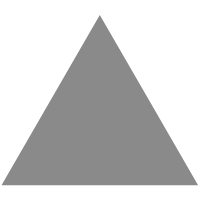
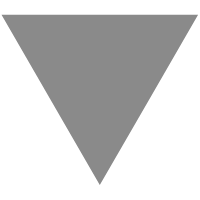
即时消息 - 时信魔方教程
source link: http://www.cnblogs.com/shixincube/p/14226585.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
文:徐江威
时信魔方
的即时消息通过 MessagingService 模块来操作。在 Cube 启动之后使用 MessagingService 的 sendTo
、 sendToContact
或者 sendToGroup
向指定的联系人或群组发送消息。通过加入事件监听器来接收 MessagingService 的事件,从而实现接收来自其他联系人或者群组的消息。
启动 Cube Engine
一般实例化 Cube Engine 之后,通过配置相关的域参数即可启动 Cube 。
Cube 支持多域管理,因此每个客户端设备启动 Cube 时都需要标记自己所在的域信息,这些信息包括 Cube 的网关机地址、域信息和 App Key 等。例如:
{ "address": "127.0.0.1", "domain": "shixincube.com", "appKey": "shixin-cubeteam-opensource-appkey" }
这个 JSON 表示的配置信息就包括服务器的地址 address
,所在域的名称 domain
,以及这个域里的 appKey
识别串。
各个客户端的启动代码如下:
Web 版
const cube = window.cube(); const config = { "address": "127.0.0.1", "domain": ... }; cube.start(config, function() { console.log('Cube 启动成功'); }, function() { console.log('Cube 启动错误'); });
Android 版
KernelConfig config = new KernelConfig(); config.address = "127.0.0.1"; config.domain = "shixincube.com"; config.appKey = "shixin-cubeteam-opensource-appkey"; CubeEngine.getInstance().start(context, config);
iOS 版
CKernelConfig *config = [[CKernelConfig alloc] init]; config.domain = @"shixincube.com"; config.appKey = @"shixin-cubeteam-opensource-appkey"; config.address = @"192.168.1.113"; [[CEngine shareEngine] startWithConfig:config];
账号签入
成功启动 Cube 之后,就需要为终端指定账号了,以便告诉服务器“我是谁”。
Cube 的账号由 ContactService 模块管理,一般来说,使用 Cube 不需要预先进行“账号注册”,也就是说应用程序可以指定任意一个 long
型 ID 为账号的 ID ,无需先进行这个 ID 的注册和添加,这样可以方便应用程序快速绑定一个账号到 Cube 。
例如如果应用程序当前登录的账号 ID 是 102030405
,那么可以直接将 Cube 的签入联系人 ID 设置为 102030405
,实现 Cube ID 和应用程序账号一致,以便管理。
签入联系示例代码如下:
Web 版
let accountId = 102030405; let accountName = 'MyApp用户的显示名'; cube.signIn(accountId, accountName);
Android 版
long accountId = 102030405; String accountName = 'MyApp用户的显示名'; CubeEngine.getInstance().getService(ContactService.class).signIn(accountId, accountName, new CubeCallback<Contact>() { @Override public void onSuccess(Contact result) { Toast.makeText(MainActivity.this, "账号设置成功", Toast.LENGTH_LONG).show(); } @Override public void onFailure(int code, String desc) { } });
iOS 版
// 签入账号 [[CEngine shareEngine] signIn:@"102030405" name:@"MyApp用户的显示名"];
发送消息
签入账号之后即可向指定的账号发送消息。直接将实例化的 Message
对象通过 MessagingService 模块发送给指定用户。
Web 版
// 实例化消息 let message = new Message({ "content": "这里是我想说的话!" }); // 发送给 ID 为 908070605 的联系人 cube.messaging.sendToContact(908070605, message);
Android 版
JSONObject payload = new JSONObject(); payload.put("content", "这里是我想说的话!"); Message message = new Message(payload); // 获取消息服务 MessagingService messaging = CubeEngine.getInstance().getService(MessagingService.class); // 发送给 ID 为 908070605 的联系人 messaging.sendToContact(908070605L, message, new CubeCallback<Message>() { @Override public void onSuccess(Message result) { Toast.makeText(MainActivity.this, "发送消息:" + message.getPayload(), Toast.LENGTH_LONG).show(); } @Override public void onFailure(int code, String desc) { Toast.makeText(MainActivity.this, "发送失败:" + desc, Toast.LENGTH_LONG).show(); } });
iOS 版
// 获取消息传输服务 CMessagingService *messaging = (CMessagingService *)[[CKernel shareKernel] getModule:CMessagingService.mName]; // 实例化消息 CMessage *message = [[CMessage alloc] initWithPayload:@{@"content":content}]; // 发送给指定联系人 [messaging sendToContact:908070605L message:message];
至此,我们就通过短短的几行代码就完成了即时消息的发送。
监听消息事件
如何接收来自其他人发送的消息呢?
Cube 通过回调监听器方法的方式来进行事件通知,包括接收到新消息、消息是否已经成功发送等事件。向 MessagingService 模块添加监听 Notify
事件的监听器或函数来接收消息:
Web 版
// 监听 Notify 事件 cube.messaging.on(MessagingEvent.Notify, onNotify); function onNotify(event) { let message = event.getData(); console.log('接收到来自:' + message.getFrom() + ' 的消息'); }
Android 版
class MyMessageListener implements MessagingListener { @Override public void onNotify(Message message) { Toast.makeText(MainActivity.this, "收到消息:" + message.getPayload(), Toast.LENGTH_LONG).show(); } }
iOS 版
// 监听 Notify 事件 [[CEngine shareEngine].messagingService attach:self]; // 主要事件 -(void)update:(CObserverState *)state{ if ([state.name isEqualToString:MessagingEvent::Notify]) { NSLog(@"recv message ..."); } }
除了 Notify
事件,每个模块都有不同的事件,通过这些事件可以方便的进行数据管理和对程序进行数据更新。具体的事件可以查看各个客户端的文档:
[完]
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK