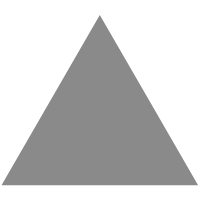
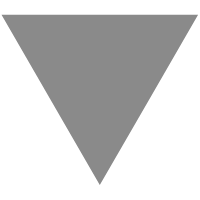
Reasons to Learn React
source link: https://www.pluralsight.com/blog/software-development/reasons-to-learn-react
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
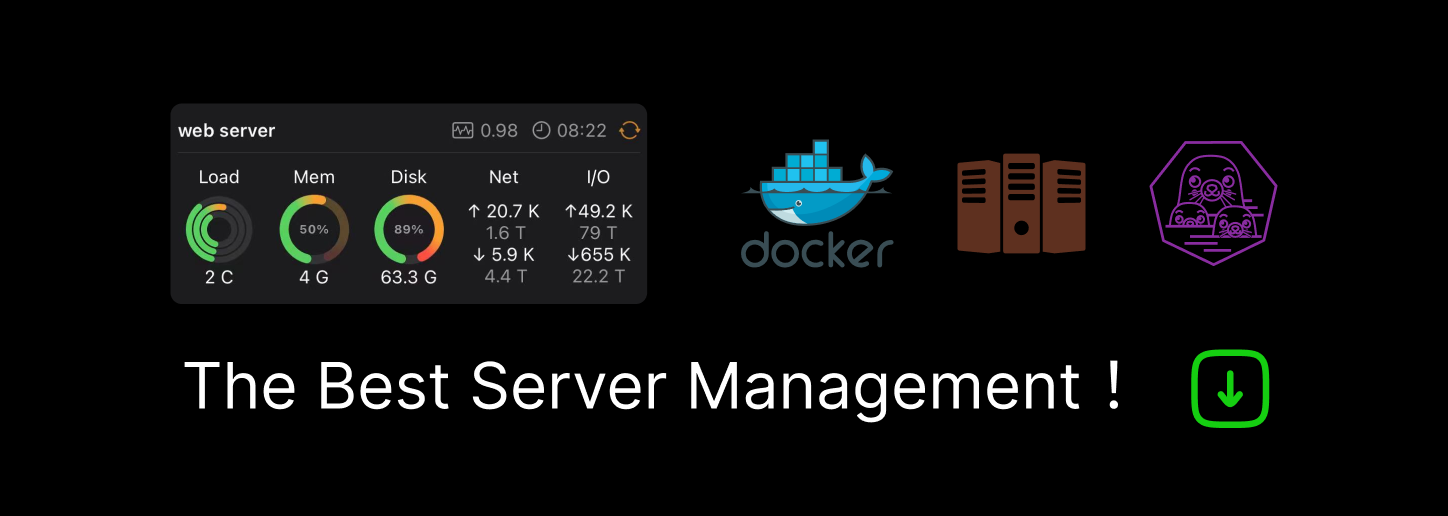
According to the latest StackOverflow developer survey (1), React is the most popular SPA Framework out there. There are three simple reason for this.
1. It’s Easy to develop with
2. It Leverages JavaScript skills
3. It Provides a great browser experience for users
Complexity is the Enemy of Productivity
Every new programming technology we as developers learn add to our accumulated knowledge we know from other related and similar technologies. For example, when we want to write some code that, in the browser, displays a counter that increments, or decrements based on button clicks, we draw on the knowledge of how we’ve done that before in other technologies.
You should be thinking, how hard can making an increment and decrement button be? You’d be surprised. Without any framework, you need to worry about how you update different browsers, how you store changing data, and how to integrate multiple html and JavaScript files to work together. With most other frameworks, you need to learn complex build systems and code generators before you even begin to write your own code.
React is different. You create simple JavaScript code that simply returns, using a special JavaScript syntax called JSX. That JSX allows us to write HTML directly in JavaScript. That is, say all you want is to have your React App display “Hello from Pluralsight at <Current Time of Day>” in bold letters. You only need to create the following simple JavaScript.
export default () => (
<b>Hello from Pluralsight {new Date().toLocaleTimeString()}</b>
);
React Built Using State from The Beginning
Unlike other JavaScript libraries, The Facebook React team built application state into React from the start. You can think of state as referring to what’s the value of your JavaScript variables at any given time. That current state will determine what is ultimately rendered to the uses browser.
In our above example, the calculated time string is the state of the page when it renders.
Let’s expand our simple app by explicitly using what we call the useState React Hook. Let’s use that hook to create a count state and a method setCount, which will update count state. Let’s also create two buttons in our JSX rendering, one that increments count and one that decrements it.
import { useState } from "react";
export default () => {
const [count, setCount] = useState(0);
return (
<div>
<h2>Hello from Pluralsight. Count Value: {count}</h2>
<button
onClick={(event) => {
setCount(count + 1);
}}
>
Increment
</button>
<button
onClick={(event) => {
setCount(count - 1);
}}
>
Decrement
</button>
</div>
);
};
The above JavaScript actually has very few changes from our previous example. First, we’ve added an import for useState from the React library. Then, we’ve converted the ECMAScript shorthand syntax for our function to the statement syntax, meaning we have a return statement and curly braces. We’ve updated our output to include count instead of the current time, and finally, we’ve added an increment and a decrement buttons with click handlers.
When this simple React app is run, the buttons change the state of the app, by increment the counter, and because of that state change, React knows to update just the parts of the DOM that need it.

You may be wondering why the name “React”. Fundamentally, it’s because apps we build with it are 100% declarative meaning that we make declarations like “increment the counter by 1”, then the rendered output from the app just reacts to the change. In other words, React apps just react to state changes. Simple as that.
The Browser Experience the User Gets is Awesome
What really matters is how well our apps we build with React run in our customers browsers. It doesn’t matter how easy they are to develop, but if they don’t run well for our customers, it just doesn’t matter.
This is where React is really awesome. Because React is the programming library behind Facebook’s website, it has to be extremely optimized and performant. In both regards it does not disappoint. Saying “React just works” is an understatement. Inside React the are components like the reconciler, optimizer and scheduler that make it so fast, but as developer, all we care about is it just works fast and reliably.
The React team is so focused on making the React library fast and stable, that in the latest release, version 17, there were literally no features added. The only change after updating to this latest version is apps bundle to much smaller size, they run significantly faster, and are more stable. That’s really something for the Facebook React team to be proud of.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK