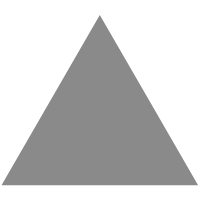
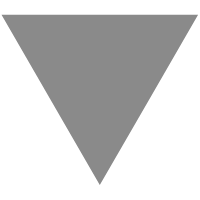
zookeeper代码实现常用命令 - 雨中散步撒哈拉
source link: https://segmentfault.com/a/1190000040076168
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
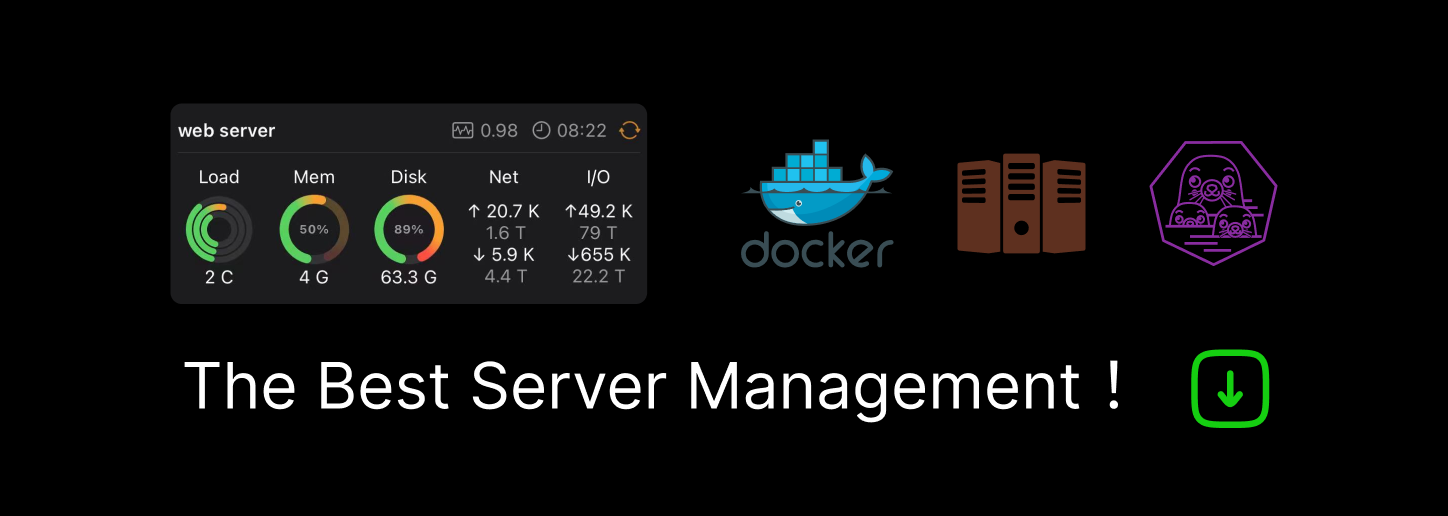
作者:雨中散步撒哈拉
来源:https://liudongdong.top
公众号:雨中散步撒哈拉
备注:欢迎关注公众号,学习技术,一起成长!文末福利:上百本电子书,等待你的领取^v^
环境说明:
- 服务器为centos7集群
- zk为zookeeper-3.4.10.tar.gz版本
- jdk为1.8
一、创建项目
1. 添加依赖包,pom文件如下
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>RELEASE</version>
</dependency>
<dependency>
<groupId>org.apache.logging.log4j</groupId>
<artifactId>log4j-core</artifactId>
<version>2.8.2</version>
</dependency>
<!--
https://mvnrepository.com/artifact/org.apache.zookeeper/zook
eeper -->
<dependency>
<groupId>org.apache.zookeeper</groupId>
<artifactId>zookeeper</artifactId>
<version>3.4.10</version>
</dependency>
</dependencies>
2. 配置日志文件
资源文件创建日志配置文件log4j.properties
log4j.rootLogger=INFO, stdout
log4j.appender.stdout=org.apache.log4j.ConsoleAppender
log4j.appender.stdout.layout=org.apache.log4j.PatternLayout
log4j.appender.stdout.layout.ConversionPattern=%d %p [%c] - %m%n
log4j.appender.logfile=org.apache.log4j.FileAppender
log4j.appender.logfile.File=target/spring.log
log4j.appender.logfile.layout=org.apache.log4j.PatternLayout
log4j.appender.logfile.layout.ConversionPattern=%d %p [%c] - %m%n
二、代码实现zk命令
0. 创建连接
连接集群ip和对外端口2181,集群映射,已在windows做了配置
hosts文件内容:
代码实现连接:
private static final String IPS = "master:2181,slave1:2181,slave2:2181";
private static final int SESSIONTIMEOUT = 200;
private ZooKeeper zkClient = null;
@Test
public void contectTest() throws Exception{
zkClient = new ZooKeeper(IPS, SESSIONTIMEOUT, new Watcher() {
@Override
public void process(WatchedEvent event) {
}
});
System.out.println("==================");
System.out.println(zkClient.getState());
System.out.println("==================");
}
运行打印结果
image.png
2. 创建节点
创建/idea节点,类型为临时节点
private static final String IPS = "master:2181,slave1:2181,slave2:2181";
private static final int SESSIONTIMEOUT = 200;
private ZooKeeper zkClient = null;
@Before
public void contectTest() throws Exception{
zkClient = new ZooKeeper(IPS, SESSIONTIMEOUT, new Watcher() {
@Override
public void process(WatchedEvent event) {
}
});
System.out.println("==================");
System.out.println(zkClient.getState());
System.out.println("==================");
}
@Test
public void createTest() throws Exception{
String s = zkClient.create("/idea", "helloworld".getBytes(), ZooDefs.Ids.OPEN_ACL_UNSAFE, CreateMode.EPHEMERAL);
System.out.println(s);
}
image.png
3. 监听节点变化
监控根节点下的子节点变化
private static final String IPS = "master:2181,slave1:2181,slave2:2181";
private static final int SESSIONTIMEOUT = 200;
private ZooKeeper zkClient = null;
@Before
public void contectTest() throws Exception{
zkClient = new ZooKeeper(IPS, SESSIONTIMEOUT, new Watcher() {
@Override
public void process(WatchedEvent event) {
System.out.println(event.getType() + "--" + event.getPath());
try {
zkClient.getChildren("/", true);
} catch (KeeperException e) {
e.printStackTrace();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
});
System.out.println("==================");
System.out.println(zkClient.getState());
System.out.println("==================");
}
@Test
public void childrenTest() throws Exception{
List<String> children = zkClient.getChildren("/", true);
for (String ch : children){
System.out.println(ch);
}
Thread.sleep(Long.MAX_VALUE);
}
在xshell模范添加节点,也可以在代码中模仿添加节点
[zk: localhost:2181(CONNECTED) 0] create -e /java "java"
Created /java
[zk: localhost:2181(CONNECTED) 1] create -e /python "java"
Created /python
image.png
4. 判断节点是否存在
private static final String IPS = "master:2181,slave1:2181,slave2:2181";
private static final int SESSIONTIMEOUT = 200;
private ZooKeeper zkClient = null;
@Before
public void contectTest() throws Exception{
zkClient = new ZooKeeper(IPS, SESSIONTIMEOUT, new Watcher() {
@Override
public void process(WatchedEvent event) {
}
});
System.out.println("==================");
System.out.println(zkClient.getState());
System.out.println("==================");
}
@Test
public void exTest() throws Exception{
Stat exists = zkClient.exists("/test", false);
System.out.println(exists == null ? "no" : "yes");
}
image.png
5. 测试完整代码
package com.example.demo;
import org.apache.zookeeper.*;
import org.apache.zookeeper.data.Stat;
import org.junit.Before;
import org.junit.Test;
import java.util.List;
public class ZKDemo {
private static final String IPS = "master:2181,slave1:2181,slave2:2181";
private static final int SESSIONTIMEOUT = 200;
private ZooKeeper zkClient = null;
@Before
public void contectTest() throws Exception{
zkClient = new ZooKeeper(IPS, SESSIONTIMEOUT, new Watcher() {
@Override
public void process(WatchedEvent event) {
/*System.out.println(event.getType() + "--" + event.getPath());
try {
zkClient.getChildren("/", true);
} catch (KeeperException e) {
e.printStackTrace();
} catch (InterruptedException e) {
e.printStackTrace();
}*/
}
});
System.out.println("==================");
System.out.println(zkClient.getState());
System.out.println("==================");
}
@Test
public void createTest() throws Exception{
String s = zkClient.create("/idea", "helloworld".getBytes(), ZooDefs.Ids.OPEN_ACL_UNSAFE, CreateMode.EPHEMERAL);
System.out.println(s);
}
@Test
public void childrenTest() throws Exception{
List<String> children = zkClient.getChildren("/", true);
for (String ch : children){
System.out.println(ch);
}
Thread.sleep(Long.MAX_VALUE);
}
@Test
public void exTest() throws Exception{
Stat exists = zkClient.exists("/test", false);
System.out.println(exists == null ? "no" : "yes");
}
}
包含c、c++、java、python、linux、html、php等上百本电子书!
获取方式:
搜索并关注公众号:雨中散步撒哈拉
回复关键词:001
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK