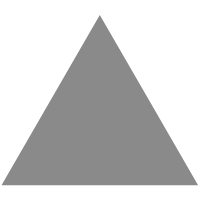
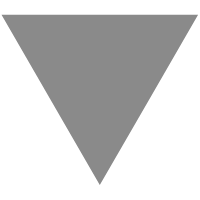
如何将元素插入数组的指定索引?
source link: http://database.51cto.com/art/202102/646233.htm
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
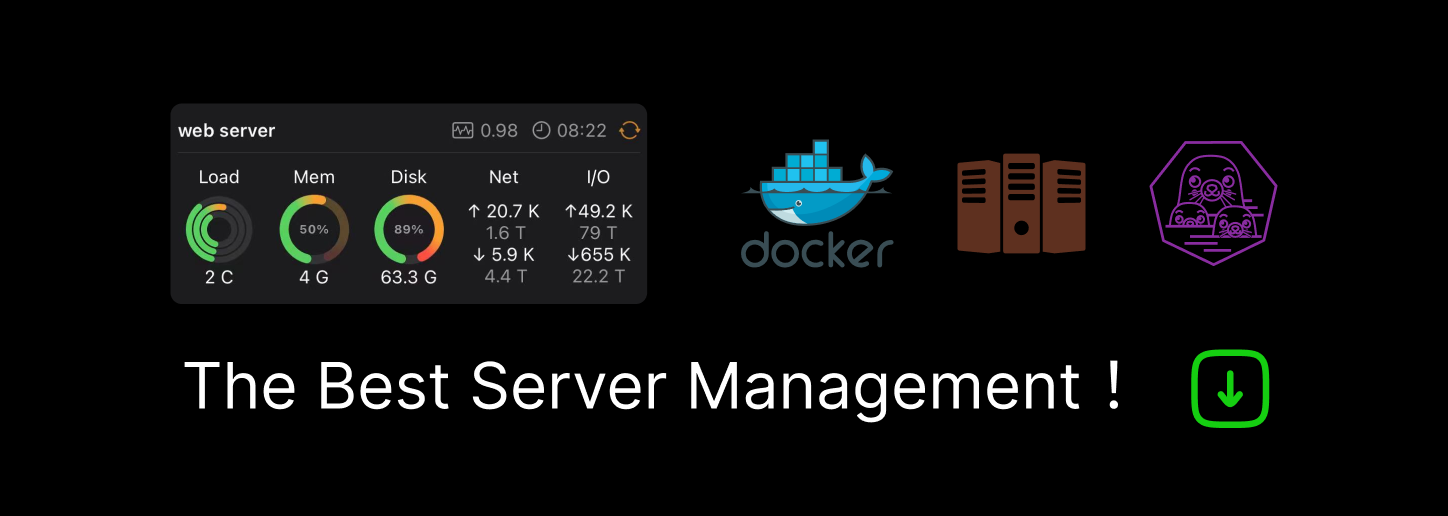
本文已经过原作者 Guest Contributor 授权翻译!
简介
数组是一种线性数据结构,可以说是编程中最常用的数据结构之一。修改数组是一种常见的操作,这里,我们来讨论如何在 JS 中数组的任何位置添加元素。
元素可以添加到数组中的三个位置
- 开始/第一个元素
- 结束/最后元素
- 其他地方
1111
接着,我们一个一个过一下:
数组对象中的unshift()方法将一个或多个元素添加到数组的开头,并返回数组的新长度:
const startArray = [3, 4, 5]; const newLength = startArray.unshift(2); console.log(newLength); console.log(startArray); startArray.unshift(-1, 0, 2); console.log(startArray);
上面输出的结果:
4 [ 2, 3, 4, 5 ] [ -1, 0, 2, 2, 3, 4, 5 ]
将元素添加到数组的末尾
使用数组的最后一个索引
要在数组末尾添加元素,可以使用数组的长度总是比下标小1这一技巧。
const indexArray = [1, 2, 3]; console.log(indexArray.length); console.log(indexArray[2]); console.log(indexArray[3]); indexArray[indexArray.length] = 4 console.log(indexArray);
上面输出的结果:
3 3 undefined [ 1, 2, 3, 4 ]
数组的长度是3,第二个元素是3。没有第三个元素,所以我们用undefined开头。最后,在该位置插入值4。
使用 push() 方法
数组的push()方法将一个或多个元素添加到数组的末尾。就像unshift()一样,它也会返回数组的新长度
const pushArray = [1, 2, 3] const newLength = pushArray.push(4, 5, 6, 7); console.log(newLength); console.log(pushArray);
上面输出的结果:
7 [ 1, 2, 3, 4, 5, 6, 7 ]
使用 concat() 方法
通过数组的concat()方法实现两个或更多数组的合并。它创建新的副本,且不影响原始数组。与以前的方法不同,它返回一个新数组。使用该方法,要连接的值始终位于数组的末尾。
const example1Array1 = [1, 2, 3]; const valuesToAdd = [4, 5, 6]; const example1NewArray = example1Array1.concat(valuesToAdd); console.log(example1NewArray); console.log(example1Array1);
上面输出的结果:
[ 1, 2, 3, 4, 5, 6 ] [ 1, 2, 3 ]
我们可以将一个数组与一系列值连接起来:
const array = [1,2,3]; const newArray = array.concat('12', true, null, 4,5,6,'hello'); console.log(array); console.log(newArray);
上面输出的结果:
[ 1, 2, 3 ] [ 1, 2, 3, '12', true, null, 4, 5, 6, 'hello' ]
可以将一个数组与多个数组连接起来:
const array1 = [1, 2, 3]; const array2 = [4, 5, 6]; const array3 = [7, 8, 9]; const oneToNine = array1.concat(array2, array3); console.log(oneToNine);
上面输出的结果:
[ 1, 2, 3, 4, 5, 6, 7, 8, 9 ]
在数组的任何位置添加元素
现在我们将讨论一个masterstroke方法,它可以用于在数组的任何位置添加元素——开始、结束、中间和中间的任何位置。
splice()方法添加,删除和替换数组中的元素。它通常用于数组管理,此方法不会创建新数组,而是会更新调用它的数组。
我们来看看splice()的实际应用。这里有一个weekdays数组,现在,我们想在'周二'和'周四'之间添加一个'周三'元素
const weekdays = ['周一', '周三', '周四', '周五'] const deletedArray = weekdays.splice(2, 0, '周二'); console.log(weekdays); console.log(deletedArray);
上面输出的结果:
["周一", "周二", "周三", "周四", "周五"] []
分析一下上面的代码。我们想在weekdays数组的第二个位置添加'周二'。这里不需要删除任何元素。weekdays.splice(2, 0, 'wednesday')被读取为第二个位置,不移除任何元素并添加'周二'。
下面是使用splice()的一般语法:
let removedItems = array.splice(start[, deleteCount[, item1[, item2[, ...]]]])
- start-开始修改数组的索引。
- deleteCount -从start 删除的数组中可选的项目数。如果省略,则start后的所有项目都将被删除。
- item1, item2, ...-从start 添加到数组的可选项目。如果省略,它将仅从数组中删除元素。
我们看一下slice()的另一个示例,在该示例中我们同时添加和删除数组。我们将在第二个位置添加 '周三',但是我们还将在该处删除错误的周末值:
const weekdays = ['周一', '周三', '周六', '周日', '周四', '周五'] const deletedArray = weekdays .splice(2, 2, '周二'); console.log(weekdays); console.log(deletedArray);
上面输出的结果:
["周一", "周三", "周二", "周四", "周五"] ["周六", "周日"]
总结
在本文中,我们研究了 JS 中可以向数组添加元素的多种方法。我们可以使用 unshift()将它们添加到开头。我们可以使用索引,pop()方法和concat()方法将它们添加到末尾。通过splice()方法,我们可以更好地控制它们的放置位置。
完~ 我是小智,我要去刷碗了,我们下期见~
作者:Guest Contributor 译者:前端小智 来源:stackabuse原文:https://stackabse.com/javascript-how-to-inser-elements-into-a-specific-index-of-an-array/
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK