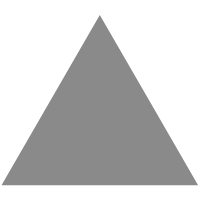
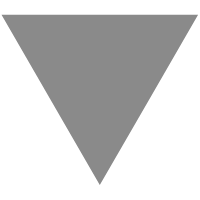
Variadic functions (...T)
source link: https://yourbasic.org/golang/variadic-function/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
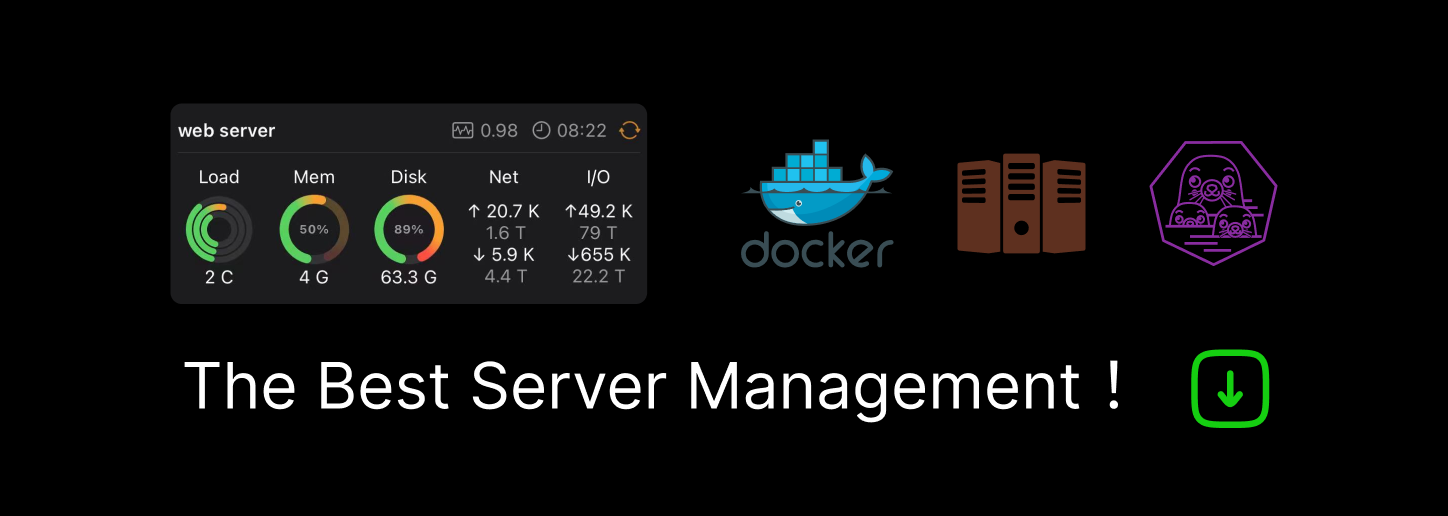
Variadic functions (...T)
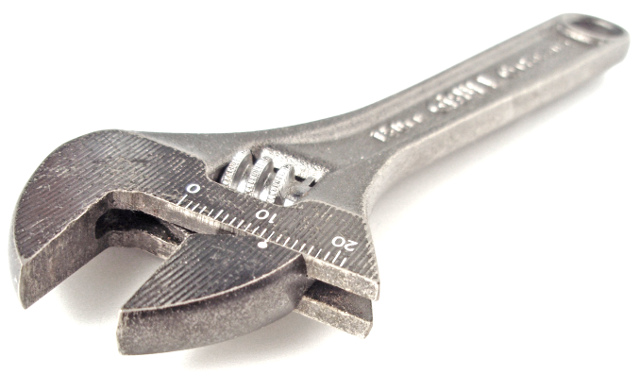
Basics
If the last parameter of a function has type ...T
it can be called with any number of trailing arguments of type T
.
func Sum(nums ...int) int {
res := 0
for _, n := range nums {
res += n
}
return res
}
func main()
fmt.Println(Sum()) // 0
fmt.Println(Sum(1, 2, 3)) // 6
}
The actual type of ...T
inside the function is []T
.
Pass slice elements to a variadic function
You can pass the elements of a slice s
directly to a variadic function using the s...
notation.
In this case no new slice is created.
primes := []int{2, 3, 5, 7}
fmt.Println(Sum(primes...)) // 17
Append is variadic
The built-in append function is variadic and can be used to append any number of elements to a slice.
As a special case, you can append a string to a byte slice:
var buf []byte
buf = append(buf, 'a', 'b')
buf = append(buf, "cd"...)
fmt.Println(buf) // [97 98 99 100]
Further reading
How to append anything (element, slice or string) to a slice
Share:
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK