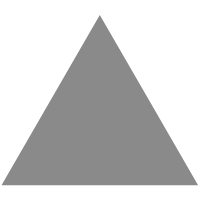
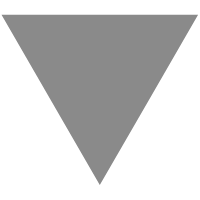
Angular Scheduler: Row Filtering | DayPilot Code
source link: https://code.daypilot.org/52354/angular-scheduler-row-filtering
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
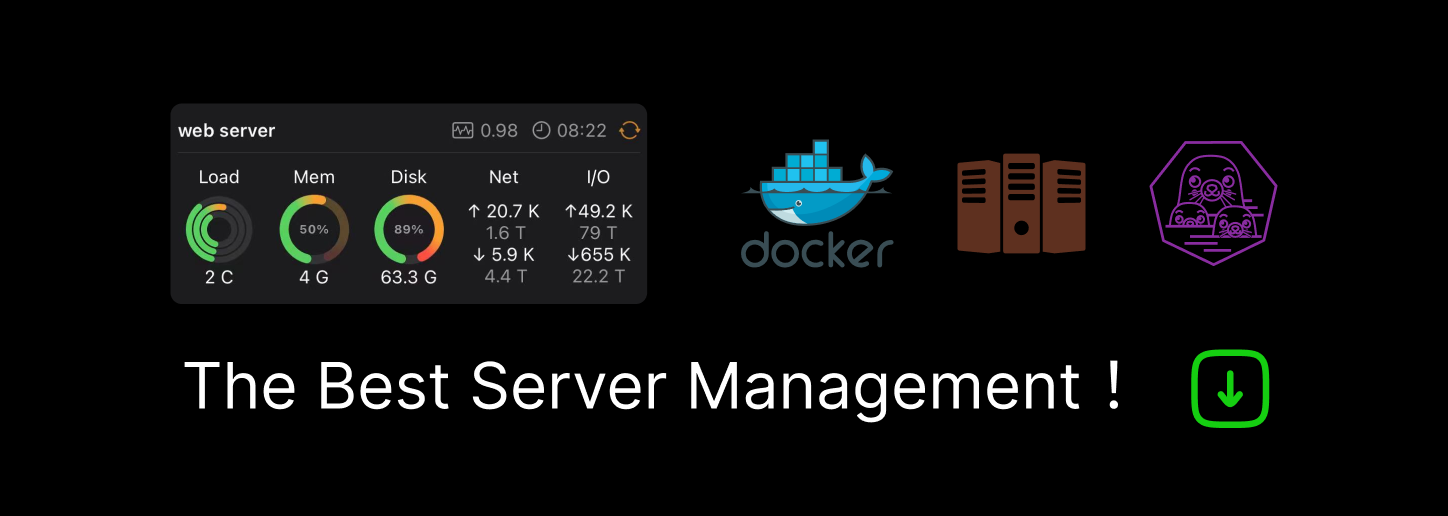
Features
Angular Scheduler component with support for row filtering from DayPilot Pro for JavaScript
Real-time filtering by row name
Allows hiding rows with no events
Includes a trial version of DayPilot Pro for JavaScript
Uses Angular 12
For an introduction to using Angular Scheduler, please see the following tutorial:
See also a similar tutorial on event filtering:
License
Licensed for testing and evaluation purposes. Please see the license agreement included in the sample project. You can use the source code of the tutorial if you are a licensed user of DayPilot Pro for JavaScript. Buy a license.
Live Demo
You can test this project in a live demo:
Filtering Scheduler Rows by Name
Full Scheduler with all rows:
Filtered rows:
The row filter implementation has three parts:
The filter UI (
<input>
element for the filter text)rows.filter() method that is invoked on every filter UI change - it passes the filter parameters to the Scheduler and requests an update
onRowFilter event handler which resolves the filter parameters for every row (to determine whether it should be visible or not)
Filter input UI:
<input type="text" [ngModel]="filter.text" (ngModelChange)="changeText($event)" />
Filter update logic:
export class SchedulerComponent implements AfterViewInit { @ViewChild("scheduler") scheduler!: DayPilotSchedulerComponent; filter = { text: "" }; // ... changeText(text: string): void { this.filter.text = text; this.applyFilter(); } applyFilter(): void { this.scheduler.control.rows.filter(this.filter); } }
Row filter event handler:
config: any = { // ... onRowFilter: args => { if (args.row.name.toLowerCase().indexOf(args.filter.text.toLowerCase()) < 0) { args.visible = false; } } };
By default all rows are visible (args.visible
is set to false
). The onRowFilter
event handler checks the filter condition sent from the rows.filter()
method (it's available as args.filter
in the event handler). If the row doesn't meet the filter condition it's marked as not visible (args.visible = false
).
Note that the parent row will always be displayed (even if marked with args.visible = false
).
Full row name filter implementation (scheduler.component.ts
):
import {Component, ViewChild, AfterViewInit, ChangeDetectorRef} from "@angular/core"; import {DayPilot, DayPilotSchedulerComponent} from "daypilot-pro-angular"; import {DataService} from "./data.service"; @Component({ selector: 'scheduler-component', template: ` <div class="space"> Filter: <input type="text" [ngModel]="filter.text" (ngModelChange)="changeText($event)" /> ... </div> <daypilot-scheduler [config]="config" [events]="events" #scheduler></daypilot-scheduler> `, styles: [``] }) export class SchedulerComponent implements AfterViewInit { @ViewChild("scheduler") scheduler!: DayPilotSchedulerComponent; events: any[] = []; filter = { text: "" }; config: DayPilot.SchedulerConfig = { // ... onRowFilter: args => { if (args.row.name.toLowerCase().indexOf(args.filter.text.toLowerCase()) < 0) { args.visible = false; } } }; changeText(text: string): void { this.filter.text = text; this.applyFilter(); } applyFilter(): void { this.scheduler.control.rows.filter(this.filter); } }
Filtering by Events
The same approach is used for filtering the rows depending on their content. The onRowFilter
event handler checks if there are any events in the row using args.row.events.all()
:
onRowFilter: args => { if (args.filter.eventsOnly && args.row.events.all().length === 0) { args.visible = false; } }
scheduler.component.ts
import {Component, ViewChild, AfterViewInit, ChangeDetectorRef} from "@angular/core"; import {DayPilot, DayPilotSchedulerComponent} from "daypilot-pro-angular"; import {DataService} from "./data.service"; @Component({ selector: 'scheduler-component', template: ` <div class="space"> ... <label for="eventsonly"><input type="checkbox" id="eventsonly" [ngModel]="filter.eventsOnly" (ngModelChange)="changeWithEvents($event)"> Only rows with events</label> ... </div> <daypilot-scheduler [config]="config" [events]="events" #scheduler></daypilot-scheduler> `, styles: [``] }) export class SchedulerComponent implements AfterViewInit { @ViewChild("scheduler") scheduler!: DayPilotSchedulerComponent; events: any[] = []; filter = { eventsOnly: false }; config: DayPilot.SchedulerConfig = { // ... onRowFilter: args => { if (args.filter.eventsOnly && args.row.events.all().length === 0) { args.visible = false; } } }; changeWithEvents(val: string): void { this.filter.eventsOnly = val; this.applyFilter(); } applyFilter(): void { this.scheduler.control.rows.filter(this.filter); } }
Clearing the Filter
In order to clear the filter, we simply reset the filter object and apply the filter:
export class SchedulerComponent implements AfterViewInit { filter = { text: "", eventsOnly: false }; // ... applyFilter(): void { this.scheduler.control.rows.filter(this.filter); } clearFilter(): boolean { this.filter.text = ""; this.filter.eventsOnly = false; this.applyFilter(); return false; } }
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK