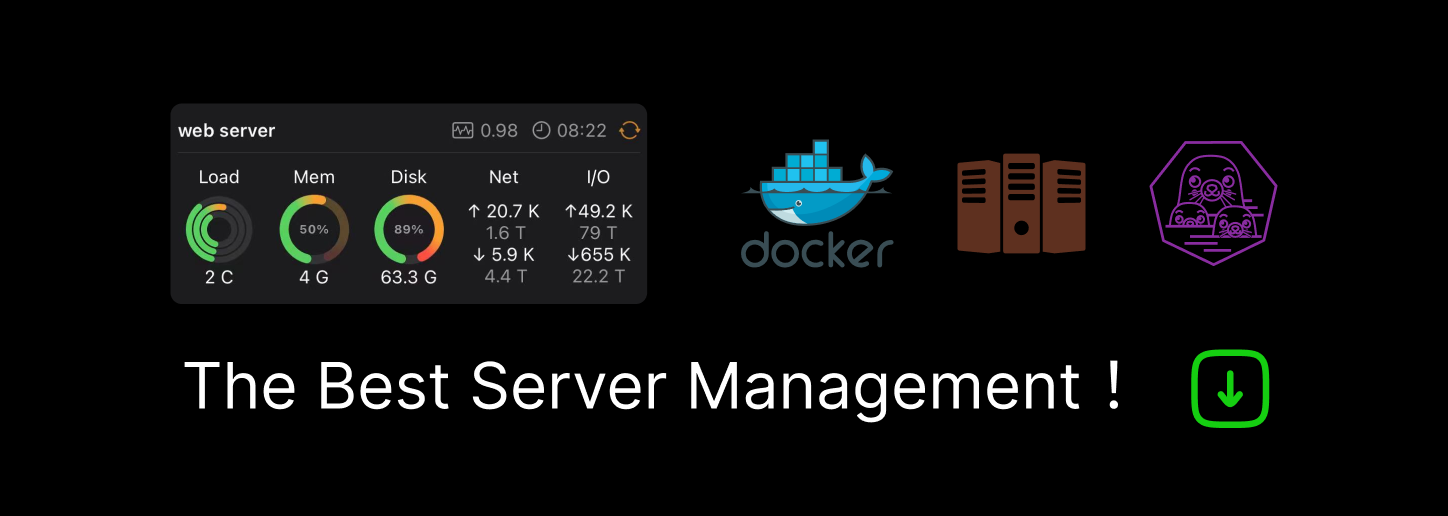
Alternative to AJAX async: false option for form send button click on event handler
I have a login form where we make an AJAX call to the server to perform a bit of validation before letting the login form continue submitting. The current code is outlined below:
(function ($) {
var errorMessageHtml = "";
function isUserValid(username) {
if (username.length <= 0) {
return false;
}
var userIsValid = false;
$.ajax({
async: false,
url: "/myAjaxCall?username=" + username
}).success(function (validationResult) {
userIsValid = validationResult.IsValid;
errorMessageHtml = validationResult.ErrorMessage;
}).fail(function () {
errorMessageHtml = "Error contacting server. Please try again.";
});
return userIsValid;
}
var $usernameTextbox = $("#UserName");
var $errorMessageLabel = $(".errorMessageContainer");
$(".loginButton").on("click", function (e) {
$errorMessageLabel.hide();
if (isUserValid($usernameTextbox.val())) {
return true;
} else {
$errorMessageLabel.show();
$errorMessageLabel.html(errorMessageHtml);
}
e.preventDefault();
return false;
});
})(jQuery);
I know that async: false
is something that shouldn't be used since it's going out of style. My question is: What's the alternative. My click event handler needs to return true or false, meaning it has to wait for the ajax call to complete. If async: false
is no longer an option, then the isUserValid
method is going to return immediately without properly setting the userIsValid
bool.
Now I can inline the ajax method call straight into the click event handler that's called on $(".loginButton")
, but the same problem presents itself: It needs to either return true, or prevent default (i.e. prevent login) and return false depending on the result of the ajax call. Is there a way I can force the click event handler to wait for the result of the ajax call before returning, without using async: false
? I understand there's a jQuery when()
method, but I don't know if I can use that in this situation.
First thing, a form
can be submited without clicking on respective submit button. So bind instead submit
event to the form
. Now depending ajax request result, you can submit the form
, using e.g:
(function ($) {
var errorMessageHtml = "";
function isUserValid(username) {
$errorMessageLabel.hide();
if (username.length <= 0) {
return false;
}
var userIsValid = false;
// return the promise from ajax method
return $.ajax({
url: "/myAjaxCall?username=" + username
}).success(function (validationResult) {
userIsValid = validationResult.IsValid;
errorMessageHtml = validationResult.ErrorMessage;
}).fail(function () {
errorMessageHtml = "Error contacting server. Please try again.";
});
}
var $usernameTextbox = $("#UserName");
var $errorMessageLabel = $(".errorMessageContainer");
// "form:has(.loginButton)" or whatever more relevant selector
$("form:has(.loginButton)").on("submit", function (e) {
$errorMessageLabel.hide();
isUserValid($usernameTextbox.val())).always(function(validationResult ){
if(validationResult && validationResult.IsValid) {
this.submit();
} else {
$errorMessageLabel.html(errorMessageHtml).show();
}
}.bind(this));
e.preventDefault();
});
})(jQuery);
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK