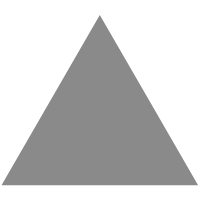
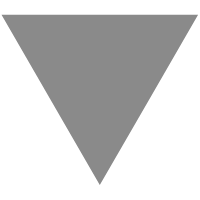
make_shared
source link: https://www.cplusplus.com/reference/memory/make_shared/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
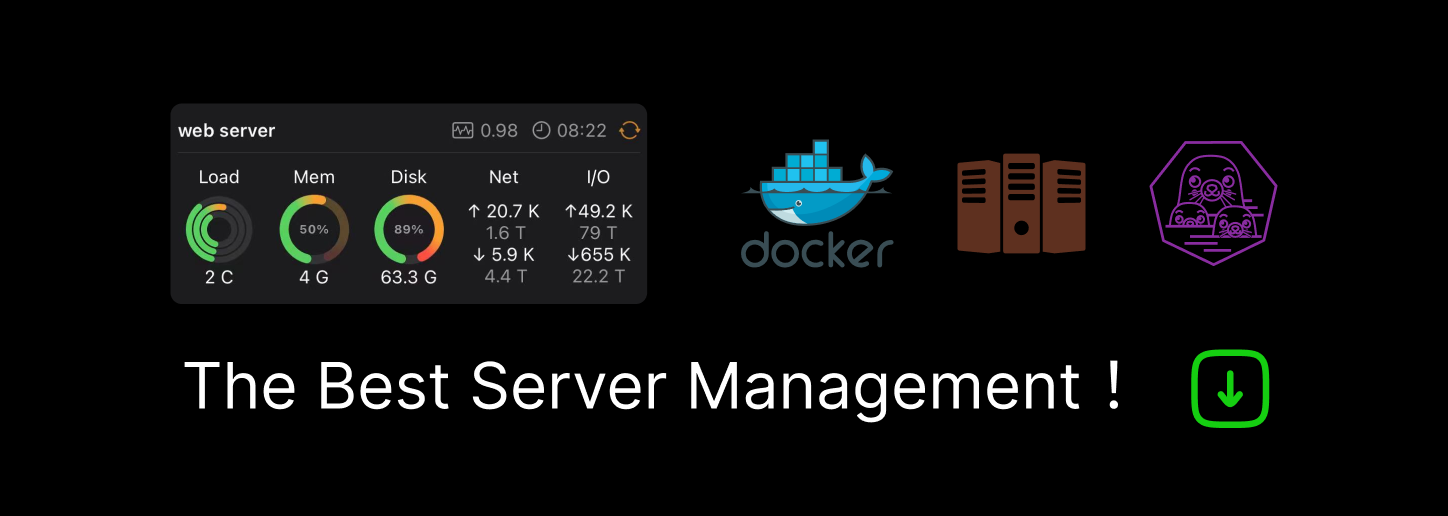
std::make_shared
template <class T, class... Args> shared_ptr<T> make_shared (Args&&... args);
Allocates and constructs an object of type T passing args to its constructor, and returns an object of type shared_ptr<T> that owns and stores a pointer to it (with a use count of 1).
This function uses ::new to allocate storage for the object. A similar function, allocate_shared, accepts an allocator as argument and uses it to allocate the storage.
Parameters
args List of elements passed to T's constructor.Args is a list of zero or more types.
Return Value
A shared_ptr object that owns and stores a pointer to a newly allocated object of type T.Example
// make_shared example
#include <iostream>
#include <memory>
int main () {
std::shared_ptr<int> foo = std::make_shared<int> (10);
// same as:
std::shared_ptr<int> foo2 (new int(10));
auto bar = std::make_shared<int> (20);
auto baz = std::make_shared<std::pair<int,int>> (30,40);
std::cout << "*foo: " << *foo << '\n';
std::cout << "*bar: " << *bar << '\n';
std::cout << "*baz: " << baz->first << ' ' << baz->second << '\n';
return 0;
}
*foo: 10 *bar: 20 *baz: 30 40
See also
allocate_sharedAllocate shared_ptr (function template )shared_ptr::shared_ptrConstruct shared_ptr (public member function )shared_ptr::operator=shared_ptr assignment (public member function )Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK