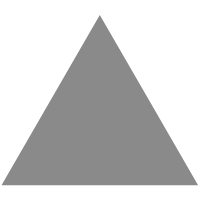
5
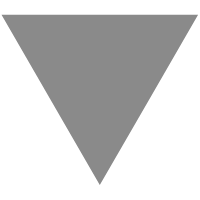
【前端工具】前端工具函数合集
source link: https://blog.vadxq.com/article/fe-tools-functions/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
【前端工具】前端工具函数合集
收集一些前端工具函数,整理成集,以便查询使用。随时更新:2021-03-03
正则匹配相关
手机号正则验证
- 匹配所有手机卡
^(?:\+?86)?1(?:3\d{3}|5[^4\D]\d{2}|8\d{3}|7(?:[235-8]\d{2}|4(?:0\d|1[0-2]|9\d))|9[0-35-9]\d{2}|66\d{2})\d{6}$
- 更多可以查看一组匹配中国大陆手机号码的正则表达式
将手机号中间四位隐藏为****
const phone = 17600000000;
const data = phone.toString().replace(/(\d{3})\d{4}(\d{4})/, "$1****$2");
console.log(data);
// 176****0000
cookie 操作相关
/**
* 设置cookie
* @param {String} name cookie name
* @param {String} val cookie value
*/
export const setCookie = (name, val) => {
const date = new Date();
const value = val;
// Set it expire in 7 days
date.setTime(date.getTime() + 7 * 24 * 60 * 60 * 1000);
// Set it
document.cookie =
name + "=" + value + "; expires=" + date.toUTCString() + "; path=/";
};
/**
* 获取cookie
* @param {String} name cookie name
*/
export const getCookie = (name) => {
const value = "; " + document.cookie;
const parts = value.split("; " + name + "=");
if (parts.length === 2) {
const ppop = parts.pop();
if (ppop) {
return ppop.split(";").shift();
}
}
};
/**
* 删除cookie
* @param {String} name cookie name
*/
export const deleteCookie = (name) => {
const date = new Date();
// Set it expire in -1 days
date.setTime(date.getTime() + -1 * 24 * 60 * 60 * 1000);
// Set it
document.cookie = name + "=; expires=" + date.toUTCString() + "; path=/";
};
格式化时间
将时间戳变为个位数带 0 前缀
/**
* 格式化时间,间隔符为-
* @param {Number} date 大小,单位时间戳13位
* @return {String} 格式化后的大小 2021-03-12 20:21:02
*/
export const formatDate = (date) => {
const da = new Date(date);
const year = da.getFullYear();
const month = da.getMonth() + 1;
const day = da.getDate();
const hours = da.getHours();
const mins = da.getMinutes();
const secs = da.getSeconds();
return `${year}-${month > 9 ? month : "0" + month}-${
day > 9 ? day : "0" + day
} ${hours > 9 ? hours : "0" + hours}:${mins > 9 ? mins : "0" + mins}:${
secs > 9 ? secs : "0" + secs
}`;
};
生成 UUID
/**
* 生成uuid
* @param {Number} len 生成的uuid长度
* @param {Number} radix 随机字符的长度选择
* @return {String} uuid
*/
export const UUID = (len, radix) => {
const chars = "0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz".split(
""
);
const uuid = [];
let i;
radix = radix || chars.length;
if (len) {
// Compact form
for (i = 0; i < len; i++) uuid[i] = chars[0 | (Math.random() * radix)];
} else {
// rfc4122, version 4 form
let r;
// rfc4122 requires these characters
uuid[8] = uuid[13] = uuid[18] = uuid[23] = "-";
uuid[14] = "4";
// Fill in random data. At i==19 set the high bits of clock sequence as
// per rfc4122, sec. 4.1.5
for (i = 0; i < 36; i++) {
if (!uuid[i]) {
r = 0 | (Math.random() * 16);
uuid[i] = chars[i === 19 ? (r & 0x3) | 0x8 : r];
}
}
}
return uuid.join("");
};
type 类型判断
export const isString = (e) => { //是否字符串
return Object.prototype.toString.call(e).slice(8, -1) === 'String'
}
export const isNumber = (e) => { //是否数字
return Object.prototype.toString.call(e).slice(8, -1) === 'Number'
}
export const isBoolean = (e) => { //是否boolean
return Object.prototype.toString.call(e).slice(8, -1) === 'Boolean'
}
export const isFunction = (e) => { //是否函数
return Object.prototype.toString.call(e).slice(8, -1) === 'Function'
}
export const isNull = (e) => { //是否为null
return Object.prototype.toString.call(e).slice(8, -1) === 'Null'
}
export const isUndefined = (e) => { //是否undefined
return Object.prototype.toString.call(e).slice(8, -1) === 'Undefined'
}
export const isObj = (e) => { //是否对象
return Object.prototype.toString.call(e).slice(8, -1) === 'Object'
}
export const isArray = (e) => { //是否数组
return Object.prototype.toString.call(e).slice(8, -1) === 'Array'
}
export const isDate = (e) => { //是否时间
return Object.prototype.toString.call(e).slice(8, -1) === 'Date'
}
export const isRegExp = (e) => { //是否正则
return Object.prototype.toString.call(e).slice(8, -1) === 'RegExp'
}
export const isError = (e) => { //是否错误对象
return Object.prototype.toString.call(e).slice(8, -1) === 'Error'
}
export const isSymbol = (e) => { //是否Symbol函数
return Object.prototype.toString.call(e).slice(8, -1) === 'Symbol'
}
export const isPromise = (e) => { //是否Promise对象
return Object.prototype.toString.call(e).slice(8, -1) === 'Promise'
}
export const isSet = (e) => { //是否Set对象
return Object.prototype.toString.call(e).slice(8, -1) === 'Set'
}
random随机数
返回一定范围的整数随机数
// min, 最小的值,max最大的数,包含两者
export const randoms = (min, max) => Math.floor(min + Math.random() * ((max + 1) - min))
/**
* 去除空格
* @param {String} srt: 传入字符串
* @param {Number} type: 1-所有空格 2-前后空格 3-前空格 4-后空格
* @return {String}
*/
export const trim = (str, type = 1) => {
switch (type) {
case 1:
return str.replace(/\s+/g, '')
case 2:
return str.replace(/(^\s*)|(\s*$)/g, '')
case 3:
return str.replace(/(^\s*)/g, '')
case 4:
return str.replace(/(\s*$)/g, '')
default:
return str
}
}
大小写处理
/**
* @param {String} str:
* @param {type} type: 1:首字母大写 2:首页母小写 3:大小写转换 4:全部大写 5:全部小写
* @return {String}
*/
export const changeCase = (str, type = 4) => {
switch (type) {
case 1:
return str.replace(/\b\w+\b/g, (word) => return word.substring(0, 1).toUpperCase() + word.substring(1).toLowerCase())
case 2:
return str.replace(/\b\w+\b/g, (word) => word.substring(0, 1).toLowerCase() + word.substring(1).toUpperCase())
case 3:
return str.split('').map((word) => {
if (/[a-z]/.test(word)) {
return word.toUpperCase()
} else {
return word.toLowerCase()
}
}).join('')
case 4:
return str.toUpperCase()
case 5:
return str.toLowerCase()
default:
return str
}
}
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK