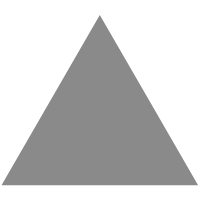
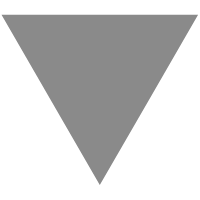
Decrypt multidimensional arrays allocated dynamically.
source link: https://www.codesd.com/item/decrypt-multidimensional-arrays-allocated-dynamically.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
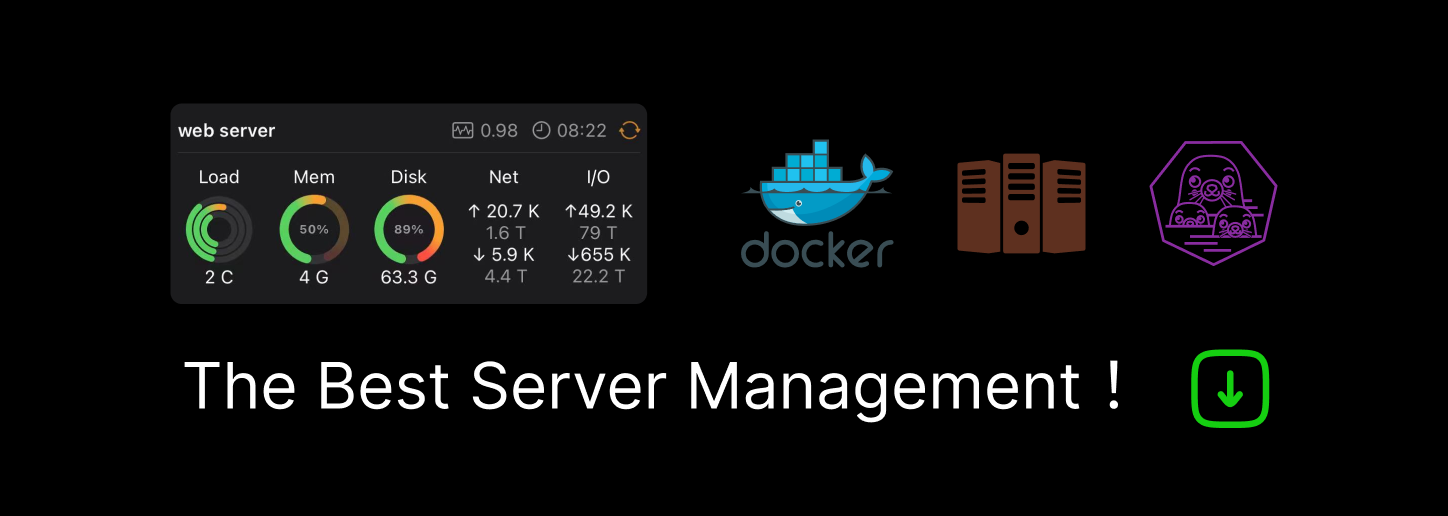
Decrypt multidimensional arrays allocated dynamically.
So, I have this code, and I am trying to deallocate the array ppint
at the end. I have tried using leaks with Xcode to figure out if it is working, but I don't quite understand it. Will doing this work?
delete ppint[0];
delete ppint[1];
delete ppint[2];
delete ppint[3];
Or is there something else that must be done?
#include <iostream>
#include <string>
#include <unistd.h>
using namespace std;
int main()
{
int **ppint;
ppint = new int * [4];
for(int i = 0; i < 4; i++ ) {
ppint [i] = new int[4];
} // declares second layer of arrays
for(int i = 0, count = 0; i < 4; i++ ) {
for(int j = 0; j < 4; j++ ) {
count++;
ppint [i] [j] = count;
} //init part 2
} // init array
for(int i = 0; i < 4; i++ ) {
for(int j = 0; j < 4; j++ ) {
cout << ppint [i] [j] << endl;
} // print part 2
} //print array
}
Close. You would have to use delete[]
because you allocated each of them with new[]
:
delete[] ppint[0];
delete[] ppint[1];
delete[] ppint[2];
delete[] ppint[3];
But of course, you should use a loop:
for(int i = 0; i < 4; i++ ) {
delete[] ppint[i];
}
And then don't forget to delete[]
ppint
itself:
delete[] ppint;
However, in C++, we prefer to not mess around with dynamically allocated arrays. Use a std::vector<std::vector<int>>
or a std::array<std::array<int, 4>, 4>
. If you care about locality of data, try boost::multi_array
.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK