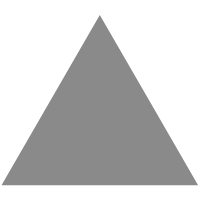
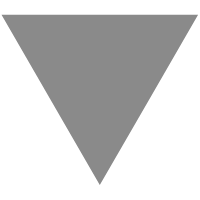
7 Interview Questions on "this" keyword in JavaScript. Can You Answer...
source link: https://dmitripavlutin.com/javascript-this-interview-questions/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
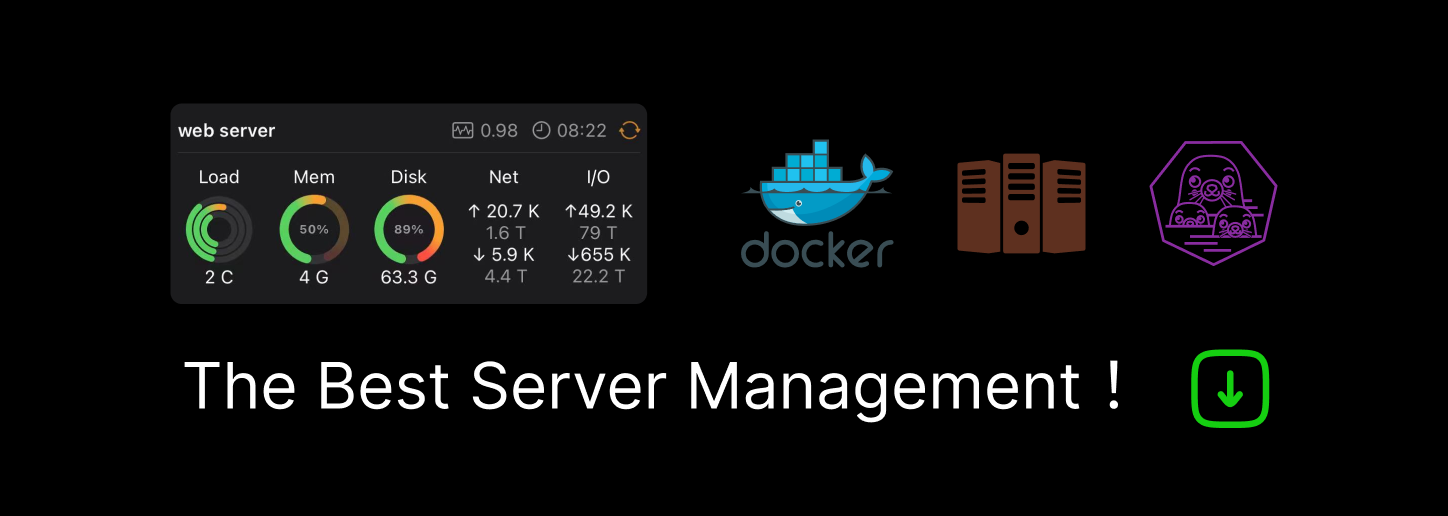
7 Interview Questions on "this" keyword in JavaScript. Can You Answer Them?
In JavaScript this
is the function invocation context.
The challenge is that this
has a complicated behavior. That’s why during a JavaScript coding interview you might be asked how this
behaves in certain situations.
Since the best way to prepare for a coding interview is to practice, in this post I compiled a list of 7 interesting interview questions on this
keyword.
If you’re not familiar with this
keyword, I recommend reading the post Gentle Explanation of “this” in JavaScript before continuing.
Note: JavaScript snippets below run in non-strict mode, also known as sloppy mode.
Question 1: Variable vs property
What logs to console the following code snippet:
const object = {
message: 'Hello, World!',
getMessage() {
const message = 'Hello, Earth!';
return this.message;
}
};
console.log(object.getMessage()); // What is logged?
Expand answer
Question 2: Cat name
What logs to console the following code snippet:
function Pet(name) {
this.name = name;
this.getName = () => this.name;
}
const cat = new Pet('Fluffy');
console.log(cat.getName()); // What is logged?
const { getName } = cat;
console.log(getName()); // What is logged?
Expand answer
Question 3: Delayed greeting
What logs to console the following code snippet:
const object = {
message: 'Hello, World!',
logMessage() {
console.log(this.message); // What is logged?
}
};
setTimeout(object.logMessage, 1000);
Expand answer
Question 4: Artificial method
How can you call logMessage
function so that it logs "Hello, World!"
?
const object = {
message: 'Hello, World!'
};
function logMessage() {
console.log(this.message); // "Hello, World!"
}
// Write your code here...
Expand answer
Question 5: Greeting and farewell
What logs to console the following code snippet:
const object = {
who: 'World',
greet() {
return `Hello, ${this.who}!`;
},
farewell: () => {
return `Goodbye, ${this.who}!`;
}
};
console.log(object.greet()); // What is logged?
console.log(object.farewell()); // What is logged?
Expand answer
Question 6: Tricky length
What logs to console the following code snippet:
var length = 4;
function callback() {
console.log(this.length); // What is logged?
}
const object = {
length: 5,
method(callback) {
callback();
}
};
object.method(callback, 1, 2);
Expand answer
Question 7: Calling arguments
What logs to console the following code snippet:
var length = 4;
function callback() {
console.log(this.length); // What is logged?
}
const object = {
length: 5,
method() {
arguments[0]();
}
};
object.method(callback, 1, 2);
Expand answer
Summary
If you’ve answered correctly 5 or more questions, then you have a good understanding of this
keyword!
Otherwise, you need a good refresher on this
keyword. I recommend revising the post Gentle Explanation of “this” in JavaScript.
Ready for a new challenge? Try to solve the 7 Interview Questions on JavaScript Closures.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK