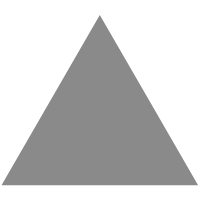
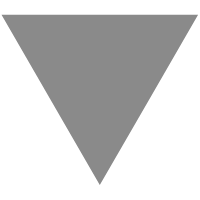
Longest subsequence with a given OR value : Dynamic Programming Approach
source link: https://www.geeksforgeeks.org/longest-subsequence-with-a-given-or-value-dynamic-programming-approach/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
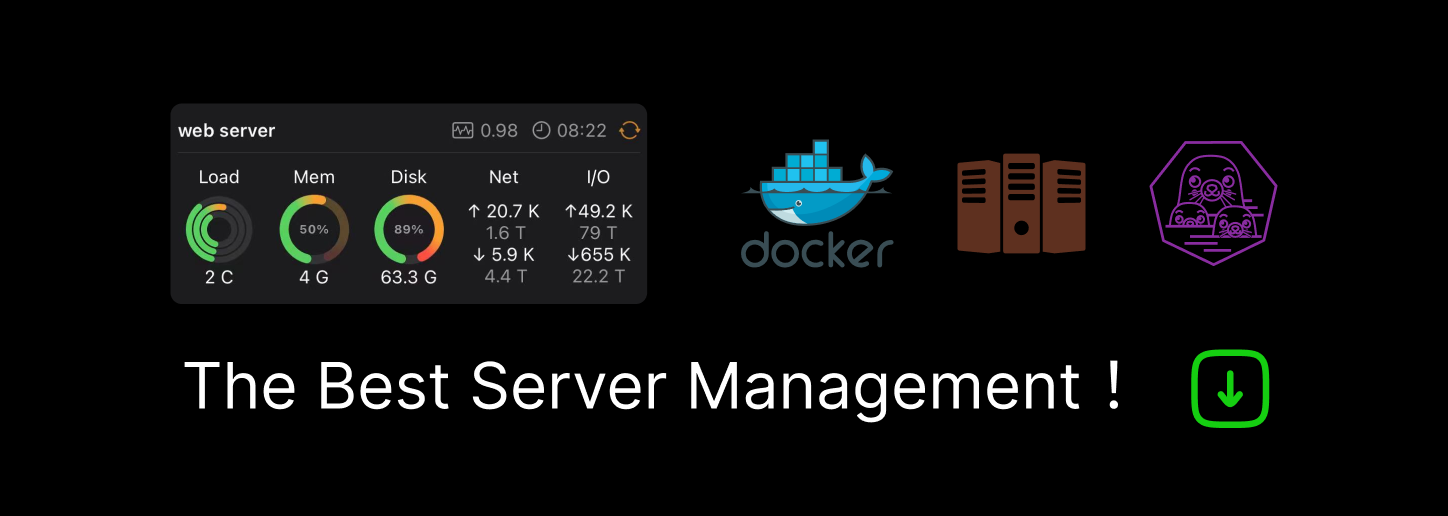
- Last Updated : 13 May, 2021
Given an array arr[], the task is to find the longest subsequence with a given OR value M. If there is no such sub-sequence then print 0.
Examples:
Input: arr[] = {3, 7, 2, 3}, M = 3
Output: 3
{3, 2, 3} is the required subsequence
3 | 2 | 3 = 3
Input: arr[] = {2, 2}, M = 3
Output: 0
Approach: A simple solution is to generate all the possible sub-sequences and then find the largest among them with the required OR value. However, for smaller values of M, a dynamic programming approach can be used.
Let’s look at the recurrence relation first.
dp[i][curr_or] = max(dp[i + 1][curr_or], dp[i + 1][curr_or | arr[i]] + 1)
Let’s understand the states of DP now. Here, dp[i][curr_or] stores the longest subsequence of the subarray arr[i…N-1] such the curr_or gives M when gets ORed with this subsequence. At each step, either choose the index i and update curr_or or reject index i and continue.
Below is the implementation of the above approach:
- Python3
- Javascript
// C++ implementation of the approach
#include <bits/stdc++.h>
using
namespace
std;
#define maxN 20
#define maxM 64
// To store the states of DP
int
dp[maxN][maxM];
bool
v[maxN][maxM];
// Function to return the required length
int
findLen(
int
* arr,
int
i,
int
curr,
int
n,
int
m)
{
// Base case
if
(i == n) {
if
(curr == m)
return
0;
else
return
-1;
}
// If the state has been solved before
// return the value of the state
if
(v[i][curr])
return
dp[i][curr];
// Setting the state as solved
v[i][curr] = 1;
// Recurrence relation
int
l = findLen(arr, i + 1, curr, n, m);
int
r = findLen(arr, i + 1, curr | arr[i], n, m);
dp[i][curr] = l;
if
(r != -1)
dp[i][curr] = max(dp[i][curr], r + 1);
return
dp[i][curr];
}
// Driver code
int
main()
{
int
arr[] = { 3, 7, 2, 3 };
int
n =
sizeof
(arr) /
sizeof
(
int
);
int
m = 3;
int
ans = findLen(arr, 0, 0, n, m);
if
(ans == -1)
cout << 0;
else
cout << ans;
return
0;
}
3
Time Complexity: O(N * maxArr) where maxArr is the maximum element from the array.
Attention reader! Don’t stop learning now. Get hold of all the important DSA concepts with the DSA Self Paced Course at a student-friendly price and become industry ready. To complete your preparation from learning a language to DS Algo and many more, please refer Complete Interview Preparation Course.
In case you wish to attend live classes with industry experts, please refer DSA Live Classes
Recommend
-
8
Longest Palindromic Subsequence in Java In this article, we will look at yet another interesting problem popular in coding interviews. The problem states that Given a String we have to Find the Longest...
-
12
Leetcode 516. Longest Palindromic Subsequence 当前位置:XINDOO > 算法 > 正文
-
1
POJ 2533 - Longest Ordered Subsequence Time: 2000MS Memory: 65536K 难度: 初级 分类: 动态规划 动态规划,求LIS最大不...
-
12
[Golang] Longest Common Subsequence Length via Recursion May 04, 2017...
-
1
Longest common subsequence with permutations allowedSkip to content
-
4
Longest consecutive subsequenceLongest consecutive subsequence | SDE Sheet | HashingHi. In this video, we will learn...
-
2
Longest Bitonic Subsequence (Visualisation)Skip to content
-
7
How Do You Find the Longest Common Subsequence of Two Strings in Java?NotificationsOver 100,000 votes have been casted for this year’s Noonies Nominees. Vote for your favorite now!...
-
1
Longest Palindromic SubsequenceSkip to content
-
4
Set 4 (Longest Common Subsequence)Skip to content
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK