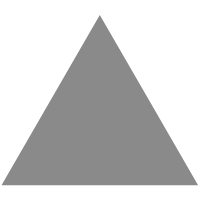
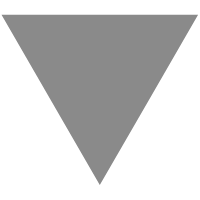
Ajax基础 --学习记录(三)
source link: https://segmentfault.com/a/1190000039803676
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
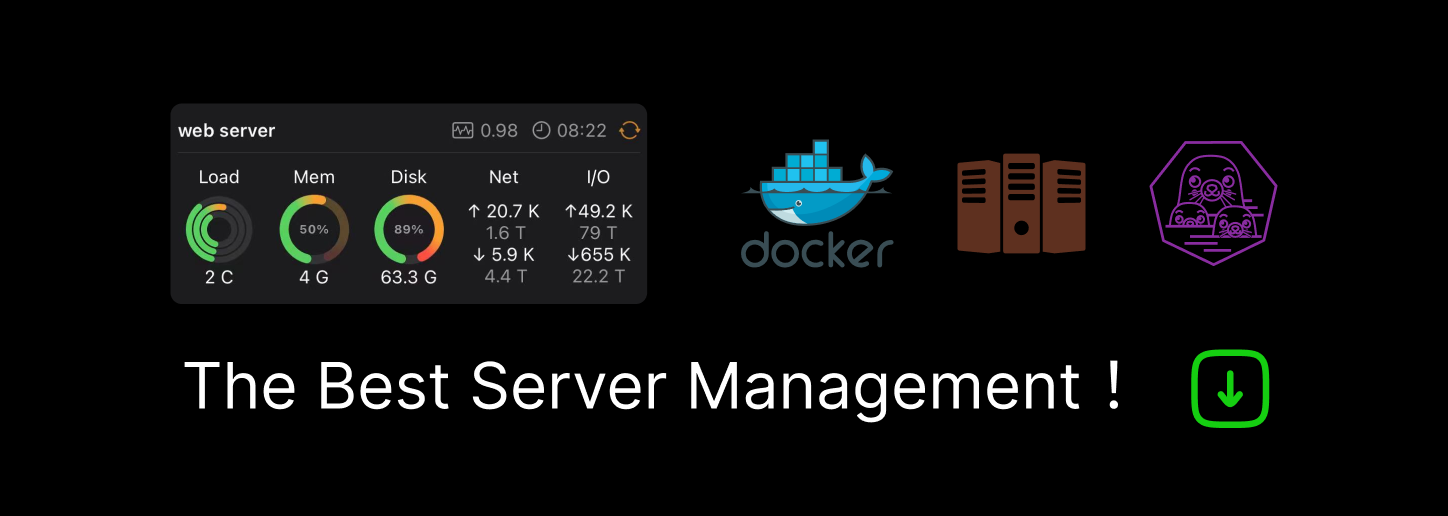
jQuery 中的 Ajax
$.ajax()
$.ajax()方法概述
作用:发送Ajax请求。
$.ajax({
type: 'get',
url: 'http://www.example.com',
data: { name: 'zhangsan', age: '20' },
//data: 'name=zhangsan&age=20'
contentType: 'application/x-www-form-urlencoded',
//contentType: 'application/json'
beforeSend: function () {
return false
},
success: function (response) {},
error: function (xhr) {}
});
beforeSend方法说明
$.ajax({
// 请求方式
type: 'post',
// 请求地址
url: '/user',
// 在请求发送之前调用
beforeSend: function () {
alert('请求不会被发送')
// 请求不会被发送
return false;
},
// 请求成功以后函数被调用
success: function (response) {
// response为服务器端返回的数据
// 方法内部会自动将json字符串转换为json对象
console.log(response);
}
})
作用:发送jsonp请求。
$.ajax({
url: 'http://www.example.com',
// 指定当前发送jsonp请求
dataType: 'jsonp',
// 修改callback参数名称
jsonp: 'cb',
// 指定函数名称
jsonCallback: 'fnName',
success: function (response) {}
})
serialize方法
作用:将表单中的数据自动拼接成字符串类型的参数
var params = $('#form').serialize();
// name=zhangsan&age=30
$.get()、$.post
$.get()、$.post()方法概述
作用:$.get方法用于发送get请求,$.post方法用于发送post请求。
$.get('http://www.example.com', {name: 'zhangsan', age: 30}, function (response) {})
$.post('http://www.example.com', {name: 'lisi', age: 22}, function (response) {})
jQuery中Ajax全局事件
只要页面中有Ajax请求被发送,对应的全局事件就会被触发
.ajaxStart() // 当请求开始发送时触发
.ajaxComplete() // 当请求完成时触发
NProgress
官宣:纳米级进度条,使用逼真的涓流动画来告诉用户正在发生的事情!
<link rel='stylesheet' href='nprogress.css'/>
<script src='nprogress.js'></script>
NProgress.start(); // 进度条开始运动
NProgress.done(); // 进度条结束运动
RESTful 风格的 API
传统请求地址回顾
GET http://www.example.com/getUsers // 获取用户列表
GET http://www.example.com/getUser?id=1 // 比如获取某一个用户的信息
POST http://www.example.com/modifyUser // 修改用户信息
GET http://www.example.com/deleteUser?id=1 // 删除用户信息
RESTful API 概述
一套关于设计请求的规范。
GET: 获取数据
POST: 添加数据
PUT: 更新数据
DELETE: 删除数据
RESTful API 的实现
每一个请求地址都要对应资源
users => /users
articles => /articles
GET:http://www.example.com/users
获取用户列表数据
POST:http://www.example.com/users
创建(添加)用户数据
GET:http://www.example.com/users/1
获取用户ID为1的用户信息
PUT:http://www.example.com/users/1
修改用户ID为1的用户信息
DELETE:http://www.example.com/users/1
删除用户ID为1的用户信息
注意:传统的html表单提交不支持put和delete,在Ajax中支持
服务器端:
// 获取用户列表信息
app.get('/users', (req, res) => {
res.send('当前是获取用户列表信息的路由');
});
// 获取某一个用户具体信息的路由
app.get('/users/:id', (req, res) => {
// 获取客户端传递过来的用户id
const id = req.params.id;
res.send(`当前我们是在获取id为${id}用户信息`);
});
// 删除某一个用户
app.delete('/users/:id', (req, res) => {
// 获取客户端传递过来的用户id
const id = req.params.id;
res.send(`当前我们是在删除id为${id}用户信息`);
});
// 修改某一个用户的信息
app.put('/users/:id', (req, res) => {
// 获取客户端传递过来的用户id
const id = req.params.id;
res.send(`当前我们是在修改id为${id}用户信息`);
});
<script type="text/javascript">
// 获取用户列表信息
$.ajax({
type: 'get',
url: '/users',
success: function (response) {
console.log(response)
}
})
// 获取id为1的用户信息
$.ajax({
type: 'get',
url: '/users/1',
success: function (response) {
console.log(response)
}
})
// 删除id为10的用户信息
$.ajax({
type: 'delete',
url: '/users/10',
success: function (response) {
console.log(response)
}
})
// 修改id为1的用户信息
$.ajax({
type: 'put',
url: '/users/10',
success: function (response) {
console.log(response)
}
})
</script>
XML 基础
XML是什么
XML 的全称是 extensible markup language,代表可扩展标记语言,它的作用是传输和存储数据。
<students>
<student>
<sid>001</sid>
<name>张三</name>
</student>
<student>
<sid>002</sid>
<name>王二丫</name>
</student>
</students>
app.get('/xml', (req, res) => {
res.header('content-type', 'text/xml');
res.send('<message><title>消息标题</title><content>消息内容</content></message>')
});
XML DOM
XML DOM 即 XML 文档对象模型,是 w3c 组织定义的一套操作 XML 文档对象的API。浏览器会将 XML 文档解析成文档对象模型。
btn.onclick = function () {
var xhr = new XMLHttpRequest();
xhr.open('get', '/xml');
xhr.send();
xhr.onload = function () {
// xhr.responseXML 获取服务器端返回的xml数据
var xmlDocument = xhr.responseXML;
var title = xmlDocument.getElementsByTagName('title')[0].innerHTML;
container.innerHTML = title;
}
}
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK