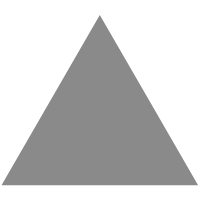
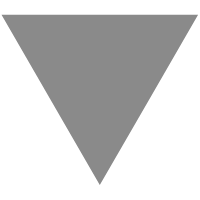
RESTful API Design Best Practices | Developer.com
source link: https://www.developer.com/web-services/best-practices-restful-api/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
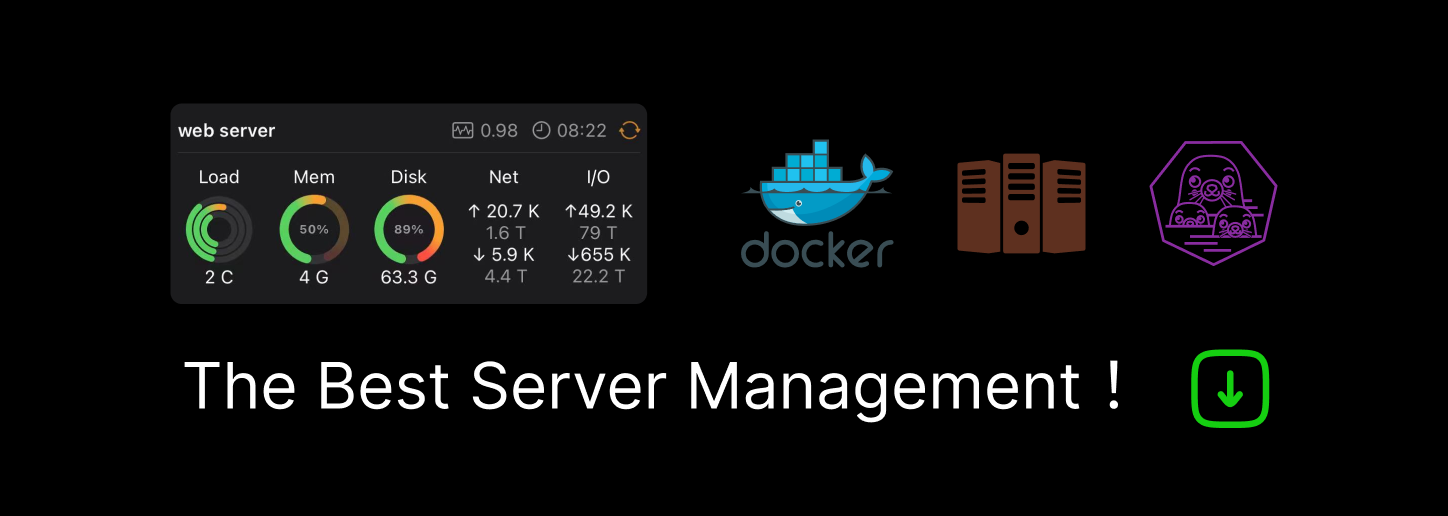
Poorly designed APIs are difficult to maintain over time, and they can fail often. Such APIs are also vulnerable to security threats from hackers looking for sensitive data. This article talks about what REST is all about, showcases some REST resources, and outlines the best practices that should be followed to design RESTful APIs.
What is REST?
REST, an acronym for Representational State Transfer, is a common architectural paradigm for developing scalable services that interact statelessly via the HTTP protocol. REST is neither technology nor a set of standards; it is a collection of constraints built around a cacheable, stateless communication protocol. A RESTful API or Service follows the REST principles and operates on data using HTTP verbs.
The REST architectural style partitions the state and functionality of an application into resources. These resources are accessible through URIs over HTTP, have a similar interface, and are addressable uniquely.
Editor’s Note: Read more REST articles.
The architectural constraints of the REST architectural style include the following:
- Uniform Interface
- Stateless
- Cacheable
- Client-Server
- Layered System
- Code on Demand
Best Practices for using RESTful APIs
Here are the best practices that can be adopted when working with RESTful APIs.
Use JSON for Communication
JSON is an accepted standard these days for communicating with APIs. It is a preferred choice over XML since it reduces the payload significantly, which leads to faster transmission of data. Hence your REST APIs should use JSON to communicate between the client and the server and vice-versa. Note that REST is format-agnostic, which means that you can use any format of your choice for data exchange, such as XML, HTML, JSON, and even custom formats.
To guarantee that your REST API communicates in JSON, you may specify the Content-Type header of the response to application/json. Essentially, your REST APIs should return data in JSON as well as should be capable of accepting JSON payloads.
Versioning
API versioning is the process followed to manage changes to an API. It is a good practice to always version your API. When designing REST-based APIs, you should remember change is inevitable. You might need to make a breaking change in your API to cater to client demands.
Such a breaking change might be needed because of a change in the format of the response data, change in the type of the response, introducing new functionalities, editing or deleting existing functionalities, etc. Hence it is imperative that you have strategies in place to cater to the change.
Versioning is one such strategy that helps you iterate faster. There are different ideas around how API versioning can be implemented. Broadly, here are the strategies you can adopt for implementing versioning of your RESTful APIs.
URI Path
URI path is the simplest strategy to convey version information. In this strategy, you would typically want to put the version number in the URI path. The version number is prefixed using the character “v”.
Here are a few examples that illustrate how API versioning can be specified using the URI path:
- http://www.mywebsite.com/api/v1.0/products
- http://www.mywebsite.com/api/v2.1/orders
Request Parameter
You can specify version information in the request parameter as well. The base URL would remain the same – the version information is passed via the request parameter as shown in the code snippet given below:
- http://www.mywebsite.com/api/products/{id}?version=1.0
This is quite a flexible approach for requesting the version of the resource.
Request Header
This is yet another strategy to specify version information in RESTful APIs. Here’s an example that illustrates how version information can be used in the accept header.
Accept: version=1.0
So, you can specify version information in the request header – you need not make any change in the URL.
Documentation
An API is incomplete without proper documentation. The documentation should be publicly accessible and talk about the API endpoints and request/response cycles. Remember that once your API is publicly accessible, you should not make changes without notifying them.
Handle Errors Gracefully
You should handle errors gracefully and return the relevant HTTP response codes when an error has occurred. The HTTP response codes would provide enough information to understand the cause of the error and the problem that has occurred.
Here’s a list of the common HTTP status codes:
- 400 Bad Request – this is usually returned occurs when a client-side input is invalid
- 401 Unauthorized – this is returned when an unauthorized user tries to access a resource
- 403 Forbidden – this is returned when the user is authenticated but doesn’t have the privilege to access a particular resource
- 404 Not Found – this is returned when a resource that has been requested by the consumer is not found
- 500 Internal server error – this is returned when the server encounters an unforeseen circumstance that prevents it from completing a certain request
- 502 Bad Gateway – this is returned when there is an invalid response from an upstream server
- 503 Service Unavailable – this is returned to indicate that something unexpected has happened on the server-side
You should return error details in the response body – this would help your developers to debug and detect the cause of the problem.
HTTP/1.1 400 Bad Request Content-Type: application/json { "error": "Invalid request.", "detail": { "address": "The address field is required." } }
You should use your status codes consistently. As an example, if you’ve used a 200 OK for a GET endpoint, you should use the same status code for all GET endpoints.
Here’s what you should use to ensure consistency:
- GET: 200 OK
- POST: 201 Created
- PUT: 200 OK
- PATCH: 200 OK
- DELETE: 204 No Content
Avoid Using Verbs in the URIs
You should not describe the action being performed using verbs in the URL. It is a bad practice to use verbs in your RESTful API. The HTTP method itself is a verb: GET, PUT, POST, DELETE, while the URL in a RESTful API should always contain nouns. Moreover, RESTful URI must refer to a resource (a noun) rather than an action (a verb).
There are certain exceptions to this as well. As an example, verbs are used for some specific actions, as shown in the code snippet given below:
/login /logout /register
Use SSL, Always
You should always use SSL. Period. There cannot be any exception to this at all. Your APIs might be accessed from almost anywhere as long as there is internet connectivity. Hence you should ensure that your APIs are secure. The communication between the server and the consumer using your RESTful APIs should be secured, encrypted. That’s exactly why you should use SSL.
Improving Performance
For improved performance, you should use JSON responses instead of XML responses. XML is not a good choice for designing RESTful APIs since it is verbose, difficult to read and parse. The size of the payload will also reduce if you use JSON in place of XML.
You can make PATCH calls to the APIs when the amount of data to be updated is less. For example, you can return updated or created representation as part of the response to eliminate the need to call the API repeatedly for an updated representation.
Another good way to boost the performance of RESTful APIs is by limiting the fields returned by the API. Most of the time, your API wouldn’t have to return the complete representation of a resource. By reducing the number of fields returned by your API, you can minimize network traffic and boost the performance of your APIs.
RESTFul API Best Practices Summary
There isn’t any specific approach to API design – you just need to adhere to the best practices and guidelines. RESTful APIs should be complete, concise, easy to read and work with, and well documented. Remember, building and designing RESTful APIs is crucial for every organization – the consumers of your RESTful APIs should be able to consume and work with them effortlessly.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK