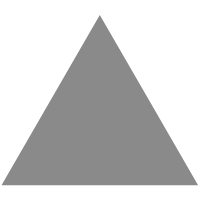
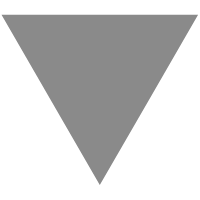
ASP.NET Core 中间件的使用(二):依赖注入的使用
source link: https://www.cnblogs.com/xiongze520/p/14155858.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
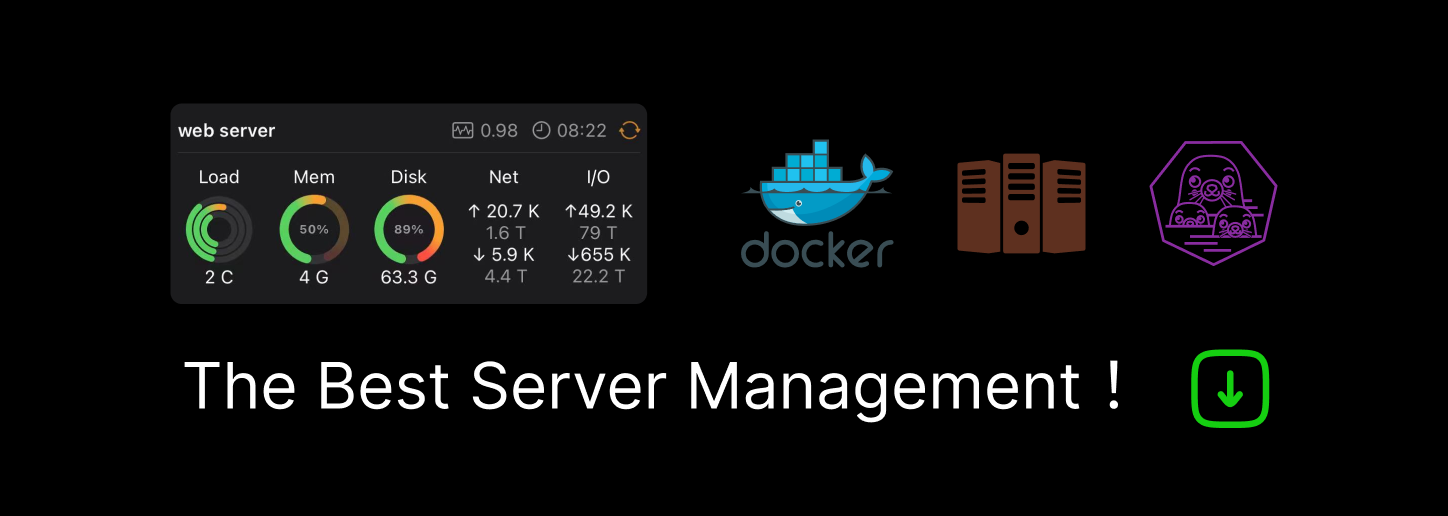
写在前面
上一篇大家已经粗略接触了解到.NET Core中间件的使用:ASP .Net Core 中间件的使用(一):搭建静态文件服务器/访问指定文件,
.NET Core框架中很多核心对象都是通过依赖注入的方式提供的,那什么是依赖注入?
这也是个老生常谈的问题,到底依赖注入是什么? 为什么要用它? 初学者特别容易对控制反转IOC(Iversion of Control),DI等概念搞晕。
什么是依赖注入?
提到依赖注入,大家一定会想到控制反转,怎么了解,控制反转是一种设计原则(Inversion of Control,缩写为IoC),而依赖注入((Dependency Injection,简称DI))是它的一种实现方式。
当一个类需要另一个类协作来完成工作的时候就产生了依赖。比如我们在AccountController这个控制器需要完成和用户相关的注册、登录 等事情。
这里有一个设计原则:依赖于抽象,而不是具体的实现,当一个类依赖于具体依赖时,它被认为与该类紧密耦合。
依赖注入的目的是为了什么?
控制反转用于解耦,将接口和实现的耦合降低,有一个好处就是,一个接口,可以进行不同的实现,这样提高扩展性,确保代码的可维护性和扩展性。
通俗的讲,就是对象在被使用前,我们需要New一下对象,创建一个实例对象,然后在进行其他操作。
怎么使用依赖注入?
.NET Core 自带了依赖注入的框架,我们可以归纳为这几个使用方式,当然还有很多使用方法(常规,泛型,工厂),就不一一举例了:
-
构造函数注入;
-
方法注入;
-
属性注入;
这么归纳是不是感觉不太好理解?没关系,我们进一步细化为如下使用方式:
-
在Startup类型的构造函数中注入;
-
在Startup类型的Configure方法中注入;
-
在中间件类型构造函数中注入;
-
在中间件类型的Invoke/InvokeAsync方法中注入;
-
在Controller类型的构造函数中注入;
-
在Controller的Action方法中注入;
一、在Startup类型的构造函数中注入
配置的IConfiguration对象和表示承载环境的IHostEnvironment对象可以直接注入Startup构造函数中。
当然也可以通过注入IWebHostEnvironment对象的方式得到当前承载环境相关的信息,
这是因为ASP.NET Core应用中的承载环境通过IWebHostEnvironment接口表示,IWebHostEnvironment接口派生于IHostEnvironment接口)。
我们可以通过一个简单的实例来验证针对Startup的构造函数注入。
如下面的代码片段所示,我们在调用IWebHostBuilder接口的Startup<TStartup>方法时注册了自定义的Startup类型。
在定义Startup类型时,我们在其构造函数中注入上述3个对象,提供的调试断言不仅证明了3个对象不为Null,还表明采用IHostEnvironment接口和IWebHostEnvironment接口得到的其实是同一个实例。
class Program { static void Main() { Host.CreateDefaultBuilder().ConfigureWebHostDefaults(builder => builder.UseStartup<Startup>()) .Build() .Run(); } } public class Startup { public Startup(IConfiguration configuration, IHostEnvironment hostingEnvironment,IWebHostEnvironment webHostEnvironment) { Debug.Assert(configuration != null); Debug.Assert(hostingEnvironment != null); Debug.Assert(webHostEnvironment != null); Debug.Assert(ReferenceEquals(hostingEnvironment, webHostEnvironment)); } public void Configure(IApplicationBuilder app) { } }
二、在Startup类型的Configure方法中注入
这种注入方式也叫管道注入,这种是使用比较多的一种注入方式,因为.NET Core创建项目的时候已经在Startup.cs类里面生成框架了(管道注入),
如果构造函数注入还可以对注入的服务有所选择,那么对于Configure方法来说,通过任意方式注册的服务都可以注入其中,包括通过调用
IHostBuilder、IWebHostBuilder和Startup自身的ConfigureServices方法注册的服务,还包括框架自行注册的所有服务。
如下面的代码代码片段所示,我们分别调用IWebHostBuilder和Startup的ConfigureServices方法注册了针对IStudent接口和ISchool接口的服务,这两个服务直接注入Startup的Configure方法中。另外,Configure方法要求提供一个用来注册中间件的IApplicationBuilder对象作为参数,但是对该参数出现的位置并未做任何限制。
class Program { static void Main() { Host.CreateDefaultBuilder().ConfigureWebHostDefaults(builder => builder .UseStartup<Startup>() .ConfigureServices(svcs => svcs.AddSingleton<IStudent, Student>())) .Build() .Run(); } } public class Startup { public void ConfigureServices(IServiceCollection services) => services.AddSingleton<ISchool, School>(); public void Configure(IApplicationBuilder app, IStudent student, ISchool school) { Debug.Assert(student != null); Debug.Assert(school!= null); } }
三、在中间件类型构造函数中注入
ASP.NET Core请求处理管道最重要的对象是用来真正处理请求的中间件。
由于ASP.NET Core在创建中间件对象并利用它们构建整个请求处理管道时,所有的服务都已经注册完毕,所以任何一个注册的服务都可以注入中间件类型的构造函数中。
如下所示的代码片段体现了针对中间件类型的构造函数注入。
class Program { static void Main() { Host.CreateDefaultBuilder().ConfigureWebHostDefaults(builder => builder .ConfigureServices(svcs => svcs .AddSingleton<studentschoolMiddleware>() .AddSingleton<IStudent, student>() .AddSingleton<ISchool, school>()) .Configure(app => app.UseMiddleware<studentschoolMiddleware>())) .Build() .Run(); } } public class studentschoolMiddleware : IMiddleware { public studentschoolMiddleware(IStudent student, ISchool school) { Debug.Assert(student != null); Debug.Assert(school != null); } public Task InvokeAsync(HttpContext context, RequestDelegate next) { Debug.Assert(next != null); return Task.CompletedTask; } }
四、在中间件类型的Invoke/InvokeAsync方法中注入
如果采用基于约定的中间件类型定义方式,注册的服务还可以直接注入真正用于处理请求的InvokeAsync方法或者Invoke方法中。
另外,将方法命名为InvokeAsync更符合TAP(Task-based Asynchronous Pattern)编程模式,之所以保留Invoke方法命名,主要是出于版本兼容的目的。
如下所示的代码片段展示了针对InvokeAsync方法的服务注入。
class Program { static void Main() { Host.CreateDefaultBuilder().ConfigureWebHostDefaults(builder => builder .ConfigureServices(svcs => svcs .AddSingleton<IStudent, student>() .AddSingleton<ISchool, school>()) .Configure(app => app.UseMiddleware<studentschoolMiddleware>())) .Build() .Run(); } } public class studentschoolMiddleware { private readonly RequestDelegate _next; public studentschoolMiddleware(RequestDelegate next) => _next = next; public Task InvokeAsync(HttpContext context, IStudent student, ISchool school) { Debug.Assert(context != null); Debug.Assert(student != null); Debug.Assert(school != null); return _next(context); } }
对于基于约定的中间件,构造函数注入与方法注入存在一个本质区别。
由于中间件被注册为一个Singleton对象,所以我们不应该在它的构造函数中注入Scoped服务。
Scoped服务只能注入中间件类型的InvokeAsync方法中,因为依赖服务是在针对当前请求的服务范围中提供的,所以能够确保Scoped服务在当前请求处理结束之后被释放。
五、在Controller类型的构造函数中注入
在一个ASP.NET Core MVC应用中,我们可以在定义的Controller中以构造函数注入的方式注入所需的服务。
在如下所示的代码片段中,我们将IStudentschool服务注入到HomeController的构造函数中。
class Program { static void Main() { Host.CreateDefaultBuilder().ConfigureWebHostDefaults(builder => builder .ConfigureServices(svcs => svcs .AddSingleton<IStudentschool, studentschool>() .AddSingleton<ISchool, school>() .AddControllersWithViews()) .Configure(app => app .UseRouting() .UseEndpoints(endpoints => endpoints.MapControllers()))) .Build() .Run(); } } public class HomeController : Controller { public HomeController(IStudentschool studentschool) => Debug.Assert(studentschool!= null); }
六、在Controller的Action方法中注入
借助于ASP.NET Core MVC基于模型绑定的参数绑定机制,我们可以将注册的服务绑定到目标Action方法的参数上,进而实现针对Action方法的依赖注入。
在采用这种类型的注入方式时,我们需要在注入参数上按照如下的方式标注FromServicesAttribute特性,用以确定参数绑定的来源是注册的服务。
在如下所示的代码片段
class Program { static void Main() { Host.CreateDefaultBuilder().ConfigureWebHostDefaults(builder => builder .ConfigureServices(svcs => svcs .AddSingleton<IStudentschool, Studentschool>() .AddControllersWithViews()) .Configure(app => app .UseRouting() .UseEndpoints(endpoints => endpoints.MapControllers()))) .Build() .Run(); } } public class HomeController: Controller { [HttpGet("/")] public void Index([FromServices]IStudentschool studentschool) { Debug.Assert(Studentschool!= null); } }
七、在视图中注入
在ASP.NET Core MVC应用中,我们还可以将服务注册到现的View中。
假设我们定义了如下这个简单的MVC程序,并定义了一个简单的HomeController。
如下代码片段
class Program { static void Main() { Host.CreateDefaultBuilder().ConfigureWebHostDefaults(builder => builder .ConfigureServices(svcs => svcs .AddSingleton<IStudentschool, Studentschool>() .AddControllersWithViews()) .Configure(app => app .UseRouting() .UseEndpoints(endpoints => endpoints.MapControllers()))) .Build() .Run(); } } public class HomeController: Controller { [HttpGet("/")] public IActionResult Index() => View(); }
我们为HomeController定义了一个路由指向根路径(“/”)的Action方法Index,该方法在调用View方法呈现默认的View之前,
将注入的IStudentschool服务以ViewBag的形式传递到View中。
如下所示的代码片段是这个Action方法对应View(/Views/Home/Index.cshtml)的定义,我们通过@inject指令注入了IStudentschool服务,并
将属性名设置为Studentschool,这意味着当前View对象将添加一个Studentschool属性来引用注入的服务。
@inject IStudentschool Studentschool @ { Debug.Assert(Studentschool!= null); }
写在后面
到这里就简单介绍了.NET Core依赖注入的使用方式,更多的使用方式还有待探索,一些使用过程当中的注意事项也需要探索,如:
- 有效地设计服务及其依赖关系;
- 防止多线程问题;
- 防止内存泄漏;
- 防止潜在的错误;
- 如果使用了服务注入,还要考虑服务生命周期(服务不能依赖于生命周期小于其自身的服务。);
参考: https://www.cnblogs.com/artech/p/di-in-asp-net-core-3.html
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK