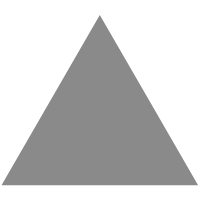
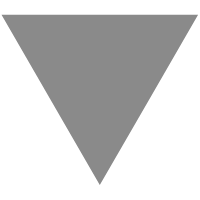
JSON Server to Mock API Servers: A Quick and Easy Solution
source link: https://keyholesoftware.com/2020/12/14/json-server-to-mock-api-servers-a-quick-and-easy-solution/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
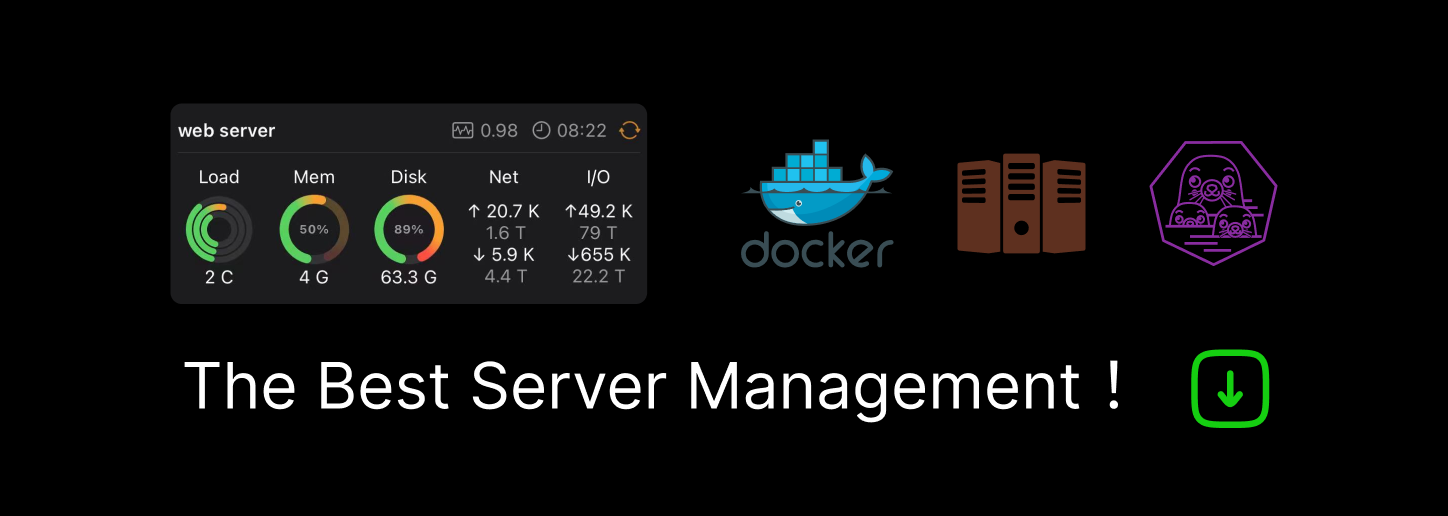
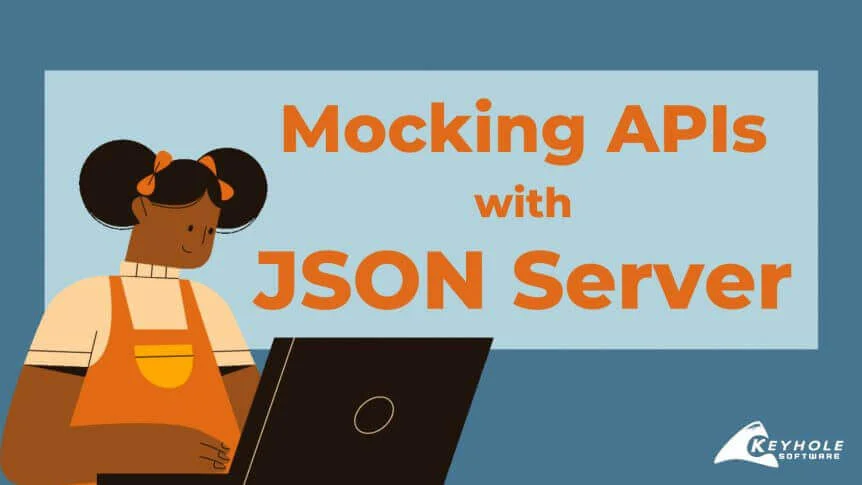
I have found myself in the following scenario many times throughout my career. Everyone is ready to get the project started. You know what you are going to be working on, and as the frontend developer, you get started right away. You get to the point that you are ready to test your code to see how the API calls work. There’s one major thing in your way, though. The APIs aren’t done yet.
You could just create some static JSON files and read them in, but that doesn’t really test out calling an API. That’s where JSON Server swoops in to save the day. In this post, I’ll walk through how to use JSON Server. We’ll set up our environment, serve a simple JSON file, and generate mock data.
Setting Up JSON Server
Let’s get started by making sure our development environment is set up. First, we’ll check that we have Node.js installed by opening up a terminal and entering node --version
.
If Node is installed, you will see the version number of what is installed. If you don’t see the version number go ahead and visit Node.js and follow the instructions to install.
Now that we have Node ready to go, let’s install JSON Server. In the terminal enter:
npm install -g json-server
JSON Server is now installed globally so we can use it for whatever project we would like.
With both of these installed, we now have everything we need to get started with a simple JSON Server that we can use to serve up data. So, let’s go ahead and create a place for our server to live.
In the terminal, navigate to where the project will reside. Then, create the new folder and initialize it.
mkdir mock-api-server cd mock-api-server npm init --yes
Serve Simple JSON File
Let’s start with just serving a simple JSON file that contains simple data for a customer. We will start with just the name of the customer in our data. Here is our data.json file:
{ "customers": [ {"id": 1, "name": {"first": "Leroy", "last": "Walton"}}, {"id": 2, "name": {"first": "Shane", "last": "Garrett"}}, {"id": 3, "name": {"first": "Sheryl", "last": "Robinson"}}, {"id": 4, "name": {"first": "Fred", "last": "Bush"}}, {"id": 5, "name": {"first": "Julie", "last": "Dawson"}}, {"id": 6, "name": {"first": "Ervin", "last": "Vega"}}, {"id": 7, "name": {"first": "Emanuel", "last": "Watson"}}, {"id": 8, "name": {"first": "Fred", "last": "Sharp"}}, {"id": 9, "name": {"first": "Virginia", "last": "Hall"}}, {"id": 10, "name": {"first": "Carrie", "last": "Sanchez"}} ], "addresses": [ { "id": 1, "address": "Belmont Avenue", "state": "KS" }, { "id": 2, "address": "10th Street", "state": "MO" }, { "id": 3, "address": "Route 100", "state": "IA" }, { "id": 4, "address": "Evergreen Lane", "state": "MN" }, { "id": 5, "address": "Main Street", "state": "WI" }, { "id": 6, "address": "Briarwood Drive", "state": "MN" }, { "id": 7, "address": "Linden Avenue", "state": "IA" }, { "id": 8, "address": "Eagle Road", "state": "MO" }, { "id": 9, "address": "Glenwood Avenue", "state": "KS" }, { "id": 10, "address": "Elm Street", "state": "WI" } ] }
Now that we have our data file, serving it up is as easy as the following line.
json-server --watch data.json
Once the server is started, navigate to http://localhost:3000/customers/3, and you should get:
{"id": 3, "name": {"first": "Sheryl", "last": "Robinson"}}
JSON Server supports the following request methods.
GET
POST
PUT
PATCH
DELETE
Using a POST
, PUT
, PATCH
, or DELETE
command will save the data from the request body to the data file.
If you’re using the POST
, PUT
, or PATCH
, make sure to include a Content-Type: application/json
header. If you forget the header, it will return a 2XX status code, but nothing will be written to your file.
Query Strings
JSON Server offers a variety of options for getting the data back that you need. Below are some query strings that can be used to get the results needed for your application.
I’ll only cover a few of the many query strings available for JSON Server. Check out this link to find more.
Filter
To query using deep properties of the data object, use:
http://localhost:3000/customers?name.first=Fred
Paginate
To get data for pagination use _page
and to set a limit on that data, use _limit
. 10 items are returned by default. The link header will include the first, prev, next, and last links.
http://localhost:3000/customers?_page=1&_limit=5 http://localhost:3000/customers?_page=3&_limit=3
To return the data sorted, use _sort
, and to set the order, use _order
. The default order is ascending order.
http://localhost:3000/customers?_sort=name.first http://localhost:3000/customers?_sort=name.last&_order=desc http://localhost:3000/addresses?_sort=state,address&_order=asc,desc
Slice
To get a subset of the data, use _start
and _end
or limit
. The response will also contain the X-Total-Count
header. Be aware that _start
is inclusive, _end
is exclusive, and that this is based on a zero index.
http://localhost:3000/addresses?_start=5&_end=9 http://localhost:3000/addresses?_start=2&_limit=5
Generated Mock Data
We now have a totally viable mock API server. If we want to just have the one static file for our data, we are all set.
However, another option would be to generate our mock data so we don’t have to come up with our own. Let’s use faker.js to help us generate the same set of mock data that we had written by hand.
Install faker
First things first, let’s get faker installed.
npm install faker
Create faker Code
In your favorite IDE, create an index.js
file in the root directory of our project.
In this file, we will put the following code. This code will automatically generate both our customers and addresses data sets that we previously had to create by hand.
Faker.js gives you the ability to generate fake data for addresses, companies, images, names, and more.
Now, let’s add the code into the index.js
file we created:
const faker = require('faker'); module.exports = () => { const data = { customers: [], addresses: [], } for (let i = 1; i <= 100; i++) { let customer = {}; let address = {}; customer.id = i; let name = {}; name.first = faker.name.firstName(); name.last = faker.name.lastName(); customer.name = name; data.customers.push(customer); address.id = i; address.address = faker.address.streetAddress(); address.state = faker.address.stateAbbr(); data.addresses.push(address); } return data; }
And that is all we need to get set up for a simple in-memory mock API server!
Serve Up the Data
One of the nice features of JSON Server is that it can be used as a static file server. So all we need to do to get our APIs live is to run the following code.
json-server index.js
With the server up and running, we should now have the following endpoints available to us.
http://localhost:3000/customers http://localhost:3000/addresses
Conclusion
What we discussed above just barely scratches the surface of what can be done with JSON Server. You have the ability to make something as simple as we have made or as extravagant as anything your imagination can come up with.
I personally like the option of being able to stand up my applications a little bit quicker. This gives me the ability to demonstrate what the application can accomplish without having to wait for the real data to be ready.
Happy mocking, and please leave any feedback or questions in the comments below!
Find the Full Repository
In case you want to take a look, I have made the entire project available in this GitHub Repository.
[Editor’s Note: Never miss a post by subscribing to receive new blogs by email. No spam, opt-out anytime.]Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK