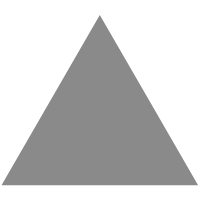
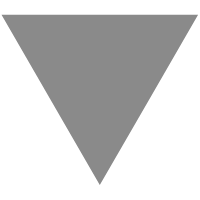
Speech to the text: NullPointerException
source link: https://www.codesd.com/item/speech-to-the-text-nullpointerexception.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
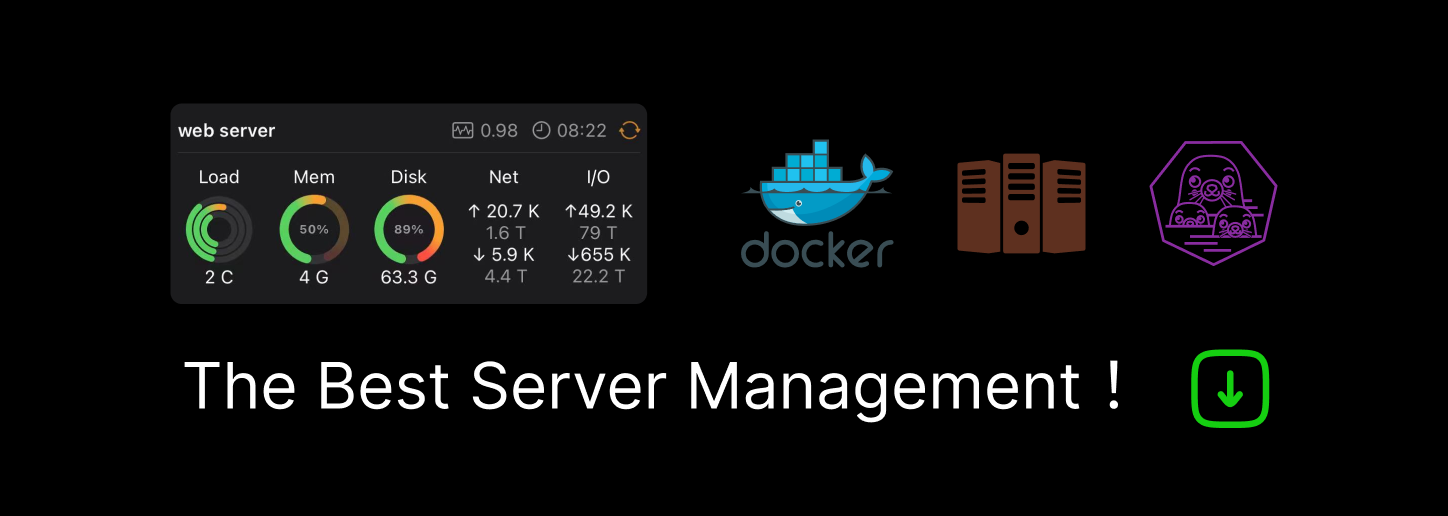
Speech to the text: NullPointerException
Please have a look at the following code
package com.ace.voicebuddy;
import java.util.ArrayList;
import android.os.Bundle;
import android.app.Activity;
import android.content.ActivityNotFoundException;
import android.content.Intent;
import android.speech.RecognizerIntent;
import android.view.Menu;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.EditText;
import android.widget.ImageView;
import android.widget.Toast;
public class VoiceNotes extends Activity {
private ImageView speakNow;
private EditText voiceEdt;
private static final int RESULT_SPEECH = 1;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_voice_notes);
//Intialize instance variables
speakNow = (ImageView)findViewById(R.id.speak_now);
voiceEdt = (EditText)findViewById(R.id.voice_to_text_edt);
//Registering Event Handlers
speakNow.setOnClickListener(new SpeakNowEvent());
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.voice_notes, menu);
return true;
}
//Event handler for Speak Now button
private class SpeakNowEvent implements OnClickListener
{
@Override
public void onClick(View arg0) {
// TODO Auto-generated method stub
Intent intent = new Intent(RecognizerIntent.ACTION_RECOGNIZE_SPEECH);
intent.putExtra(RecognizerIntent.EXTRA_LANGUAGE_MODEL, "en-US");
try
{
startActivityForResult(intent,RESULT_SPEECH);
}
catch(ActivityNotFoundException e)
{
Toast.makeText(VoiceNotes.this, "Ooops! Your device is not suppoting Speech to Text!", Toast.LENGTH_LONG).show();
}
}
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data)
{
super.onActivityResult(requestCode, resultCode, data);
if(RESULT_SPEECH==requestCode)
{
ArrayList<String> text = data.getStringArrayListExtra(RecognizerIntent.EXTRA_RESULTS);
voiceEdt.setText(text.get(0));
}
}
}
This is the first time I am working with Speech to text in android so some methods like "onActivityResult" are pretty new to me. As soon as I run this code, I get the following error
11-13 10:23:52.968: E/AndroidRuntime(1148): FATAL EXCEPTION: main
11-13 10:23:52.968: E/AndroidRuntime(1148): java.lang.RuntimeException: Failure delivering result ResultInfo{who=null, request=1, result=0, data=null} to activity {com.ace.voicebuddy/com.ace.voicebuddy.VoiceNotes}: java.lang.NullPointerException
11-13 10:23:52.968: E/AndroidRuntime(1148): at android.app.ActivityThread.deliverResults(ActivityThread.java:3319)
11-13 10:23:52.968: E/AndroidRuntime(1148): at android.app.ActivityThread.handleSendResult(ActivityThread.java:3362)
11-13 10:23:52.968: E/AndroidRuntime(1148): at android.app.ActivityThread.access$1100(ActivityThread.java:141)
11-13 10:23:52.968: E/AndroidRuntime(1148): at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1282)
11-13 10:23:52.968: E/AndroidRuntime(1148): at android.os.Handler.dispatchMessage(Handler.java:99)
11-13 10:23:52.968: E/AndroidRuntime(1148): at android.os.Looper.loop(Looper.java:137)
11-13 10:23:52.968: E/AndroidRuntime(1148): at android.app.ActivityThread.main(ActivityThread.java:5041)
11-13 10:23:52.968: E/AndroidRuntime(1148): at java.lang.reflect.Method.invokeNative(Native Method)
11-13 10:23:52.968: E/AndroidRuntime(1148): at java.lang.reflect.Method.invoke(Method.java:511)
11-13 10:23:52.968: E/AndroidRuntime(1148): at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:793)
11-13 10:23:52.968: E/AndroidRuntime(1148): at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:560)
11-13 10:23:52.968: E/AndroidRuntime(1148): at dalvik.system.NativeStart.main(Native Method)
11-13 10:23:52.968: E/AndroidRuntime(1148): Caused by: java.lang.NullPointerException
11-13 10:23:52.968: E/AndroidRuntime(1148): at com.ace.voicebuddy.VoiceNotes.onActivityResult(VoiceNotes.java:77)
11-13 10:23:52.968: E/AndroidRuntime(1148): at android.app.Activity.dispatchActivityResult(Activity.java:5293)
11-13 10:23:52.968: E/AndroidRuntime(1148): at android.app.ActivityThread.deliverResults(ActivityThread.java:3315)
11-13 10:23:52.968: E/AndroidRuntime(1148): ... 11 more
What I have done wrong here? Please help.
Your app is crashing because
In your onActivityResult
method your data
(Object of Intent ) is null and you are trying to get something form null
.
ArrayList<String> text = data.getStringArrayListExtra(RecognizerIntent.EXTRA_RESULTS);
Update your onActivityResult()
method like this
if(RESULT_SPEECH==requestCode)
{
if (data!=null)
{
ArrayList<String> text = data.getStringArrayListExtra(RecognizerIntent.EXTRA_RESULTS);
voiceEdt.setText(text.get(0));
}
else
Toast.makeText(ActivityMain.this,"No data recieved", 1).show();
}
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK