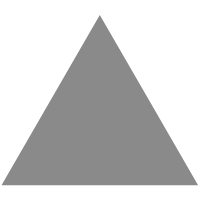
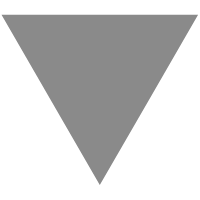
Auxiliary constructor in Scala
source link: https://blog.knoldus.com/auxiliary-constructor-in-scala/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
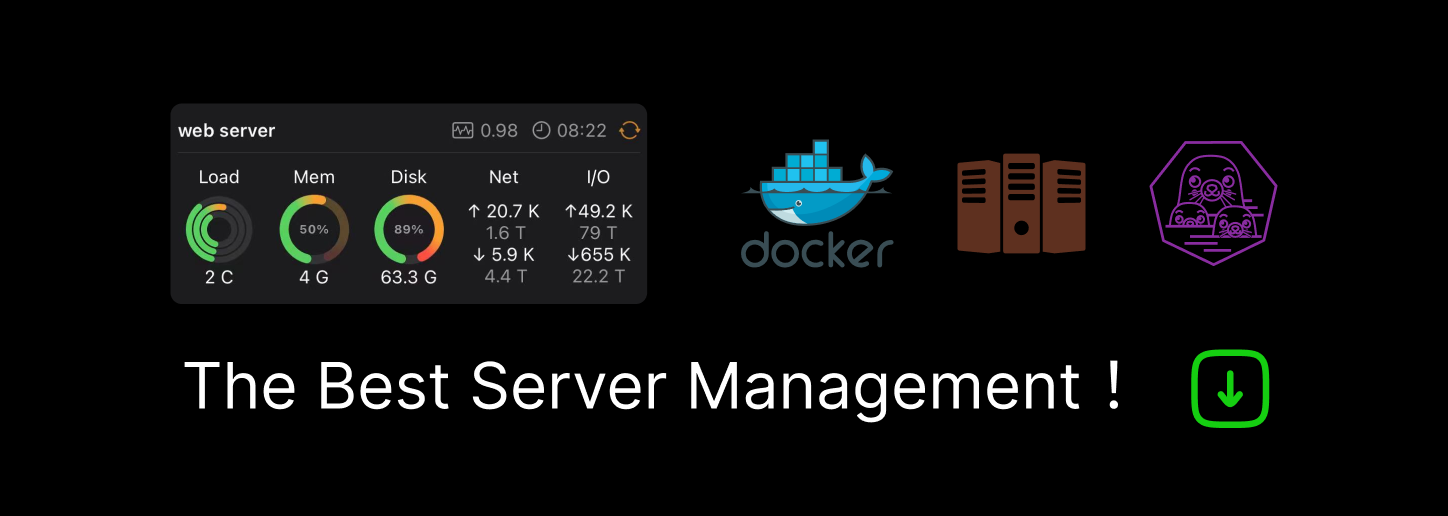
Auxiliary constructor in Scala
Reading Time: 3 minutes
Before starting with auxiliary constructor I request you to first visit my previous blog Primary Constructor in Scala.
Scala has two types of constructor
i ) primary constructor.
ii ) auxiliary constructor (secondary constructor).
A scala class can contain zero or more auxiliary constructor.
The auxiliary constructor in Scala is used for constructor overloading and defined as a method using this name.
The auxiliary constructor must call either previously defined auxiliary constructor or primary constructor in the first line of its body. Hence every auxiliary constructor invokes directly or indirectly to a primary constructor.
we can call the primary constructor or other auxiliary constructor using this.
Here is an example,
xxxxxxxxxx
scala> class Employee(empId: Int,name: String,salary :Double){
| def this()
| {
| this(0,"",0.0)
| println("Zero-argument auxiliary constructor")
| }
| println("Primary construtor")
| }
defined class Employee
scala> val emp = new Employee()
Primary construtor
Zero-argument auxiliary constructor
emp: Employee = Employee@7186b202
See the above code, when we create an object using zero-argument auxiliary constructor then there is the first statement this(0,””,0.0) which will call to a primary constructor. Hence first Primary constructor body will be executed then after auxiliary constructor body.
we can also call directly to the primary constructor.
xxxxxxxxxx
scala> val emp = new Employee(101,"Smith",50000)
Primary construtor
emp: Employee = Employee@6f930e0
When you compile Employee.scala using scalac Employee.scala and after convert it into java code using javap Employee.class.
see the below-generated java code.
Compiled from "Employee.scala" public class Employee { public Employee(int, java.lang.String, double); //java parameterized constructor. public Employee(); // java default constructor. }
Now create Scala class with a primary constructor and multiple auxiliary constructors
xxxxxxxxxx
scala> class Employee(empId: Int,name: String,salary :Double){
| def this(empId:Int,name:String)
| {
| this(0,"",0.0) // here it invokes primary constructor.
| println("Two-argument auxiliary constructor")
| }
| def this(empId:Int)
| {
| this(0,"",0.0) // here it invokes primary constructor.
| println("One-argument auxiliary constructor")
| }
| def this()
| {
| this(0) // here it invokes one-argument auxiliary constructor.
| println("Zero-argument auxiliary constructor")
| }
| println("Primary construtor")
| }
defined class Employee
scala> val emp = new Employee()
Primary construtor
One-argument auxiliary constructor
Zero-argument auxiliary constructor
emp: Employee = Employee@291a4791
see the above output when we create an instance with the help of zero arguments auxiliary constructor. Zero argument constructor call to One argument auxiliary constructor and One argument constructor to a Primary constructor. So the primary constructor body would execute first then after one argument constructor body then after zero arguments.
Now,
xxxxxxxxxx
scala> val emp = new Employee(101,"John")
Primary construtor
Two-argument auxiliary constructor
emp: Employee = Employee@37e69c43
When you invoke more than one time this in the auxiliary constructor then it will invoke apply() in the class,
xxxxxxxxxx
scala> class Point(x: Int,y: Int) {
| def this(x: Int) {
| this(x,5)
| println("auxiliary constructor")
| this(x,5); // it will invokes object's apply method like this.apply(x,5).
| }
| def apply(x:Int,y:Int) = println(s" x= $x and y = $y")
| }
defined class Point
scala> val point = new Point(10)
auxiliary constructor
x= 10 and y = 5
point: Point = Point@310b2b6f
If an apply method does not define in the class and call more than one times this in the auxiliary constructor then it will give compile time error.
xxxxxxxxxx
scala> class Point(x: Int,y: Int) {
| def this(x: Int) {
| this(x,5)
| println("auxiliary constructor")
| this(x,5)
| }
| }
:15: error: Point does not take parameters
this(x,5)
In Scala, We can also create a primary constructor with a default value. If you don’t provide value then it will take default value which is provided in the Primary constructor. Otherwise the value we provide when an instance created with the help of a parameter name of the class.
Here is an example,
xxxxxxxxxx
scala> class Employee(empId: Int = 0,name: String = "",salary :Double = 0.0){
| println(s"empId = $empId , empName = $name , salary = $salary")
| }
defined class Employee
scala> val emp = new Employee()
empId = 0 , empName = , salary = 0.0
emp: Employee = Employee@713999c2
scala> val emp1 = new Employee(name = "Smith")
empId = 0 , empName = Smith , salary = 0.0
emp1: Employee = Employee@6be766d1
scala> val emp1 = new Employee(name = "Smith", empId = 9)
empId = 9 , empName = Smith , salary = 0.0
emp1: Employee = Employee@5ddb302
If we provide a wrong parameter name of the class at the time of instance creation then it will give the compile-time error.
xxxxxxxxxx
scala> val emp1 = new Employee(name = "Smith", empIf = 9)
:13: error: unknown parameter name: empIf
val emp1 = new Employee(name = "Smith", empIf = 9)
Please comment, if you have any doubt or suggestion.
thank you
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK