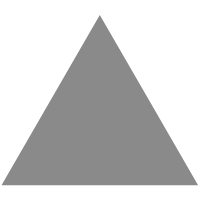
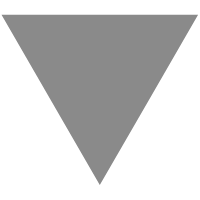
Python Tips and Tricks
source link: http://uzairadamjee.com/blog/python-tips/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
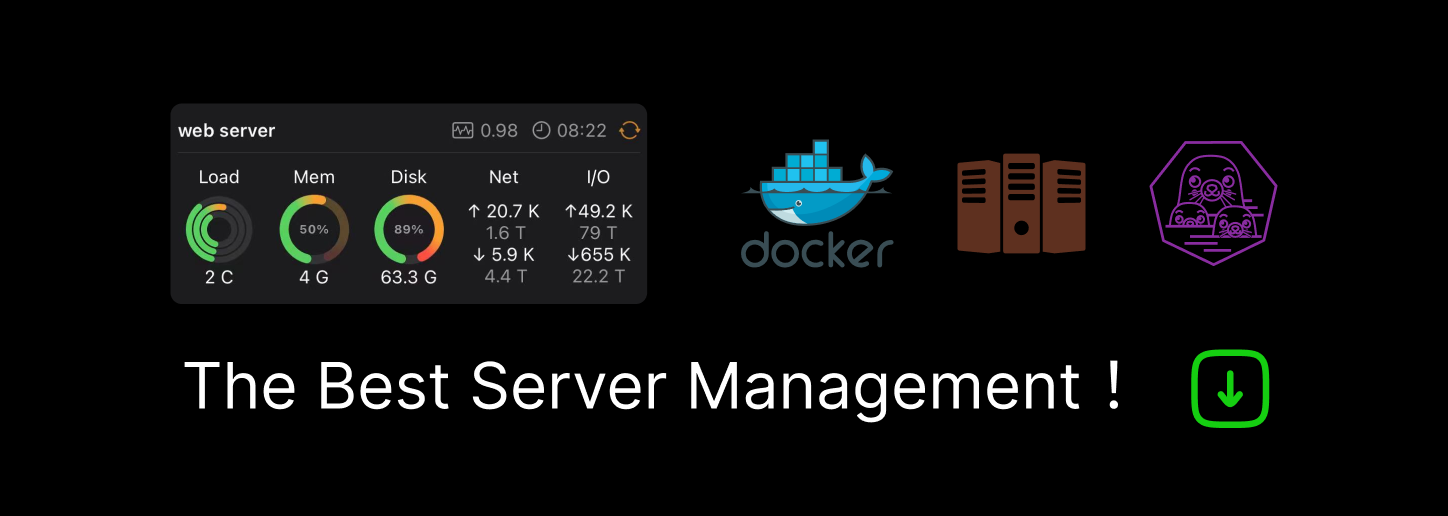

Python Tips and Tricks
Python is a beautiful language. It is an interpreted, high-level, general-purpose programming language. Python is dynamically typed and garbage-collected. It supports multiple programming paradigms, including structured (particularly, procedural), object-oriented, and functional programming.
Following are the python tips and tricks every Python programmer should know.
- Create a single string from all the elements in list
a = ["My", "name", "is", "Uzair"]
print(" ".join(a))
- Return Multiple Values From Functions
def x():
return 1, 2, 3, 4
a, b, c, d = x()
print(a, b, c, d)
- Find The Most Frequent Value In A List
test = [1, 2, 3, 4, 2, 2, 3, 1, 4, 4, 4]
print(max(set(test), key = test.count))
- Swap Variables In-Place
temp = x
x = y
y = temp
## OR
x, y = 8, 10
print(x, y)
x, y = y, x
print(x, y)
- Returning multiple values from a function
def get_multi_string():
x = "Karachi"
y = "Pakistan"
z = 5
return x, y, z
var = get_multi_string()
(x,y,z) = var
- Assigning multiple values in multiple variables
x, y = 10, 20
- Concatenate Strings
print('Python' + ' Coding' + ' Tips')
- Reverse a string
name = "Uzair"
name[::-1]
- Merging dictionaries
x = {'a': 1, 'b': 2}
y = {'b': 3, 'c': 4}
z = {**x, **y}
- Iterating over a dictionary
m = {'a': 1, 'b': 2, 'c': 3, 'd': 4}
for key, value in m.items():
print('{0}: {1}'.format(key, value))
- Iterating over list values while getting the index too
m = ['a', 'b', 'c', 'd']
for index, value in enumerate(m):
print('{0}: {1}'.format(index, value))
- Initializing empty collections
lst = list()
dic = dict()
s = set()
- Removing useless characters at the end of string
str1 = " Hello world "
str2 = "hello///"
str1.strip()
str2.strip("/")
- Removing duplicates items from a list
listNumbers = [1, 10, 10, 2, 2, 1, 5, 10, 20,30,50,20,100]
print("Original= ", listNumbers)
listNumbers = list(set(listNumbers))
print("After removing duplicate= ", listNumbers)
- The enumerate() function adds a counter to an iterable object.
subjects = ('Python', 'Coding', 'Tips')
for i, subject in enumerate(subjects):
print(i, subject)
- A set of strings and perform search in set using IF
objects = {"python", "coding", "tips", "for", "beginners"}
# Print set.
print(objects)
print(len(objects))
# Use of "in" keyword.
if "tips" in objects:
print("Found")
# Use of "not in" keyword.
if "test" not in objects:
print("Not Found")
Thank you for reading.
I hope you found this article useful! If there are tips you think I should have included or other suggestions then please do comment.
Happy coding!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK