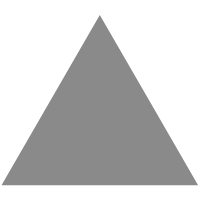
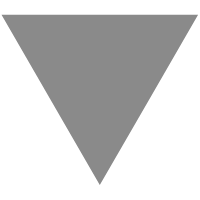
Java 14 – The good, the bad and the ugly – Learn. Write. Repeat.
source link: https://sergiuoltean.com/2020/04/09/java-14-the-good-the-bad-and-the-ugly/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
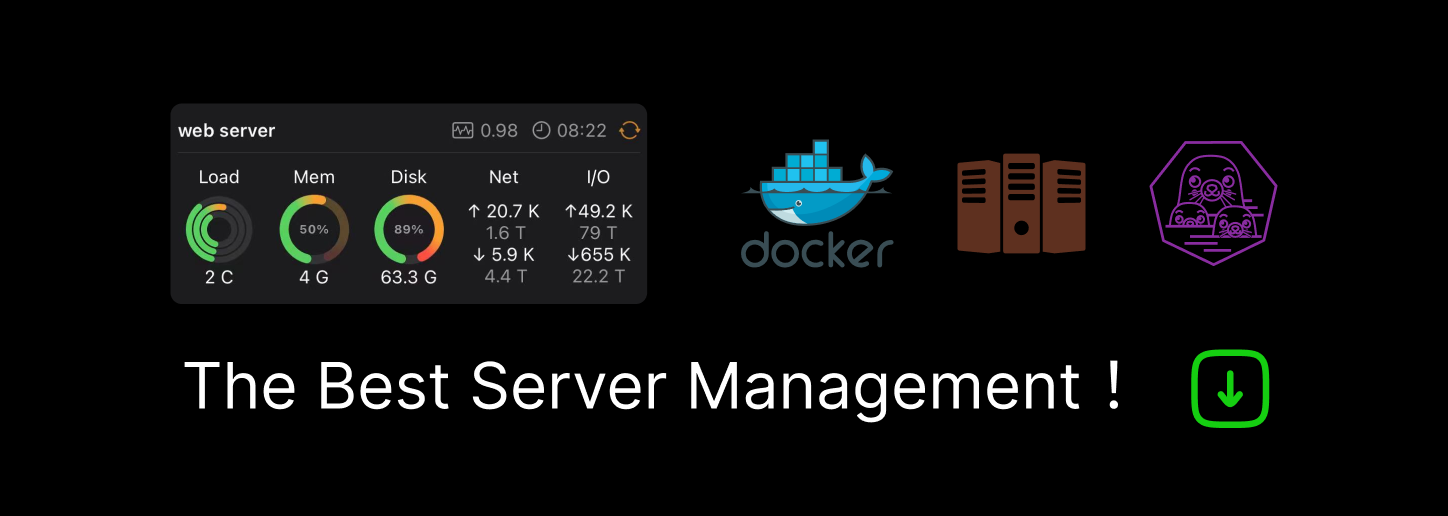
Another non LTS version which was released on 17th of march 2020. Let’s go through some of the features.
The Good
You see, in this world there’s two kinds of people, my friend: Those with loaded guns and those who dig. You dig.
Records
Well this was a long awaited feature. It’s still a preview, but it will become permanent as this will reduce the ceremony and this functionality is already present in many programming languages. This is nothing more than Lombok integrated into Java.
Lombok
@Value
public
class
User{
private
String username;
private
String password;
}
Java 14
record User(String username, String password) {}
They both generate at compile time code that allows us to interact with these.
Lombok will generate
public
final
class
User {
private
final
String username;
private
final
String password;
public
User(
final
String username,
final
String password) {
this
.username = username;
this
.password = password;
}
public
String getUsername() {
return
this
.username;
}
public
String getPassword() {
return
this
.password;
}
public
boolean
equals(
final
Object o) {
//ignored for brevity
}
public
int
hashCode() {
//ignored for brevity
}
public
String toString() {
//ignored for brevity
}
}
Java 14 will generate this
final
class
User
extends
java.lang.Record {
private
final
java.lang.String username;
private
final
java.lang.String password;
public
User(java.lang.String username, java.lang.String password) {
/* compiled code */
}
public
java.lang.String toString() {
/* compiled code */
}
public
final
int
hashCode() {
/* compiled code */
}
public
final
boolean
equals(java.lang.Object o) {
/* compiled code */
}
public
java.lang.String username() {
/* compiled code */
}
public
java.lang.String password() {
/* compiled code */
}
}
You see here many similarities.
Switch Expressions
The only thing that changed here is that they became permanent additions of the language after in Java 12 and 13 they were preview features.
Removal of the Concurrent Mark and Sweep GC
This was deprecated for some time now in Java 9 when the G1 was made the default GC. In this release it was removed entirely. Also now ZGC is also available on macOs and Windows, so we are not in shortage of GCs.
The bad
People with ropes around their necks don’t always hang. Even a filthy beggar like that has a protecting angel. A golden-haired angel watches over him.
Pattern matching for instanceOf
Every developer who respects himself will not use the instanceOf idiom since it’s not OOP. It breaks the Open/Close and Liskov from SOLID principles. If we take the context of polymorphism every time we would create a subtype we would have to add this instanceOf check to the newly created type. There are better patterns out there, but this post is not about this. Anyways if instanceOf is needed we can now move from
if
(obj
instanceof
String) {
String s = (String) obj;
// use s
}
which involves a check, a new variable and a cast to
if
(obj
instanceof
String s) {
// can use s here
}
just a check. The declaration and casting is happening on the same line.
Helpful NullPointerExceptions
I don’t know about this. I mean NPEs do not mean anything. And somehow we make that anything more helpful. That’s why we have Optional and NullObject pattern. Anyhow there are now more details in the stacktrace when we encounter a NPE.
Exception in thread
"main"
java.lang.NullPointerException:
Cannot read field
"c"
because
"a.b"
is
null
at Prog.main(Prog.java:
5
)
The ugly
If you want to shoot, just shoot don’t start talking
Text blocks (continued)
To allow finer control of the processing of newlines and white space, we introduce two new escape sequences.
What? We are not gonna start writing books in java!
\<line-terminator> escape sequence explicitly suppresses the insertion of a newline character.
String text =
""
"
There are
2
kinds of spurs my friend,\
those who come through the door (sign of the cross\
& those who come through the window!
""
";
There are 2 kinds of spurs my friend,those
who
come through the door (sign of the cross& those
who
come through the window!
\s
escape sequence simply translates to a single space. This is useful when we want our lines to have same number of characters. It also prevent the stripping of trailing white space.
String text =
""
"
There are
2
kinds of spurs my friend, \s
those who come through the door (sign of the cross\s
& those who come through the window! \s
""
";
There are 2 kinds of spurs my friend,
those
who
come through the door (sign of the cross
& those
who
come through the window!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK