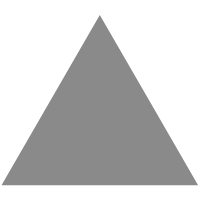
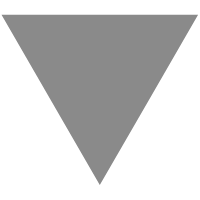
Let us have a tour on Scala
source link: https://blog.knoldus.com/let-us-have-a-tour-on-scala/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
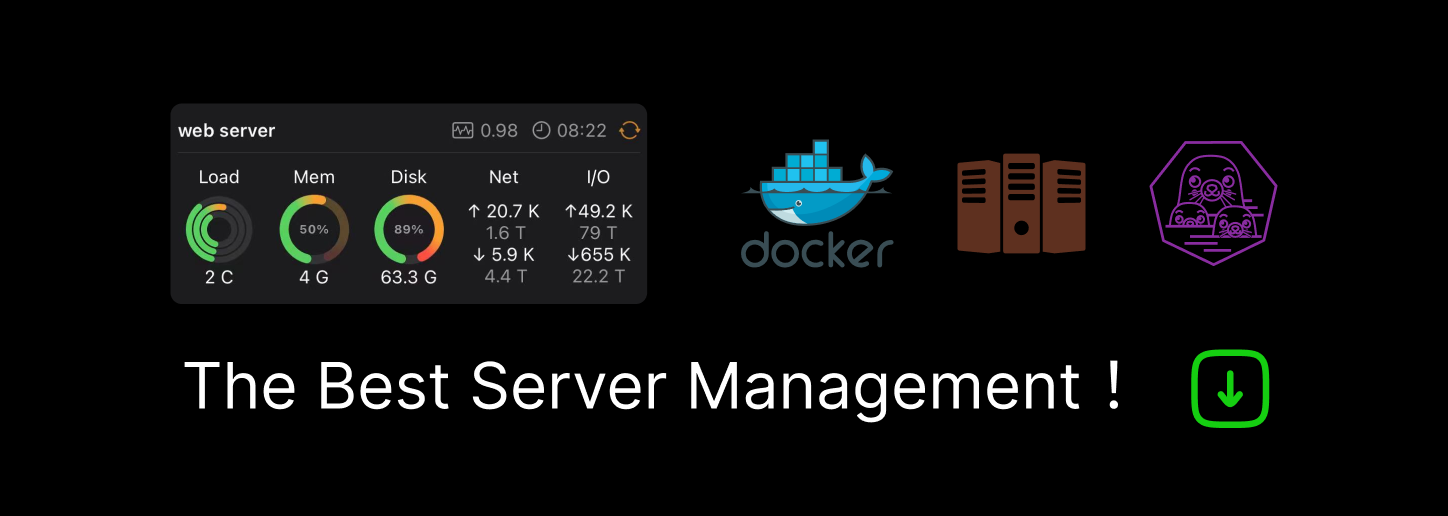
Let us have a tour on Scala
Hello Readers,
If you want to explore what is Scala, why we use Scala and would like to explore some basic concepts of Scala, then this blog is for you.
If you’re a Java programmer, for example, reading this blog will surely expose you to many concepts of Scala.
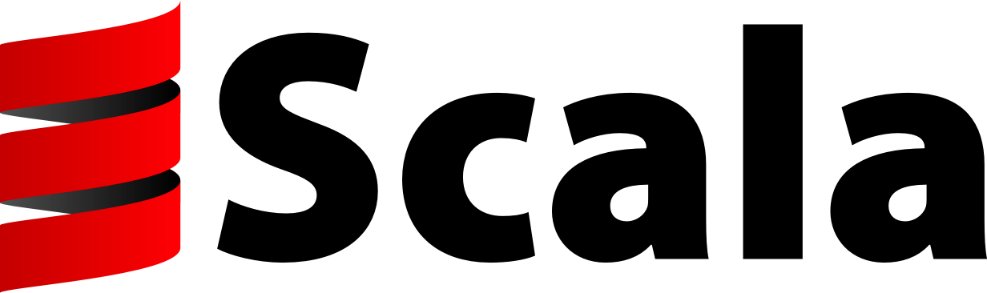
The name Scala stands for “scalable language”. The language is so named because it was designed to grow with the demands of its users. You can apply Scala to a wide range of programming tasks, from writing small scripts to building large systems.
Scala is a modern multi-paradigm programming language designed to express common programming patterns in a concise, elegant, and type-safe way.
So Scala is a scalable programming language for component software with the focus on patterns like abstraction, composition and decomposition and not on primitives.
I’m sure there must be a lot of questions like why should we choose something like Scala?
I have found that there are actually many reasons besides scalability to like programming in Scala. Four of the most important aspects are compatibility, brevity, high-level abstractions, and advanced static typing. Let’s discuss them one by one :
- Scala is compatible
Scala programs compile to JVM bytecodes. Their run-time performance is usually on par with Java programs. Scala code can call Java methods, access Java fields, inherit from Java classes, and implement Java interfaces. None of this requires special syntax, explicit interface descriptions, or glue code. In fact, almost all Scala code makes heavy use of Java libraries, often without programmers being aware of this fact.
- Scala is concise
Scala programs tend to be short. Scala programmers have reported reductions in number of lines of up to a factor of ten compared to Java. Fewer lines of code mean not only less typing, but also less effort at reading and understanding programs and fewer possibilities of defects. There are several factors that contribute to this reduction in lines of code.
As an example, compare how you write classes and constructors in Java and Scala. In Java, a class with a constructor often looks like this:
class MyClass {
private int index;
private String name;
public MyClass(int index, String name) {
this.index = index;
this.name = name;
}
}
In Scala, you would likely write this instead:
class MyClass(index: Int, name: String)
Given this code, the Scala compiler will produce a class that has two private instance variables,an Int named index and a String named name, and a constructor that takes initial values for those
variables as parameters. The code of this constructor will initialize the two instance variables with the values passed as parameters. In short, you get essentially the same functionality as the more verbose Java version.
- Scala is high-level
Scala helps you manage complexity by letting you raise the level of abstraction in the interfaces you design and use. As an example, imagine you have a String variable name, and you want to find out whether or not that String contains an upper case character. Prior to Java 8, you might have written a loop, like this:
boolean nameHasUpperCase = false; // this is Java
for (int i = 0; i < name.length(); ++i) {
if (Character.isUpperCase(name.charAt(i))) {
nameHasUpperCase = true;
break;
}
}
Whereas in Scala, you could write this:
val nameHasUpperCase = name.exists(_.isUpper)
The Java code treats strings as low-level entities that are stepped through character by character in a loop. The Scala code treats the same strings as higher-level sequences of characters that can be queried with predicates. Clearly, the Scala code is much shorter and—for trained eyes—easier to understand than the Java code. So the Scala code weighs less heavily on the total complexity budget. It also gives you less opportunity to make mistakes.
Now that we are somewhat aware of Scala, let’s discuss some of its features :
Features of Scala
- Scala is object-oriented
Scala is a pure object-oriented language in the sense that every value is an object. Types and behavior of objects are described by classes and traits. Classes are extended by subclassing and a flexible mixin-based composition mechanism as a clean replacement for multiple inheritance.
- Scala is Functional
Scala is also a functional language in the sense that every function is a value. Scala provides a lightweight syntax for defining anonymous functions, it supports higher-order functions, it allows functions to be nested, and supports currying. Scala’s case classes and its built-in support for pattern matching model algebraic types used in many functional programming languages. Singleton objects provide a convenient way to group functions that aren’t members of a class.
- Scala is statically typed
Scala is equipped with an expressive type system that enforces statically that abstractions are used in a safe and coherent manner. In particular, the type system supports:
- generic classes
- variance annotations
- upper and lower type bounds,
- inner classes and abstract type members as object members
- compound types
- explicitly typed self-references
- implicit parameters and conversions
- polymorphic methods
- Type Inference
Type inference means the user is not required to annotate code with redundant type information. In combination, these features provide a powerful basis for the safe reuse of programming abstractions and for the type-safe extension of software.
- Scala is extensible
In practice, the development of domain-specific applications often requires domain-specific language extensions. Scala provides a unique combination of language mechanisms that make it easy to smoothly add new language constructs in the form of libraries.
Installing Scala
- Download Scala by typing following command in terminal:
wget https://downloads.lightbend.com/scala/2.12.8/scala-2.12.8.deb
- To install above file, type the following command:
sudo dpkg -i scala-2.12.8.deb
Some Basic Concepts of Scala
Expressions
Expressions are computable statements.
1 + 1
You can output results of expressions using println
.
println(1) // 1
println(1 + 1) // 2
println("Hello!") // Hello!
println("Hello," + " world!") // Hello, world!
Values
You can name results of expressions with the val
keyword.
val x = 1 + 1
println(x) // 2
Named results, such as x
here, are called values. Referencing a value does not re-compute it.
Values cannot be re-assigned.
x = 3 // This does not compile.
Types of values can be inferred, but you can also explicitly state the type, like this:
val x: Int = 1 + 1
Notice how the type declaration Int
comes after the identifier x
. You also need a :
.
Variables
Variables are like values, except you can re-assign them. You can define a variable with the var
keyword.
var x = 1 + 1
x = 3 // This compiles because "x" is declared with the "var" keyword.
println(x * x) // 9
As with values, you can explicitly state the type if you want:
var x: Int = 1 + 1
Blocks
You can combine expressions by surrounding them with {}
. We call this a block.
The result of the last expression in the block is the result of the overall block, too.
println({
val x = 1 + 1
x + 1
}) // 3
Functions
Functions are expressions that take parameters.
You can define an anonymous function (i.e. no name) that returns a given integer plus one:
(x: Int) => x + 1
On the left of =>
is a list of parameters. On the right is an expression involving the parameters.
You can also name functions.
val addOne = (x: Int) => x + 1
println(addOne(1)) // 2
Functions may take multiple parameters.
val add = (x: Int, y: Int) => x + y
println(add(1, 2)) // 3
Or it can take no parameters.
val getTheAnswer = () => 42
println(getTheAnswer()) // 42
Methods
Methods look and behave very similar to functions, but there are a few key differences between them.
Methods are defined with the def
keyword. def
is followed by a name, parameter lists, a return type, and a body.
def add(x: Int, y: Int): Int = x + y
println(add(1, 2)) // 3
Notice how the return type is declared after the parameter list and a colon : Int
.
Methods can take multiple parameter lists.
def addThenMultiply(x: Int, y: Int)(multiplier: Int): Int = (x + y) * multiplier
println(addThenMultiply(1, 2)(3)) // 9
Or no parameter lists at all.
def name: String = System.getProperty("user.name")
println("Hello, " + name + "!")
There are some other differences, but for now, you can think of them as something similar to functions.
Methods can have multi-line expressions as well.
def getSquareString(input: Double): String = {
val square = input * input
square.toString
}
The last expression in the body is the method’s return value. (Scala does have a return
keyword, but it’s rarely used.)
In Scala, all values have a type, including numerical values and functions. The diagram below illustrates a subset of the type hierarchy.
Scala Type Hierarchy
Any
is the supertype of all types, also called the top type. It defines certain universal methods such as equals
, hashCode
, and toString
. Any
has two direct subclasses: AnyVal
and AnyRef
.
AnyVal
represents value types. There are nine predefined value types and they are non-nullable: Double
, Float
, Long
, Int
, Short
, Byte
, Char
, Unit
, and Boolean
. Unit
is a value type which carries no meaningful information. There is exactly one instance of Unit
which can be declared literally like so: ()
. All functions must return something so sometimes Unit
is a useful return type.
AnyRef
represents reference types. All non-value types are defined as reference types. Every user-defined type in Scala is a subtype of AnyRef
. If Scala is used in the context of a Java runtime environment, AnyRef
corresponds to java.lang.Object
.
Here is an example that demonstrates that strings, integers, characters, boolean values, and functions are all objects just like every other object:
val list: List[Any] = List(
"a string",
732, // an integer
'c', // a character
true, // a boolean value
() => "an anonymous function returning a string"
)
list.foreach(element => println(element))
It defines a variable list
of type List[Any]
. The list is initialized with elements of various types, but they all are instance of scala.Any
, so you can add them to the list.
Here is the output of the program:
a string
732
c
true
<function>
Type Casting
Value types can be cast in the following way:
For example:
val x: Long = 987654321
val y: Float = x // 9.8765434E8 (note that some precision is lost in this case)
val face: Char = ''
val number: Int = face // 9786
Casting is unidirectional. This will not compile:
val x: Long = 987654321
val y: Float = x // 9.8765434E8
val z: Long = y // Does not conform
You can also cast a reference type to a subtype. This will be covered later in the tour.
Nothing and Null
Nothing
is a subtype of all types, also called the bottom type. There is no value that has type Nothing
. A common use is to signal non-termination such as a thrown exception, program exit, or an infinite loop (i.e., it is the type of an expression which does not evaluate to a value, or a method that does not return normally).
Null
is a subtype of all reference types (i.e. any subtype of AnyRef). It has a single value identified by the keyword literal null
. Null
is provided mostly for interoperability with other JVM languages and should almost never be used in Scala code. We’ll cover alternatives to null
later in the tour.
Here, I would like to introduce a book – Programming in Scala
This book mostly covers Scala’s basic and some advanced concepts and how Scala has adopted functional programming. In this book, you will find more examples than theories and concepts that will help you to understand the concept easily. Apart from basic Scala concepts, you shall learn how to program in Scala with deep-diving into the object-oriented and functional approach of solving problems using Scala.
This book contains live runnable examples for each concept explained. One doesn’t need to search in google or waste time on searching different unrelated sources for learning the concept of Scala.
Conclusion
In this Scala tour, we learned about what Scala is and the purpose of using Scala. We went through the features of Scala and installation of Scala. Also, we have learned some basic concepts of Scala in which we covered expressions, values, variables,methods and functions. We also covered the type hierarchy in Scala.
With that I would conclude this blog, I hope that this was informative and easy to understand, also, if you have any queries please feel free to list them down in the comments section.
If you would like to learn what’s new in Scala, read this fabulous blog on Scala 3.0: What’s New?
If you are looking to start your project with Scala and don’t know where to start, shoot us a message. Knoldus has been working on Scala, Akka, and Play since 2011. We love its support for functional programming and reactive architecture. Years of experience in the Scala programming language means we can support your business in any of your Scala-related projects. We support the end-to-end software development cycle and let you focus on the vision and business aspects of the product.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK