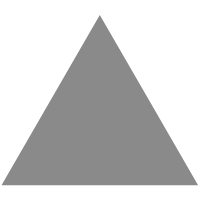
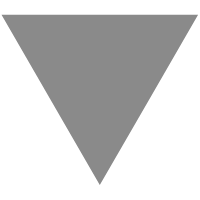
Create, Upload & Delete Google Docs using Play Scala application
source link: https://blog.knoldus.com/create-upload-delete-google-docs-using-play-scala-application/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
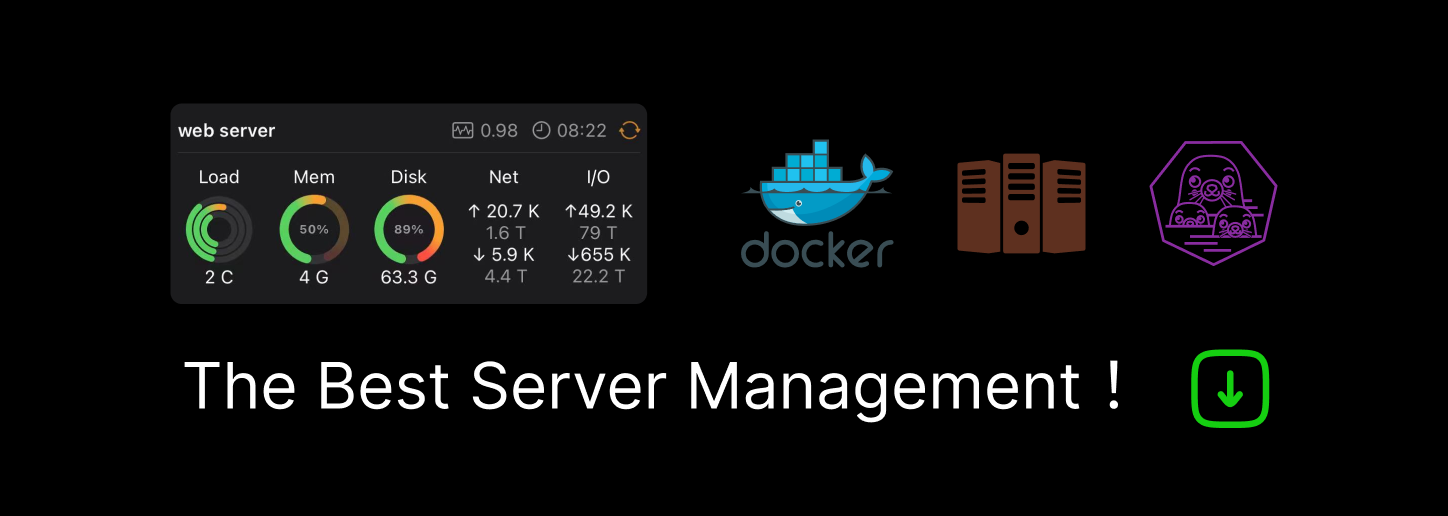
Create, Upload & Delete Google Docs using Play Scala application
Reading Time: 2 minutes
This blog talks about creating, uploading & deleting Google Docs using a Play Scala application. We have used oauth2 in order to communicate with the Google Drive to perform various Google Doc operations. Let us see the process step by step.
1.First integrate Google Drive infrastructure in your Play Scala Application. You can read about it from here to know how to do it.
2. Then in order to use Google Drive, first you have to prepare it. Now, to prepare it you have add this code.
xxxxxxxxxx
val CLIENT_ID = "your_app's_client_id"
val CLIENT_SECRET = "your_app's_client_secret"
val httpTransport = new NetHttpTransport
val jsonFactory = new JacksonFactory
// Preparing Google Drive
def prepareGoogleDrive(accessToken: String): Drive = {
//Build the Google credentials and make the Drive ready to interact
val credential = new GoogleCredential.Builder()
.setJsonFactory(jsonFactory)
.setTransport(httpTransport)
.setClientSecrets(CLIENT_ID, CLIENT_SECRET)
.build();
credential.setAccessToken(accessToken);
//Create a new authorized API client
new Drive.Builder(httpTransport, jsonFactory, credential).build()
}
Here you have to pass the Access Token for accessing the Google Drive.
3. Then to Create a new Google Doc add this code.
xxxxxxxxxx
def createANewGoogleDocument(code: String, mimeType: String): List[String] = {
val service = prepareGoogleDrive(code)
val body = new File
body.setMimeType(mimeType)
val docType = mimeType.substring(mimeType.lastIndexOf(".") + 1)
body.setTitle("Untitled " + docType)
val file = service.files.insert(body).execute
List(file.getAlternateLink, file.getTitle(), file.getThumbnailLink())
}
Here you have to pass the Access Token (code) and the type (mimeType) of Google Doc which you want to create. The type of Google Doc can be Document/Spreadsheet/Presentation.
4. To Upload a file from your Hard Disk to Google Drive add this code.
xxxxxxxxxx
def uploadToGoogleDrive(accessToken: String, fileToUpload: java.io.File, fileName: String, contentType: String): String = {
val service = prepareGoogleDrive(accessToken)
//Insert a file
val body = new File
body.setTitle(fileName)
body.setDescription(fileName)
body.setMimeType(contentType)
val fileContent: java.io.File = fileToUpload
val mediaContent = new FileContent(contentType, fileContent)
//Inserting the files
val file = service.files.insert(body, mediaContent).execute()
file.getAlternateLink
}
Here you have to pass Access Token, file to upload, file name and content type which you want to upload to Google Drive
5. At last to Delete a Google Doc add this code.
xxxxxxxxxx
def deleteAGoogleDocument(code: String, docId: String) = {
val service = prepareGoogleDrive(code)
try {
service.files().delete(docId).execute();
} catch {
case ex: Exception => println(ex)
}
}
In here you to pass Access token and Doc Id in order to delete it from Google Drive. Document Id is present in Document Url. For Example, if your Google Doc URL is this https://docs.google.com/a/knoldus.com/document/d/1l2A2iNm5 _4uX6VZ9HVncaIRd-YXBZZ0FS0W-1U7UJ08/ then the document id is .
Note :- In case of Google Spreadsheet Doc Id is key. For example if the spreadsheet’s URL is this https: //docs.google.com/a/knoldus.com/spreadsheet/ccc?key=0AiZ5C9Yq7QMUdG84X3V1M1pNMlRvM0xnNldVSzRSa3c&usp=drive then doc Id is 0AiZ5C9Yq7QMUdG84X3V1M1pNMlRvM0xnNldVSzRSa3c. So, while deleting a spreadsheet from Google Docs pass this key as Doc Id to the function given above.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK