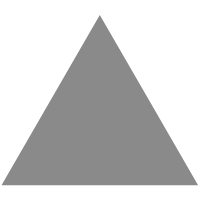
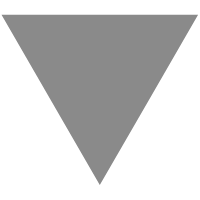
java数组转list
source link: https://studygolang.com/articles/32411
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
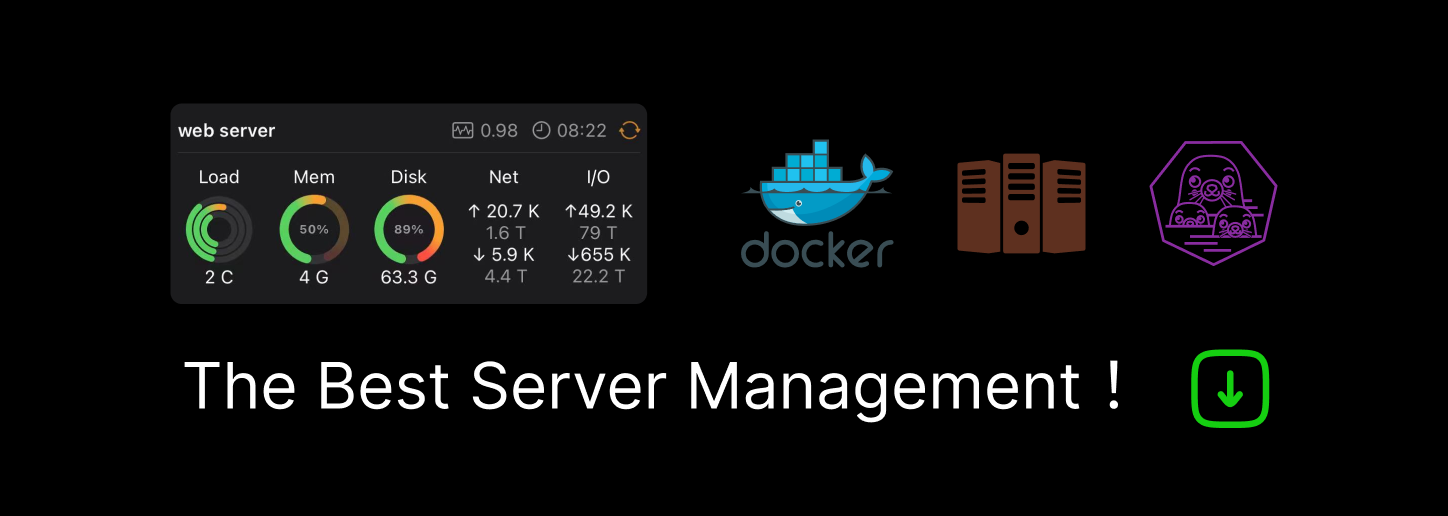
java中数组转list使用Arrays.asList(T... a)方法。
示例:
1.public class App { 2. public static void main(String[] args) { 3. List<String> stringA = Arrays.asList("hello", "world","A"); 4. String[] stringArray = {"hello","world","B"}; 5. List<String> stringB = Arrays.asList(stringArray); 6. 7. System.out.println(stringA); 8. System.out.println(stringB); 9. } 10.}
运行结果:
1.[hello, world, A] 2.[hello, world, B]
这个方法使用起来非常方便,简单易懂。但是需要注意以下两点。
一、不能把基本数据类型转化为列表
仔细观察可以发现asList接受的参数是一个泛型的变长参数,而基本数据类型是无法泛型化的,如下所示:
1.public class App { 2. public static void main(String[] args) { 3. int[] intarray = {1, 2, 3, 4, 5}; 4. //List<Integer> list = Arrays.asList(intarray); 编译通不过 5. List<int[]> list = Arrays.asList(intarray); 6. System.out.println(list); 7. } 8.}./*欢迎加入java交流Q君样:909038429一起吹水聊天 9. 10.output: 11.[[I@66d3c617]
这是因为把int类型的数组当参数了,所以转换后的列表就只包含一个int[]元素。
解决方案:
要想把基本数据类型的数组转化为其包装类型的list,可以使用guava类库的工具方法,示例如下:
1.int[] intArray = {1, 2, 3, 4}; 2.List<Integer> list = Ints.asList(intArray);
二、asList方法返回的是数组的一个视图
视图意味着,对这个list的操作都会反映在原数组上,而且这个list是定长的,不支持add、remove等改变长度的方法。
1.public class App { 2. public static void main(String[] args) { 3. int[] intArray = {1, 2, 3, 4}; 4. List<Integer> list = Ints.asList(intArray); 5. list.set(0, 100); 6. System.out.println(Arrays.toString(intArray)); 7. list.add(5); 8. list.remove(0); 9. 10. } 11.}
output:
1.[100, 2, 3, 4] 2.UnsupportedOperationException 3.UnsupportedOperationException
有疑问加站长微信联系(非本文作者)

Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK