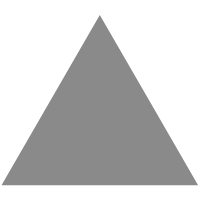
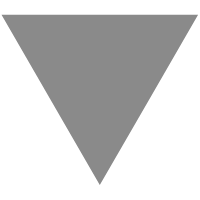
API with NestJS #16. Using the array data type with PostgreSQL and TypeORM
source link: https://wanago.io/2020/11/02/api-nestjs-array-data-type-postgresql-typeorm/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.

JavaScript NestJS SQL TypeScript
November 2, 2020Storing arrays is not an obvious thing in the world of SQL databases. Solutions such as MySQL, MariaDB, or Microsoft SQL Server don’t have a straightforward column type for arrays.
This article explores how the array data type works in PostgreSQL both through SQL queries and through TypeORM. By learning how to operate on arrays through SQL, we can better understand what the Postgres database is capable of. This will helps us quite a bit in using arrays through TypeORM.
The capabilities of the array data type in Postgres
Because databases such as MySQL don’t have an array data type, we might have had to work around this issue. One solution would be to create additional tables to store data that we would conceptualize as an array. Another solution would be to utilize the JSON data type available in MySQL and PostgreSQL.
To define the array data type column, we can append the square brackets or use the ARRAY keyword. Let’s play with our post table a bit and add the paragraphs column instead of content.
The text is a column data type that stores strings of any length
Although we could provide the array’s size, it does not affect the behavior of the database and might only serve as documentation.
When defining the array value input, we can use the curly braces notation or use the ARRAY keyword again.
Please note that we’ve surrounded the curly braces with single quotes and used the double quotes for strings
I lean more towards using the ARRAY keyword, and therefore, it is more frequently used in this article.
An important note is that PostgreSQL will keep the elements in the array in the order you’ve put them in.
Modifying an array
We can change an existing array, for example, by replacing it as a whole.
Another option is to update a single element.
We can also update just a slice of an array. The following code updates the second and the third element, leaving the first element unchanged:
PostgreSQL also supports concatenation with the use of the || operator. The following code creates a new array using the elements 1,2,5 and 6.
Searching through arrays
When searching through arrays, the ANY and ALL keywords can be very helpful.
To find a post where all paragraphs are equal to 'Apples', we can use the ALL command.
To look for a post when any paragraph equals Oranges, we can use the ANY keyword.
Multi-dimensional arrays
PostgresSQL also supports multi-dimensional arrays. To define them, we can use multiple square brackets.
Using them is similar to the regular arrays.
If you would like to use multi-dimensional arrays and need more examples, look at the official documentation.
Changing a regular column into an array
Above, we’ve completely dropped the content column. This might not be the best approach in production because it would result in data loss. Instead, let’s set the value of content to be the first element of the paragraphs array.
A simple way to achieve that is to:
- add the paragraphs column
- set its first element to be the value of the content column
- remove the content array
Keep in mind that PostgreSQL utilizes a one-based numbering convention. It means that the array starts with index number 1, not 0.
Using arrays with TypeORM
While knowing all of the above helps us understand what arrays can be used, our goal is to implement them with TypeORM and NestJS. To define a column that is an array, we need to add the array: true property.
Since we expect our users to send an array of text, we also need to change our Data Transfer Objects and their validation. To check if the property is an array of strings, we need the @IsString({ each: true }) decorator.
Doing the above is enough to start creating posts with the paragraphs array.

If you want to know how the author property is created in the above response, check out API with NestJS #3. Authenticating users with bcrypt, Passport, JWT, and cookies
When we look into the database, we can see that the paragraphs have been inserted properly.
Running more advanced queries on arrays
Since arrays are not a common data type in SQL databases, TypeORM might not support all of the features that we’ve used through regular SQL queries.
Fortunately, we can squeeze bare SQL queries into our NestJS code.
There is an issue with the above code, though. Here, we are constructing a raw SQL query using a parameter that might be provided by a user. This opens up us for a SQL injection.
Fortunately, with TypeORM, we can create a parameterized query. In the below example, the $1 would be replaced with a value of a paragraph.
The simple-array column type
A side note for creating array columns with TypeORM is that we are not completely out of luck if we don’t use PostgreSQL.
TypeORM has a special simple-array column type that uses a regular string column under the hood.
Even though TypeORM exposes the values as an array, it uses a single string column under the hood. All values are separated using a comma, so we can’t have any commas in our values.
Summary
In this article, we’ve gone through the concept of arrays in PostgreSQL both through writing SQL queries and using TypeORM. Thanks to knowing how to deal with arrays through SQL, we could better integrate them into our NestJS code. Fortunately, TypeORM allows us to write SQL queries ourselves, so the knowledge of Postgres really can come in handy.
Series Navigation<< API with NestJS #15. Defining transactions with PostgreSQL and TypeORMAPI with NestJS #17. Offset and keyset pagination with PostgreSQL and TypeORM >>Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK