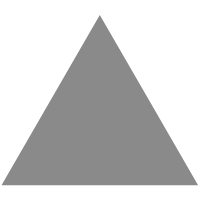
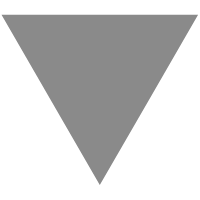
Simple Class to Make XUL StatusBarPanel Icon Blink from FireFox extension
source link: http://www.mikechambers.com/blog/2004/12/01/simple-class-to-make-xul-statusbarpanel-icon-blink-from-firefox-extension/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
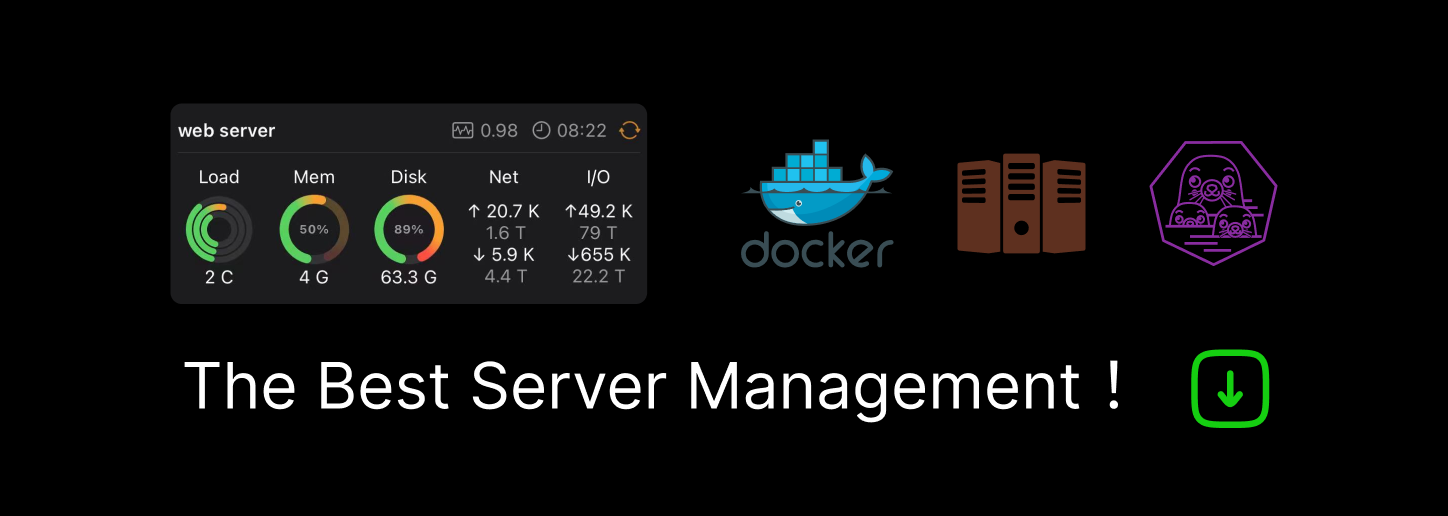
Simple Class to Make XUL StatusBarPanel Icon Blink from FireFox extension
Wednesday, December 1, 2004
I have put together a simple class that will make the icon in a XUL StatusBarPanel tag within a FireFox extension blink.
In order for this to work, the StatusBarPanel tag must have its class set to statusbarpanel-menu-iconic.
For example, here is the XUL tag:
<statusbarpanel class="statusbarpanel-menu-iconic" id="my-status" />
and the associated style sheet:
statusbarpanel#my-status[status="on"]
{
list-style-image: url("chrome://appname/skin/on_status.png");
}
statusbarpanel#my-status[status="off"]
{
list-style-image: url("chrome://appname/skin/off_status.png");
}
And finally, here is the code to use the class and make the icon blink:
var menuStatusBar = document.getElementById("my-status");
var blinker = new StatusBarPanelBlinker();
blinker.setStatusBarPanel(menuStatusBar);
blinker.setOnStatus("on");
blinker.setOffStatus("off");
blinker.startBlink();
Note that I am still getting familiar with XUL and the JavaScript API, so there may be a way to make this more generic to work with other tags. If I figure it out, I will update the class.
Here is the class:
/*
StatusBarPanelBliner Class
Created by Mike Chambers
http://www.mikechambers.com
Makes the icon of an XUL statusbarpanel instance blink.
Usage:
var blinker = new StatusBarPanelBlinker();
blinker.setStatusBarPanel(menuStatusBar);
blinker.setOnStatus("on");
blinker.setOffStatus("off");
blinker.startBlink();
The StatusBarPanel tag must have its class set to "statusbarpanel-menu-iconic"
For example, here is a tag:
and the associated style sheet:
statusbarpanel#my-status[status="on"]
{
list-style-image: url("chrome://appname/skin/on_status.png");
}
statusbarpanel#my-status[status="off"]
{
list-style-image: url("chrome://appname/skin/off_status.png");
}
*/
/***** Constructor *****/
function StatusBarPanelBlinker()
{
}
/***** Properties *****/
StatusBarPanelBlinker.prototype.onStatus = undefined; //String
StatusBarPanelBlinker.prototype.offStatus = ""; //String
StatusBarPanelBlinker.prototype.interval = 750; //Number
StatusBarPanelBlinker.prototype.statusBarPanel = undefined; //StatusBarPanel
StatusBarPanelBlinker.prototype.intervalId = undefined; //Number
/***** Methods *****/
//starts the blinking of the icon
StatusBarPanelBlinker.prototype.startBlink = function()
{
this.intervalId = setInterval(this.doBlink, this.interval, this);
}
//stops the icon from blinking
StatusBarPanelBlinker.prototype.stopBlink = function()
{
clearInterval(this.intervalId);
this.intervalId = undefined;
this.statusBarPanel.setAttribute("status", this.onStatus);
}
//private internal function that toggles the state of the icon
StatusBarPanelBlinker.prototype.doBlink = function(scope)
{
if(scope == undefined)
{
scope = this;
}
var newStatus = (scope.statusBarPanel.getAttribute("status") == scope.onStatus)?
scope.offStatus: scope.onStatus;
scope.statusBarPanel.setAttribute("status", newStatus);
}
//returns whether the icon is currently blinking, i.e. startBlink() has been called
StatusBarPanelBlinker.prototype.isBlinking = function()
{
return !(this.intervalId == undefined);
}
/***** Getter / Setters *****/
//set the statusbarpanel instance whose icon we will blink
StatusBarPanelBlinker.prototype.setStatusBarPanel = function(statusBarPanel)
{
this.statusBarPanel = statusBarPanel;
}
//return the statusbarpanel instance that the class is making blink
StatusBarPanelBlinker.prototype.getStatusBarPanel = function()
{
return this.statusBarPanel;
}
//interval between blink states in milliseconds. This is basically how fast
//the icon blinks
StatusBarPanelBlinker.prototype.setBlinkInterval = function(interval)
{
this.interval = interval;
}
//returns the blink interval
StatusBarPanelBlinker.prototype.getBlinkInterval = function()
{
return this.interval;
}
//the value of the statusbarpanel's status attribute that displays the icon
StatusBarPanelBlinker.prototype.setOnStatus = function(onStatus)
{
this.onStatus = onStatus;
}
//return's on icon status for statusbarpanel
StatusBarPanelBlinker.prototype.getOnStatus = function()
{
return this.onStatus;
}
//the value of the statusbarpanel's status attribute that displays the off
//status of the blink / icon
StatusBarPanelBlinker.prototype.setOffStatus = function(offStatus)
{
this.offStatus = offStatus;
}
//return's off icon status for statusbarpanel
StatusBarPanelBlinker.prototype.getOffStatus = function()
{
return this.offStatus;
}
Post any comments, questions or suggestions in the comments section.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK