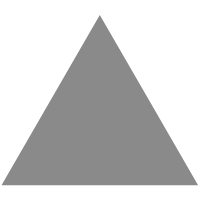
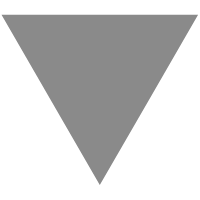
Introducing C# 9: Extending Partial Methods
source link: https://anthonygiretti.com/2020/08/10/introducing-c-9-extending-partial-methods/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
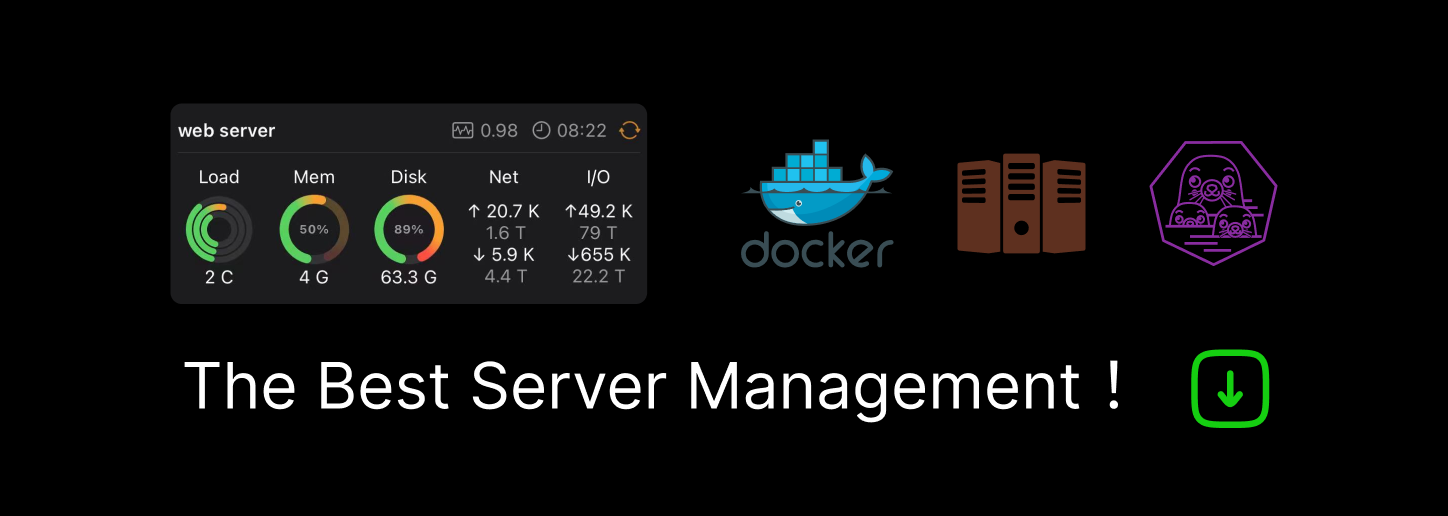

Introducing C# 9: Extending Partial Methods
Introduction
C# 8 (and above) has some restrictions regarding partial methods. For example :
- Partial methods must have a void return type
- Partial methods can’t have out parameters
- Partial methods can’t have any accessibility keyword (public, private, protected etc….)
C# 9 aims to remove these restrictions. If you want to learn more about the motivation behind this, you can find a good description on the Github page here: https://github.com/jaredpar/csharplang/blob/partial/proposals/extending-partial-methods.md
Behavior before C# 9
Below are some examples of what happens when a partial methods has or not an accessibility keyword, has or not an out parameter, a void or not return type, implements an interface:
partial class MyService : IMyService { partial void MyFirstFunction(); // Ok private partial void MySecondFunction(); // CS0750 A partial method cannot have access modifiers or the virtual, abstract, override, new, sealed, or extern modifiers private partial void MyThirdFunction(); // CS0750 A partial method cannot have access modifiers or the virtual, abstract, override, new, sealed, or extern modifiers private partial object MyFourthFunction(); // CS0750 A partial method cannot have access modifiers or the virtual, abstract, override, new, sealed, or extern modifiers + CS0766 Partial methods must have a void return type private partial object MyFifthFunction(); // CS0750 A partial method cannot have access modifiers or the virtual, abstract, override, new, sealed, or extern modifiers + CS0766 Partial methods must have a void return type private partial void MySixthFunction(out int result); // CS0750 A partial method cannot have access modifiers or the virtual, abstract, override, new, sealed, or extern modifiers + CS0752 A partial method cannot have out parameters public partial void MySeventhFunction(); // CS0750 A partial method cannot have access modifiers or the virtual, abstract, override, new, sealed, or extern modifiers }
partial class MyService { private partial void MyThirdFunction() { } // CS0750 A partial method cannot have access modifiers or the virtual, abstract, override, new, sealed, or extern modifiers private partial object MyFifthFunction() { return new { }; } // CS0750 A partial method cannot have access modifiers or the virtual, abstract, override, new, sealed, or extern modifiers + CS0766 Partial methods must have a void return type }
public interface IMyService { void MySeventhFunction(); }
Note for interface implementation: because C# 8 and above doesn’t support accessibility keyword on partial methods it’s impossible to implement partial method from its interface signature, because without any keyword, the method is implicitly a private method, which doesn’t allow to implement this method from its interface signature.
Behavior with C# 9
Now let’s take the exact same partial class definition above and see what’s the compiler behavior now:
partial class MyService : IMyService { partial void MyFirstFunction(); // Ok private partial void MySecondFunction(); // CS8795 Partial method must have an implementation part because it has accessibility modifiers private partial void MyThirdFunction(); // Ok private partial object MyFourthFunction(); // CS8795 Partial method must have an implementation part because it has accessibility modifiers private partial object MyFifthFunction(); // Ok private partial void MySixthFunction(out int result); // CS8795 Partial method must have an implementation part because it has accessibility modifiers public partial void MySeventhFunction(); // Ok }
partial class MyService { private partial void MyThirdFunction() { } // Ok private partial object MyFifthFunction() { return new { }; } // Ok public partial void MySeventhFunction() { } // Ok }
public interface IMyService { void MySeventhFunction(); }
C# 9 allows now what was missing in C# 8 and above, but, it requires now an implementation on methods that are defined with:
- void or notreturn type
- out parameters
- accessibility keyword (public, private, protected etc….)
As you can see above C# 9 brings a new error code with its message if the previous conditions are not fulfilled:
CS8795: Partial method must have an implementation part because it has accessibility modifiers
I’m really excited by what’s coming with C# 9, and you ?
Like this:
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK