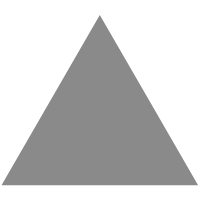
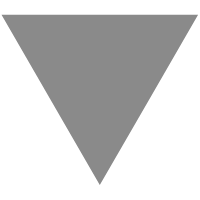
使用SharpZipLib实现zip压缩
source link: https://www.cnblogs.com/tuyile006/archive/2008/04/25/1170894.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
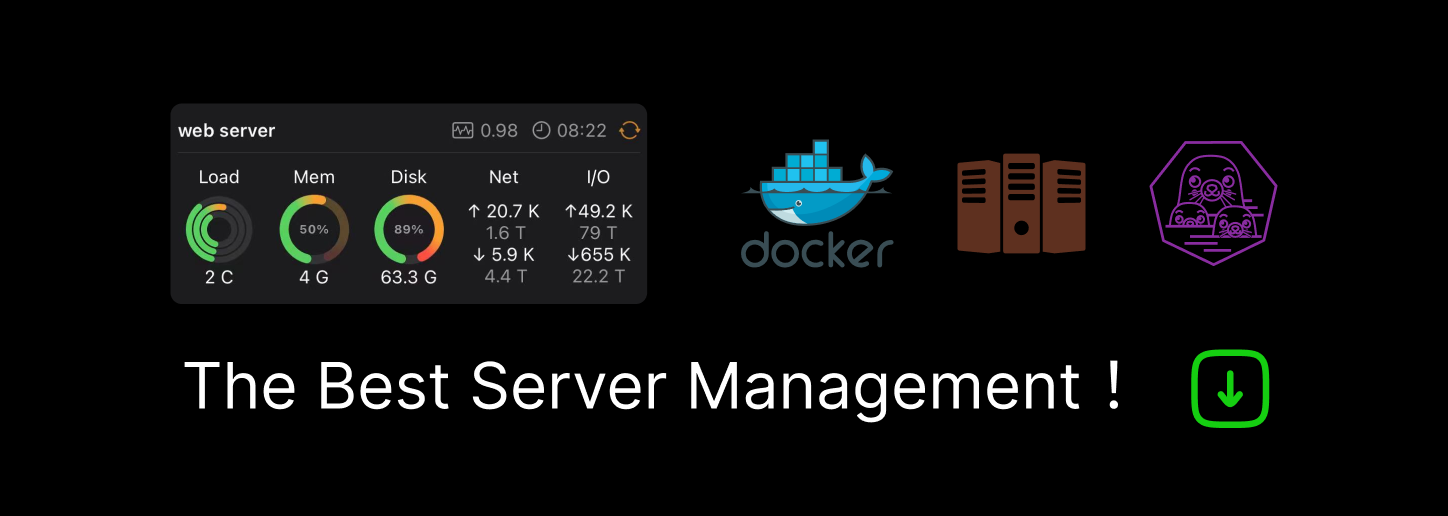
使用SharpZipLib实现zip压缩
使用国外开源加压解压库ICSharpCode.SharpZipLib实现加压,该库的官方网站为
http://www.icsharpcode.net/OpenSource/SharpZipLib/Download.aspx
使用体验:可以照着例子实现简单的加压解压,可以加压一个文件夹中的所有文件,但没有提供加压子文件夹的说明。
目前网上的一些代码有的无法加压空文件夹,有的加压了用rar解不开,这是一点需要改进的。
但如果只需要加压文件夹第一级子目录中的“文件”(不包括文件夹和子目录)的情况,使用这个库是很方便的。而且是正常zip格式。
比.Net提供的GZipStream类强在它可以按照标准zip格式加压多个文件,而GZipStream没有提供加压多个文件的方法,需要自己定义,
这样解压也只有使用自己的程序才可以,通用性方面不如SharpZipLib。
/// <summary>
/// 功能:压缩文件(暂时只压缩文件夹下一级目录中的文件,文件夹及其子级被忽略)
/// </summary>
/// <param name="dirPath">被压缩的文件夹夹路径</param>
/// <param name="zipFilePath">生成压缩文件的路径,为空则默认与被压缩文件夹同一级目录,名称为:文件夹名+.zip</param>
/// <param name="err">出错信息</param>
/// <returns>是否压缩成功</returns>
public bool ZipFile(string dirPath, string zipFilePath, out string err)
{
err = "";
if (dirPath == string.Empty)
{
err = "要压缩的文件夹不能为空!";
return false;
}
if (!Directory.Exists(dirPath))
{
err = "要压缩的文件夹不存在!";
return false;
}
//压缩文件名为空时使用文件夹名+.zip
if (zipFilePath == string.Empty)
{
if (dirPath.EndsWith("\\"))
{
dirPath = dirPath.Substring(0, dirPath.Length - 1);
}
zipFilePath = dirPath + ".zip";
}
try
{
string[] filenames = Directory.GetFiles(dirPath);
using (ZipOutputStream s = new ZipOutputStream(File.Create(zipFilePath)))
{
s.SetLevel(9);
byte[] buffer = new byte[4096];
foreach (string file in filenames)
{
ZipEntry entry = new ZipEntry(Path.GetFileName(file));
entry.DateTime = DateTime.Now;
s.PutNextEntry(entry);
using (FileStream fs = File.OpenRead(file))
{
int sourceBytes;
do
{
sourceBytes = fs.Read(buffer, 0, buffer.Length);
s.Write(buffer, 0, sourceBytes);
} while (sourceBytes > 0);
}
}
s.Finish();
s.Close();
}
}
catch (Exception ex)
{
err = ex.Message;
return false;
}
return true;
}
/// <summary>
/// 功能:解压zip格式的文件。
/// </summary>
/// <param name="zipFilePath">压缩文件路径</param>
/// <param name="unZipDir">解压文件存放路径,为空时默认与压缩文件同一级目录下,跟压缩文件同名的文件夹</param>
/// <param name="err">出错信息</param>
/// <returns>解压是否成功</returns>
public bool UnZipFile(string zipFilePath, string unZipDir, out string err)
{
err = "";
if (zipFilePath == string.Empty)
{
err = "压缩文件不能为空!";
return false;
}
if (!File.Exists(zipFilePath))
{
err = "压缩文件不存在!";
return false;
}
//解压文件夹为空时默认与压缩文件同一级目录下,跟压缩文件同名的文件夹
if (unZipDir == string.Empty)
unZipDir = zipFilePath.Replace(Path.GetFileName(zipFilePath), Path.GetFileNameWithoutExtension(zipFilePath));
if (!unZipDir.EndsWith("\\"))
unZipDir += "\\";
if (!Directory.Exists(unZipDir))
Directory.CreateDirectory(unZipDir);
try
{
using (ZipInputStream s = new ZipInputStream(File.OpenRead(zipFilePath)))
{
ZipEntry theEntry;
while ((theEntry = s.GetNextEntry()) != null)
{
string directoryName = Path.GetDirectoryName(theEntry.Name);
string fileName = Path.GetFileName(theEntry.Name);
if (directoryName.Length > 0)
{
Directory.CreateDirectory(unZipDir + directoryName);
}
if (!directoryName.EndsWith("\\"))
directoryName += "\\";
if (fileName != String.Empty)
{
using (FileStream streamWriter = File.Create(unZipDir + theEntry.Name))
{
int size = 2048;
byte[] data = new byte[2048];
while (true)
{
size = s.Read(data, 0, data.Length);
if (size > 0)
{
streamWriter.Write(data, 0, size);
}
else
{
break;
}
}
}
}
}//while
}
}
catch (Exception ex)
{
err = ex.Message;
return false;
}
return true;
}//解压结束
#endregion
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK