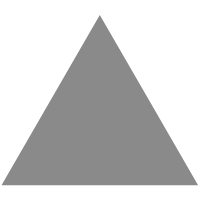
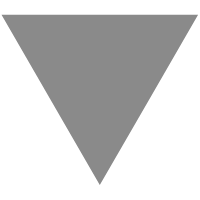
Getting Started with Chord PRO and Python
source link: https://datacrayon.com/posts/statistics/data-is-beautiful/getting-started-with-chordpro/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Preamble¶
from chord import Chord
This introduction to ChordPRO
was quickly put together to enable users to get started. You can see the most up-to-date API documentation at https://api.shahin.dev/docs.
Introduction¶
In a chord diagram (or radial network), entities are arranged radially as segments with their relationships visualised by arcs that connect them. The size of the segments illustrates the numerical proportions, whilst the size of the arc illustrates the significance of the relationships1.
Chord diagrams are useful when trying to convey relationships between different entities, and they can be beautiful and eye-catching.
Get Chord Pro¶
Click here to get lifetime access to the full-featured chord visualization API, producing beautiful interactive visualizations, e.g. those featured on the front page of Reddit.
- Produce beautiful interactive Chord diagrams.
- Customize colours and font-sizes.
- Access Divided mode, enabling two sides to your diagram.
- Symmetric and Asymmetric modes,
- Add images and text on hover,
- Access finer-customisations including HTML injection.
- Allows commercial use without open source requirement.
- Currently supports Python, JavaScript, and Rust, with many more to come (accepting requests).
The Chord Package¶
With Python in mind, there are many libraries available for creating Chord diagrams, such as Plotly, Bokeh, and a few that are lesser-known. However, I wanted to use the implementation from d3 because it can be customised to be highly interactive and to look beautiful.
I couldn't find anything that ticked all the boxes, so I made a wrapper around d3-chord myself. It took some time to get it working, but I wanted to hide away everything behind a single constructor and method call. The tricky part was enabling multiple chord diagrams on the same page, and then loading resources in a way that would support Jupyter Notebooks.
You can get the package either from PyPi using pip install chord
or from the GitHub repository. With your processed data, you should be able to plot something beautiful with just a single line, Chord(data, names).show()
License¶
To switch to the PRO version of the chord
package, you need to assign a valid username (the email you entered at purchase) and license key. This can be purchased here.
Chord.user = "your username" Chord.key = "your license key"
The chord package switches to PRO
mode when a username and license are specified. This enables the use of all the PRO
features.
This uses the Chord PRO
API service hosted on the DataCrayon.com (AWS hosted) server to generate your visualisation. Your parameter arguments (e.g. matrix, colors, etc) are sent to the API, which then generates and returns your HTML content.
matrix = [ [0, 2, 3, 1, 4, 1], [2, 0, 2, 1, 3, 2], [3, 2, 0, 1, 2, 2], [1, 1, 1, 0, 2, 2], [4, 3, 2, 2, 0, 1], [1, 2, 2, 2, 1, 0], ] names = ["Action", "Adventure", "Comedy", "Drama", "Fantasy", "Thriller"]
In basic version of chord
, matrix
and names
are the only sets of data that can be used to create a chord diagram. In the PRO version, you can also use details
and details_thumbs
. These enable the rich hover boxes.
details = [ [[], ["Movie 1","Movie 2"], ["Movie 3","Movie 4","Movie 5"], ["Movie 6","Movie 7"], ["Movie 8","Movie 9","Movie 10","Movie 11"], ["Movie 12"]], [["Movie 13","Movie 14"], [], ["Movie 15","Movie 16"], ["Movie 17"], ["Movie 18","Movie 19","Movie 20"], ["Movie 21","Movie 22"]], [["Movie 23","Movie 24","Movie 25"], ["Movie 26","Movie 27"], [], ["Movie 28"], ["Movie 29","Movie 30"], ["Movie 31","Movie 32"]], [["Movie 33"], ["Movie 34"], ["Movie 35"], [], ["Movie 36","Movie 37"], ["Movie 38","Movie 39"]], [["Movie 40","Movie 41","Movie 42","Movie 43"], ["Movie 44","Movie 45","Movie 46"], ["Movie 47","Movie 48"], ["Movie 49","Movie 50"], [], ["Movie 51"]], [["Movie 52"], ["Movie 53","Movie 54"], ["Movie 55","Movie 56"], ["Movie 57","Movie 58"], ["Movie 59"], []], ]
details_thumbs = [ [[], ["https://shahinrostami.com/images/stami-labs/lablet.png","https://shahinrostami.com/images/stami-labs/lablet.png"], ["https://shahinrostami.com/images/stami-labs/lablet.png","https://shahinrostami.com/images/stami-labs/lablet.png","https://shahinrostami.com/images/stami-labs/lablet.png"], ["https://shahinrostami.com/images/stami-labs/lablet.png","https://shahinrostami.com/images/stami-labs/lablet.png"], ["https://shahinrostami.com/images/stami-labs/lablet.png","https://shahinrostami.com/images/stami-labs/lablet.png","https://shahinrostami.com/images/stami-labs/lablet.png","https://shahinrostami.com/images/stami-labs/lablet.png"], ["https://shahinrostami.com/images/stami-labs/lablet.png"]], [["https://shahinrostami.com/images/stami-labs/lablet.png","https://shahinrostami.com/images/stami-labs/lablet.png"], [], ["https://shahinrostami.com/images/stami-labs/lablet.png","https://shahinrostami.com/images/stami-labs/lablet.png"], ["https://shahinrostami.com/images/stami-labs/lablet.png"], ["https://shahinrostami.com/images/stami-labs/lablet.png","https://shahinrostami.com/images/stami-labs/lablet.png","https://shahinrostami.com/images/stami-labs/lablet.png"], ["https://shahinrostami.com/images/stami-labs/lablet.png","https://shahinrostami.com/images/stami-labs/lablet.png"]], [["https://shahinrostami.com/images/stami-labs/lablet.png","https://shahinrostami.com/images/stami-labs/lablet.png","https://shahinrostami.com/images/stami-labs/lablet.png"], ["https://shahinrostami.com/images/stami-labs/lablet.png","https://shahinrostami.com/images/stami-labs/lablet.png"], [], ["https://shahinrostami.com/images/stami-labs/lablet.png"], ["https://shahinrostami.com/images/stami-labs/lablet.png","https://shahinrostami.com/images/stami-labs/lablet.png"], ["https://shahinrostami.com/images/stami-labs/lablet.png","https://shahinrostami.com/images/stami-labs/lablet.png"]], [["https://shahinrostami.com/images/stami-labs/lablet.png"], ["https://shahinrostami.com/images/stami-labs/lablet.png"], ["https://shahinrostami.com/images/stami-labs/lablet.png"], [], ["https://shahinrostami.com/images/stami-labs/lablet.png","https://shahinrostami.com/images/stami-labs/lablet.png"], ["https://shahinrostami.com/images/stami-labs/lablet.png","https://shahinrostami.com/images/stami-labs/lablet.png"]], [["https://shahinrostami.com/images/stami-labs/lablet.png","https://shahinrostami.com/images/stami-labs/lablet.png","https://shahinrostami.com/images/stami-labs/lablet.png","https://shahinrostami.com/images/stami-labs/lablet.png"], ["https://shahinrostami.com/images/stami-labs/lablet.png","https://shahinrostami.com/images/stami-labs/lablet.png","https://shahinrostami.com/images/stami-labs/lablet.png"], ["https://shahinrostami.com/images/stami-labs/lablet.png","https://shahinrostami.com/images/stami-labs/lablet.png"], ["https://shahinrostami.com/images/stami-labs/lablet.png","https://shahinrostami.com/images/stami-labs/lablet.png"], [], ["https://shahinrostami.com/images/stami-labs/lablet.png"]], [["https://shahinrostami.com/images/stami-labs/lablet.png"], ["https://shahinrostami.com/images/stami-labs/lablet.png","https://shahinrostami.com/images/stami-labs/lablet.png"], ["https://shahinrostami.com/images/stami-labs/lablet.png","https://shahinrostami.com/images/stami-labs/lablet.png"], ["https://shahinrostami.com/images/stami-labs/lablet.png","https://shahinrostami.com/images/stami-labs/lablet.png"], ["https://shahinrostami.com/images/stami-labs/lablet.png"], []], ]
Let's see what the Chord()
defaults produce when we invoke the show()
method.
Chord(matrix, names, details=details, details_thumbs=details_thumbs).show()
Chord Diagram
Chord Diagrams with Two Sides¶
matrix = [ [0, 0, 0, 1, 4, 1], [0, 0, 0, 1, 3, 2], [0, 0, 0, 1, 2, 2], [1, 1, 1, 0, 0, 0], [4, 3, 2, 0, 0, 0], [1, 2, 2, 0, 0, 0], ] names = ["A", "B", "C", "1", "2", "3"] hex_colours = ["#7400B8", "#5E60CE", "#5684D6", "#56CFE1", "#64DFDF", "#80FFDB"] Chord(matrix, names, title="A chord diagram with two sides.", colors=hex_colours, divide=True, divide_idx=3).show()
Chord Diagram
Optional Customisations¶
Chord PRO gives you finer control over the look and interactive components of the diagram. The parameters and their defaults include the following:
colors="d3.schemeSet1", opacity=0.8, padding=0.01, width=700, label_color="#454545", wrap_labels=True, margin=0, credit=False, font_size="16px", font_size_large="20px", details=[], details_thumbs=[], thumbs_width=85, thumbs_margin=5, thumbs_font_size=14, popup_width=350, noun="instances", details_separator=", ", divide=False, divide_idx=0, divide_size=0.5, verb="occur together in", symmetric=True, title="", arc_numbers=False, divide_left_label = "", divide_right_label = "", inner_radius_scale = 0.39, outer_radius_scale = 1.1,
You can see the most up-to-date API documentation at https://api.shahin.dev/docs.
Let's see a few of these in action.
matrix = [ [0, 0, 0, 1, 4, 1], [0, 0, 0, 1, 3, 2], [0, 0, 0, 1, 2, 2], [1, 1, 1, 0, 0, 0], [4, 3, 2, 0, 0, 0], [1, 2, 2, 0, 0, 0], ] names = ["A", "B", "C", "1", "2", "3"] hex_colours = ["#ffadad", "#ffd6a5", "#fdffb6", "#caffbf", "#9bf6ff", "#a0c4ff"] Chord(matrix, names, title="A chord diagram with customisations.", colors=hex_colours, divide=True, divide_idx=3, divide_size = 0.9, opacity=0.4, padding=0.01, outer_radius_scale=1.2, inner_radius_scale=0.35, divide_left_label="Numbers", divide_right_label="Letters", verb="do something together", noun="things", allow_download=True).show()
Conclusion¶
In this section, we've introduced major PRO features of the chord
package. We used the package and some synthetic data to demonstrate several chord diagram visualisations with different configurations. The chord Python package is available for free using pip install chord
.
-
Tintarev, N., Rostami, S., & Smyth, B. (2018, April). Knowing the unknown: visualising consumption blind-spots in recommender systems. In Proceedings of the 33rd Annual ACM Symposium on Applied Computing (pp. 1396-1399). ↩
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK