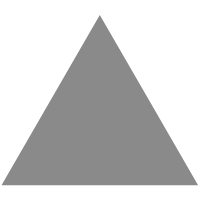
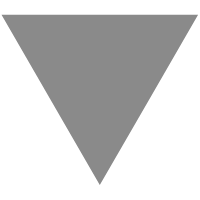
3 Ways to Merge Arrays in JavaScript
source link: https://dmitripavlutin.com/javascript-merge-arrays/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
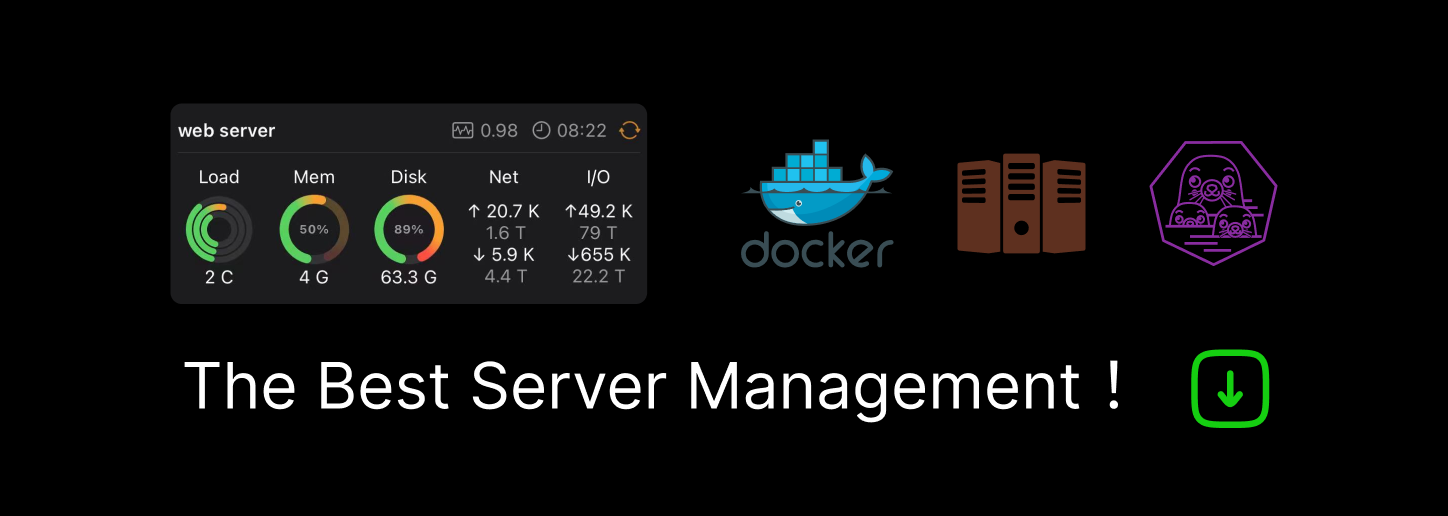
3 Ways to Merge Arrays in JavaScript
An array is a data structure representing an ordered collection of indexed items.
A common operation performed on multiple arrays is merge — when 2 or more arrays are merged to form a bigger array containing all the items of the merged arrays.
For example, having two arrays [1, 2]
and [5, 6]
, then merging these arrays results in [1, 2, 5, 6]
.
In this post, you’ll find 3 ways to merge arrays in JavaScript: 2 immutable (a new array is created after the merge) and 1 mutable (items are merged into an array).
1. Immutable merge of arrays
1.1 Merge using the spread operator
If you want to know one but a good way to merge arrays in JavaScript, then you should remember the merge using the spread operator.
Write inside the array literal two or more arrays prefixed with the spread operator ...
, and JavaScript is going to create a new array containing all the items of the arrays:
// merge array1 and array2
const mergeResult = [...array1, ...array2];
For example, consider the following 2 arrays heros
and villains
:
const heroes = ['Batman', 'Superman'];
const villains = ['Joker', 'Bane'];
const all = [...heroes, ...villains];
all; // ['Batman', 'Superman', 'Joker', 'Bane']
const all = [...heros, ...villains]
creates a new array having heros
and villains
arrays merged.
The order of merged arrays inside the array literal does matter: items of the merged arrays are inserted in the same order as the arrays appear inside the literal.
For example, let’s put the list of villains before the list of heroes in the merged array:
const heroes = ['Batman', 'Superman'];
const villains = ['Joker', 'Bane'];
const all = [...villains, ...heroes];
all; // ['Joker', 'Bane', 'Batman', 'Superman']
The spread operator approach lets you merge 2 and even more arrays at once:
const mergeResult = [...array1, ...array2, ...array3, ...arrayN];
1.2 Merge using array.concat() method
If you prefer a functional way to merge arrays, then you can use the array1.concat(array2)
method:
// merge array1 and array2
const mergeResult = array1.concat(array2);
or using another form:
// merge array1 and array2
const mergeResult = [].concat(array1, array2);
The array.concat()
method doesn’t mutate the array upon which it is called but returns a new array having the merge result.
Let’s use array.concat()
to merge the heroes
and villains
:
const heroes = ['Batman', 'Superman'];
const villains = ['Joker', 'Bane'];
const all1 = heroes.concat(villains);
const all2 = [].concat(heroes, villains);
all1; // ['Batman', 'Superman', 'Joker', 'Bane']
all2; // ['Batman', 'Superman', 'Joker', 'Bane']
heroes.concat(villains)
as well as [].concat(heros, villains)
return a new array where heros
and villains
arrays are merged.
The concat method accepts multiple arrays as arguments, thus you can merge 2 or more arrays at once:
const mergeResult = [].concat(array1, array2, array3, arrayN);
2. Mutable merge of arrays
The merge performed using the spread operator or array.concat()
creates a new array. However, sometimes you don’t want to create a new array, but rather merge it into some existing array.
The approach below performs a mutable way to merge arrays.
2.1 Merge using array.push() method
You might know already that array.push(item)
method pushes an item at the end of the array, mutating the array upon which the method is called:
const heroes = ['Batman'];
heroes.push('Superman');
heroes; // ['Batman', 'Superman']
Thanks to the fact that array.push(item1, item2, ..., itemN)
accepts multiple items to push, you can push an entire array using the spread operator applied to arguments (in other words, performing a merge into):
// merge array2 into array1
array1.push(...array2);
For example, let’s merge villains
into heros
arrays:
const heroes = ['Batman', 'Superman'];
const villains = ['Joker', 'Bane'];
heroes.push(...villains);
heroes; // ['Batman', 'Superman', 'Joker', 'Bane']
heroes.push(...villains)
pushes all the items of villains
array at the end of heroes
array — performing a mutable merge. heros
array is mutated.
Side challenge: what expression would you use to push multiple arrays at once? Share your solution in a comment below!
3. Conclusion
JavaScript offers multiple ways to merge arrays.
You can use either the spread operator [...array1, ...array2]
, or a functional way [].concat(array1, array2)
to merge 2 or more arrays. These approaches are immutable because the merge result is stored in a new array.
If you’d like to perform a mutable merge, i.e. merge into an array without creating a new one, then you can use array1.push(...array2)
approach.
What other ways to merge arrays do you know?
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK