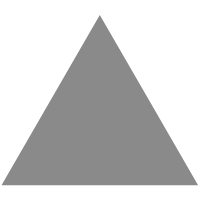
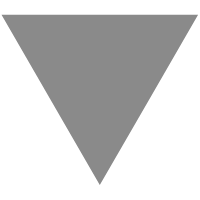
What is the effective method for adding an element to the array in java when the...
source link: https://www.codesd.com/item/what-is-the-effective-method-for-adding-an-element-to-the-array-in-java-when-the-array-size-is-small-not-more-than-5.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
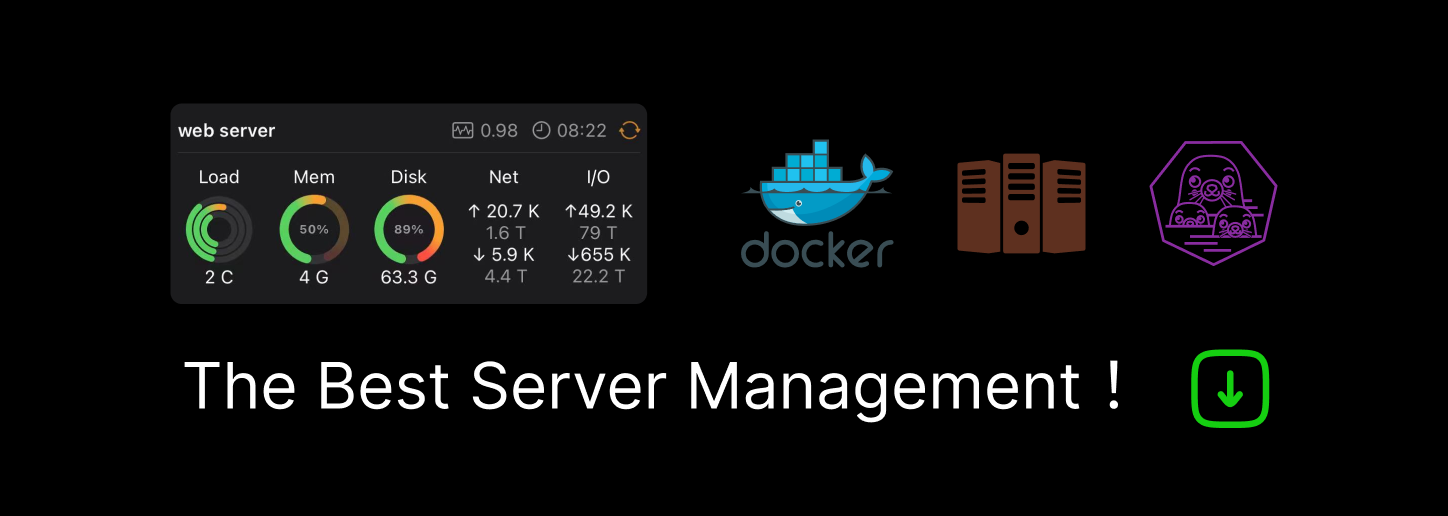
What is the effective method for adding an element to the array in java when the array size is small (not more than 5)
The generally used way of adding item to array is by using ArrayList
variable instead of generic array and calling the add
method.
I wrote this method of adding item to a generic array:
public static <T> T[] addItemToArray(T[] objects, T object) {
T[] temp = null;
temp = Arrays.copyOf(objects, objects.length + 1);
temp[objects.length] = object;
return temp;
}
Which of these method is more efficient in terms of speed and memory management and why?
Additional Information
This method is used in runtime if and when the need arises to add a new item and not just iterate over a loop to populate an array. Also noteworthy is that the size of the array would never exceed 5. Given this case would it be better to use this custom method instead of ArrayList
?
ArrayList.add() will be less memory efficient (in some ways) but faster than your implementation. The reason is that ArrayList.add() does not increase the size of the underlying array with every add operation. It only increases the size sometimes and it increases it by more than 1.
Specifically the array size is increased with the following formula when it runs out of space:
int newCapacity = (oldCapacity * 3)/2 + 1;
This increases the size of the array by roughly 1/2 when it is full.
This means that in most cases ArrayList.add() just puts an item in the array and only sometimes does the more expensive operation of increasing the size of the array. The downside of this is that ArrayList allocates slightly more space than is needed at any one time and this is more than worth it because of the relative expense of increasing the size of the array with every operation.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK