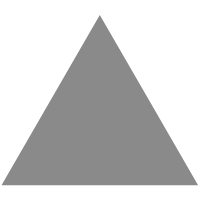
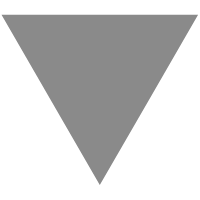
Basic Introduction to Actix-Web Part-1
source link: https://blog.knoldus.com/basic-introduction-to-actix-web-part-1/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
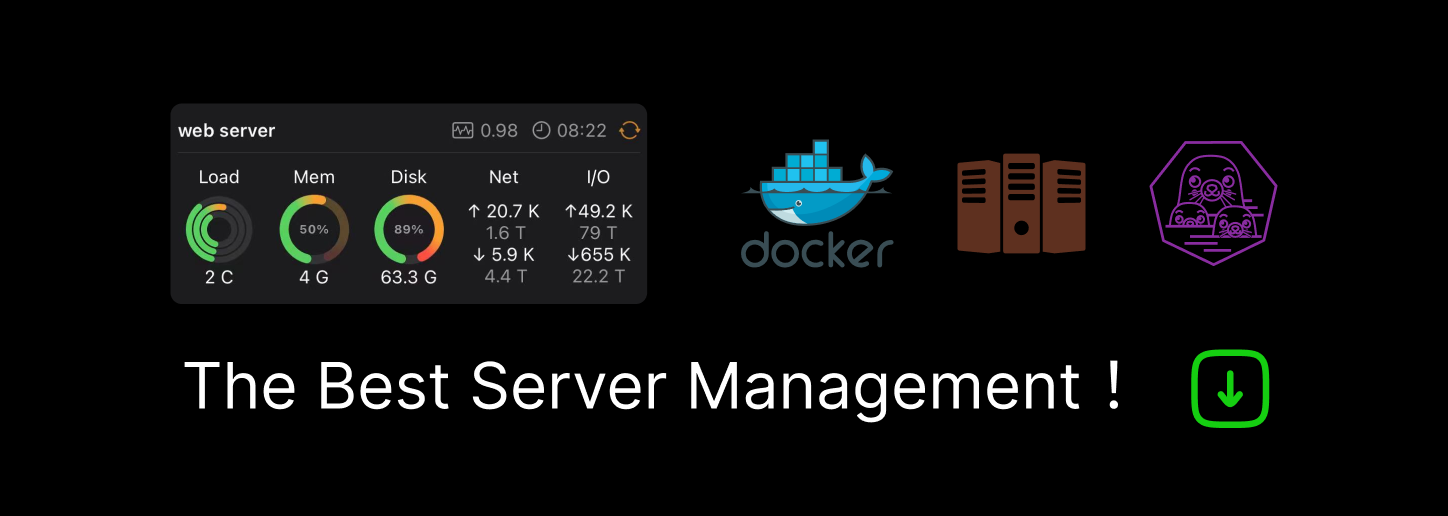
Actix-web is a small and pragmatic framework of RUST. If you are already a Rust programmer you will probably find yourself at home quickly, but even if you are coming from another programming language you should find actix-web easy to pick up.
First of all, you should add an actix-web dependency in cargo.toml
[dependencies]
actix-web = "0.7"
Creating a basic application with Actix:-
A basic example of an actix-web framework :
Filename: src/main.rs
extern crate actix_web;
use actix_web::{server, App, HttpRequest};
fn index(_req: &HttpRequest) -> &'static str {
"Hello world!"
}
fn main() {
server::new(|| App::new().resource("/", |r| r.f(index)))
.bind("127.0.0.1:8088")
.unwrap()
.run();
}
In the above code, we first import actix-web by extern crate actix-web. Next, we define a handler with name index which prints “Hello world!” on the webpage. In the main function, we first define a server instance and in it, we create an application instance and then provide resource and handler and after that, we bind server instance with localhost before calling run.
Combining applications with different states in Actix:-
Combining multiple application with a different state is possible as well. we can see this through example as below:-
Filename: src/main.rs
extern crate actix_web; use actix_web::{http, App, HttpRequest, Responder}; use std::cell::Cell; use actix_web::server; struct State1 { counter: Cell<usize>, } struct State2 { counter: Cell<usize>, } fn index(_req:&HttpRequest<State1>) -> impl Responder { let count = _req.state().counter.get() + 1; _req.state().counter.set(count); format!("Request number in state1: {}", count) } fn index2(_req:&HttpRequest<State2>) -> impl Responder { let count = _req.state().counter.get() + 1; _req.state().counter.set(count); format!("Request number in state2: {}", count) } fn main() { server::new(|| { vec! [ App::with_state(State1{ counter: Cell::new(0) }) .prefix("/app1") .resource("/", |r| r.method(http::Method::GET).f(index)) .boxed(), App::with_state(State2{ counter: Cell::new(0)}) .prefix("/app2") .resource("/",|r| r.method(http::Method::GET).f(index2)) .boxed() ] }).bind("127.0.0.1:8080") .unwrap() .run() }
In the above example, we have defined two application instances on a single server instance. Here, we have defined two structs state1 and state2 which contains a variable count for maintaining the number of hits on app1 and app2. We bind the server instance with localhost i.e. “127.0.0.1:8080” to run it.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK