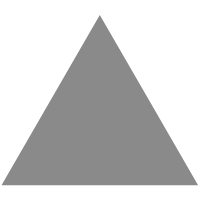
7
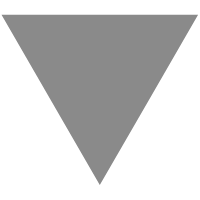
Looped carousel for React Native
source link: https://reactnativeexample.com/looped-carousel-for-react-native/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
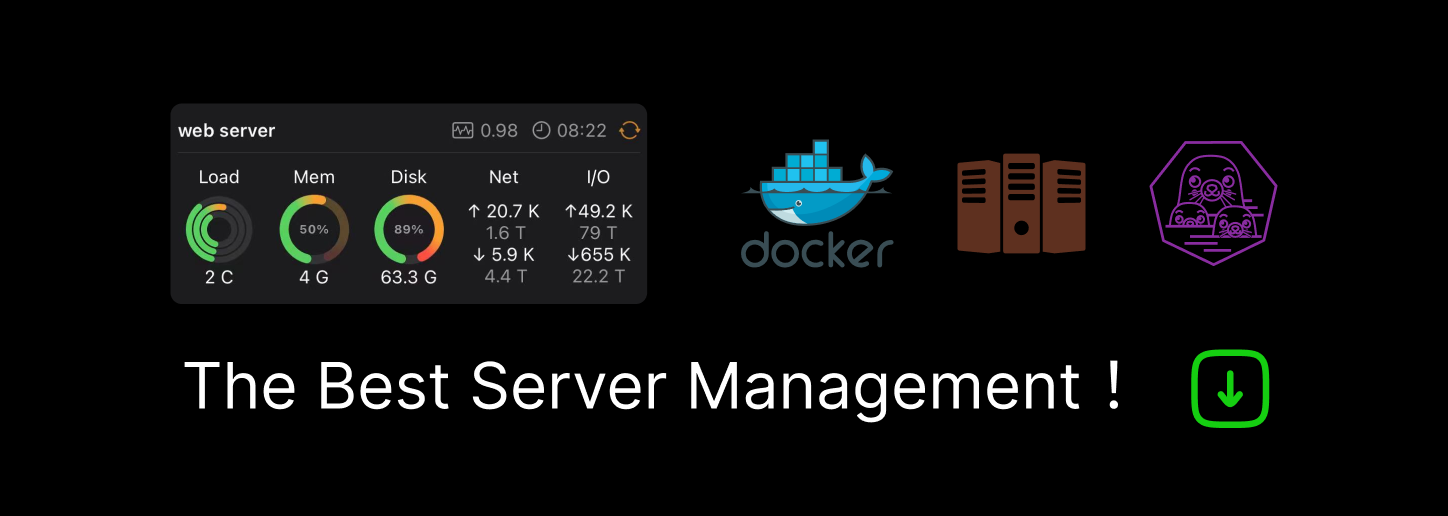
Looped carousel for React Native
Full-fledged "infinite" carousel for your next react-native project. Supports iOS and Android.
Based on react-native framework by Facebook.
Install
npm install react-native-looped-carousel --save
Examples
Props
Name propType default value description autoplay boolean true enables auto animations delay number 4000 number in milliseconds between auto animations currentPage number 0 allows you to set initial page pageStyle style null style for pages contentContainerStyle style nullcontentContainerStyle
for the scrollView
onAnimateNextPage
func
null
callback that is called with 0-based Id of the current page
onPageBeingChanged
func
null
callback that is called when scroll start with 0-based Id of the next page
swipe
bool
true
motion control for Swipe
isLooped
bool
true
if it's possible to scroll infinitely
Pagination
---
---
---
pageInfo
boolean
false
shows {currentPage} / {totalNumberOfPages}
pill at the bottom
pageInfoBackgroundColor
string
'rgba(0, 0, 0, 0.25)'
background color for pageInfo
pageInfoBottomContainerStyle
style
null
style for the pageInfo container
pageInfoTextStyle
style
null
style for text in pageInfo
pageInfoTextSeparator
string
' / '
separator for {currentPage}
and {totalNumberOfPages}
Bullets
---
---
---
bullets
bool
false
wether to show "bullets" at the bottom of the carousel
bulletStyle
style
null
style for each bullet
bulletsContainerStyle
style
null
style for the bullets container
chosenBulletStyle
style
null
style for the selected bullet
Arrows
---
---
---
arrows
bool
false
wether to show navigation arrows for the carousel
arrowStyle
style
null
style for navigation arrows
leftArrowStyle
style
null
style for left navigation arrow
rightArrowStyle
style
null
style for right navigation arrow
arrowsContainerStyle
style
null
style for the navigation arrows container
leftArrowText
string
'Left'
label for left navigation arrow
rightArrowText
string
'Right'
label for right navigation arrow
Change the page
Three options :
- Go to a specific page
- Go to the next page
- Go to the previous page
// assuming ref is set up on the carousel as (ref) => this._carousel = ref
onPress={() => {this._carousel.animateToPage(page)}}
onPress={() => {this._carousel._animateNextPage()}}
onPress={() => {this._carousel._animatePreviousPage()}}
Usage
import React, { Component } from 'react';
import {
Text,
View,
Dimensions,
} from 'react-native';
import Carousel from 'react-native-looped-carousel';
const { width, height } = Dimensions.get('window');
export default class CarouselExample extends Component {
constructor(props) {
super(props);
this.state = {
size: { width, height },
};
}
_onLayoutDidChange = (e) => {
const layout = e.nativeEvent.layout;
this.setState({ size: { width: layout.width, height: layout.height } });
}
render() {
return (
<View style={{ flex: 1 }} onLayout={this._onLayoutDidChange}>
<Carousel
delay={2000}
style={this.state.size}
autoplay
pageInfo
onAnimateNextPage={(p) => console.log(p)}
>
<View style={[{ backgroundColor: '#BADA55' }, this.state.size]}><Text>1</Text></View>
<View style={[{ backgroundColor: 'red' }, this.state.size]}><Text>2</Text></View>
<View style={[{ backgroundColor: 'blue' }, this.state.size]}><Text>3</Text></View>
</Carousel>
</View>
);
}
}
Used in
GitHub
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK