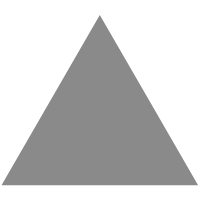
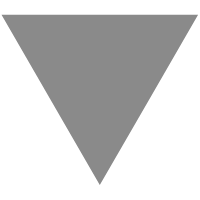
Some language features really make me worry
source link: http://rachelbythebay.com/w/2012/12/24/yield/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Some language features really make me worry
Hacker News is a pretty solid source for a bunch of crazy new things coming down the pipe. I saw one such post about two weeks ago about a new feature in Javascript: "Coroutine Event Loops". I spotted that and just got a really bad feeling about where that could go.
The example given shows an ordinary JS function which assigns values to variables from a new keyword called "yield", like this:
var x = yield;
When it gets there, it effectively remembers where it was and then returns to the caller. This is where it gets weird. Later on, you can call back to that same function to pass in a value which will then resume execution at that same point. Assuming "f" is the function, it looks like this:
f.send(123);
Back in the function, that "123" lands in "x", and execution continues from that spot. It keeps running like usual until it hits a return, the end of the function, or another yield.
This seems like a spectacular way to twist your brain completely out of shape and really confuse people who look at your code later. It basically means that multiple calls to the same function with the same arguments could do completely different things.
Granted, right now, you can already make a function which has (seemingly) nondeterministic behavior. Imagine a function like this:
function f(a, b) { switch (a) { case 1: do_first(b); break; case 2: do_second(b); break; case 3: do_third(b); break; } }
In that case, your function calls would at least look different since that first argument would change.
f(1, aaa); f(2, bbb); f(3, ccc);
Now imagine rewriting that function so it somehow kept track of how many times it had been called. You could remove that first argument, and now your calls would look like this:
f(aaa); f(bbb); f(ccc);
It's still doing different things with those arguments, but you can't tell that just by looking at the calls. I would hope this would come across as a bad thing to most people, but it's exactly what this "yield" behavior lets you do. It turns into calls like this:
f.send(aaa); f.send(bbb); f.send(ccc);
As far as I'm concerned, these last two examples are just as opaque and have the ability to be quite annoying for maintenance programmers down the road. You really have no idea what's going on in there.
Maybe I'm missing something, but this looks like a language feature which might just create more drama than it solves.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK