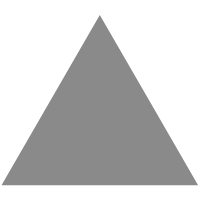
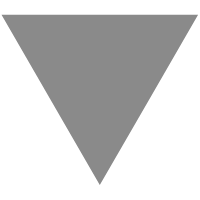
Github proposal: slices: new package to provide generic slice functions · Issue...
source link: https://github.com/golang/go/issues/45955
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
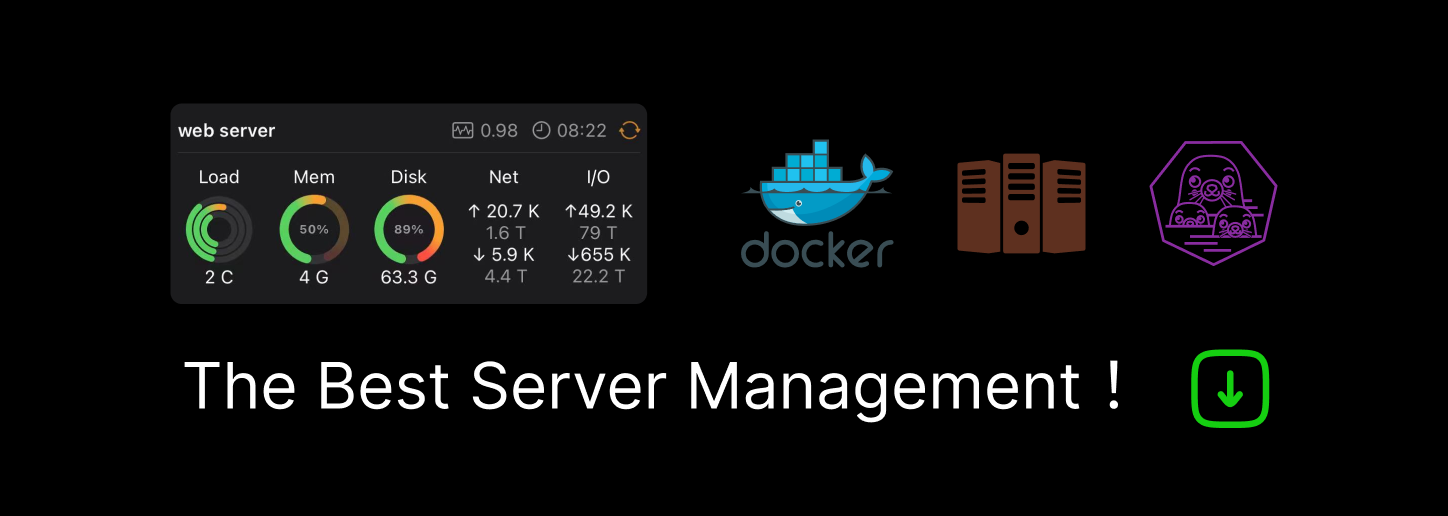
This proposal is for use with #43651. We propose defining a new package, slices
, that will provide functions that may be used with slices of any type. If this proposal is accepted, the new package will be included with the first release of Go that implements #43651 (we currently expect that that will be Go 1.18).
This description below is focused on the API, not the implementation. In general the implementation will be straightforward.
// Package slices defines various functions useful with slices of any type. // Unless otherwise specified, these functions all apply to the elements // of a slice at index 0 <= i < len(s). package slices import "constraint" // See #45458 // Equal reports whether two slices are equal: the same length and all // elements equal. Floating point NaNs are not considered equal. // The elements are compared in index order, and the comparison // stops at the first unequal pair. func Equal[T comparable](s1, s2 []T) bool // EqualFunc reports whether two slices are equal using a comparison // function on each pair of elements. The elements are compared in // index order, and the comparison stops at the first index for which // eq returns false. func EqualFunc[T any](s1, s2 []T, eq func(T, T) bool) bool // Compare does a lexicographic comparison of s1 and s2. // The elements are compared sequentially starting at index 0, // until one element is not equal to the other. The result of comparing // the first non-matching elements is the result of the comparison. // If both slices are equal until one of them ends, the shorter slice is // lexicographically less than the longer one // The result will be 0 if s1==s2, -1 if s1 < s2, and +1 if s1 > s2. func Compare[T constraint.Ordered](s1, s2 []T) int // CompareFunc is like Compare, but uses a comparison function // on each pair of elements. The elements are compared in index order, // and the comparisons stop after the first time cmp returns non-zero. // The result will be the first non-zero result of cmp; if cmp always // returns 0 the result is 0 if len(s1) == len(s2), -1 if len(s1) < len(s2), // and +1 if len(s1) > len(s2). func CompareFunc[T any](s1, s2 []T, cmp func(T, T) int) int // Index returns the index of the first occurrence of v in s, or -1 if not present. func Index[T comparable](s []T, v T) int // IndexFunc is like Index but uses a comparison function. func IndexFunc[T any](s []T, v T, cmp func(T, T) bool) int // Contains reports whether v is present in s. func Contains[T comparable](s []T, v T) bool // ContainsFunc is like Contains, but it uses a comparison function. func ContainsFunc[T any](s []T, v T, cmp func(T, T) bool) bool // LastIndex? LastIndexFunc? // Map turns a []T1 to a []T2 using a mapping function. func Map[T1, T2 any](s []T1, f func(T1) T2) []T2 // Filter returns a new slice containing all elements e in s for which keep(e) is true. func Filter[T any](s []T, keep func(T) bool) []T // Reduce reduces a []T1 to a single value of type T2 using // a reduction function. This applies f cumulatively to the elements of s. // For example, if s a slice of int, the sum of the elements of s is // Reduce(s, 0, func(a, b int) int { return a + b }). func Reduce[T1, T2 any](s []T1, initializer T2, f func(T2, T1) T2) T2 // MapInPlace copies the elements in src to dst, using a mapping function // to convert their values. This panics if len(dst) < len(src). // (Or we could return min(len(dst), len(src)) if that seems better.) func MapInPlace[type D, S](dst []D, src []S, f func(S) D) // FilterInPlace modifies s to contain only elements for which keep(e) is true. // It returns the modified slice. func FilterInPlace[T any](s []T, keep func(T) bool) []T // Insert inserts v into s at index i, returning the modified slice. // In the returned slice r, r[i] == v. This panics if !(i >= 0 && i <= len(s)). // This function is O(len(s)). func Insert[T any](s []T, i int, v T) []T // InsertSlice inserts si into s at index i, returning the modified slice. // In the returned slice r, r[i] == si[0] (unless si is empty). // This panics if !(i >= 0 && i <= len(s)). func InsertSlice[T any](s, si []T, i int) []T // Remove removes the element at index i from s, returning the modified slice. // This panics if !(i >= 0 && i < len(s)). This function is O(len(s)). // This modifies the contents of the slice s; it does not create a new slice. func Remove[T any](s []T, i int) []T // RemoveSlice removes j-i elements from s starting at index i, returning the modified slice. // This can be thought of as a reverse slice expression: it removes a subslice. // This panics if i < 0 || j < i || j > len(s). // This modifies the contents of the slice s; it does not create a new slice. func RemoveSlice[T any](s []T, i, j int) []T // Resize returns s with length c. If the length increases, the new trailing // elements will be set to the zero value of T. The capacity of the returned // slice is not specified. func Resize[T any](s []T, c int) []T
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK