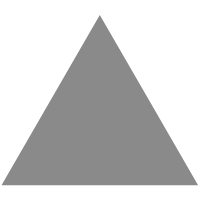
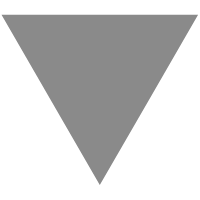
基于Python查找图像中很常见的颜色
source link: http://developer.51cto.com/art/202011/631212.htm
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
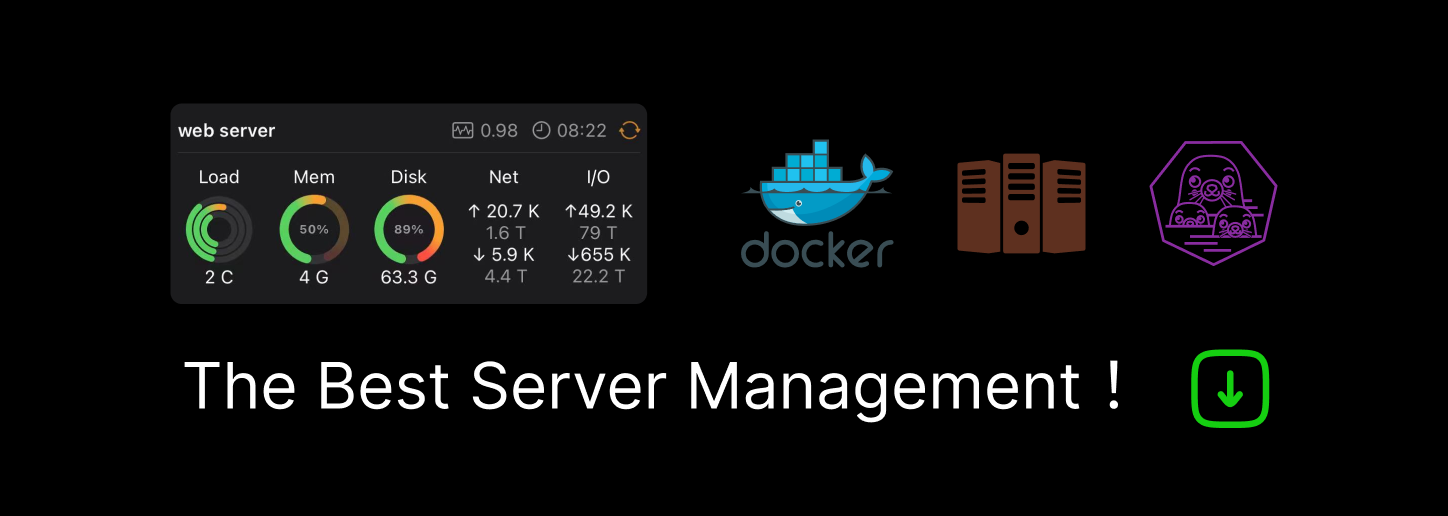
如果我们能够得知道一幅图像中最多的颜色是什么的话,可以帮助我们解决很多实际问题。例如在农业领域中想确定水果的成熟度,我们可以通过检查水果的颜色是否落在特定范围内,来判断它们是否已经成熟。
接下来我们将使用Python和一些常用库(例如Numpy,Matplotlib和OpenCV)来解决这个问题。
01. 准备工作
第一步:添加程序包
我们将在此处加载基本软件包。另外,由于我们要使用Jupyter进行编程,因此小伙伴们不要忘记添加%matplotlib inline命令。
第二步:加载并显示示例图像
我们将并排显示两个图像,因此我们需要做一个辅助函数。接下来我们将加载一些在本教程中将要使用的示例图像,并使用上述功能对其进行显示。
02. 常用方法
方法一:平均值
第一种方法是最简单(但无效)的方法-只需找到平均像素值即可。使用numpy的average功能,我们可以轻松获得行和宽度上的平均像素值-axis=(0,1)
img_temp = img.copy() img_temp[:,:,0], img_temp[:,:,1], img_temp[:,:,2] = np.average(img, axis=(0,1)) img_temp_2 = img_2.copy() img_temp_2[:,:,0], img_temp_2[:,:,1], img_temp_2[:,:,2] = np.average(img_2, axis=(0,1)) show_img_compar(img, img_temp) show_img_compar(img_2, img_temp_2)
从上面图像中可以看出,平均方法可能会产生错误结果,它给出的最常见的颜色可能并不是我们想要的颜色,这是因为平均值考虑了所有像素值。当我们具有高对比度的图像(一张图像中同时包含“浅色”和“深色”)时这个问题会很严重。在第二张图片中,这一点更加清晰。它为我们提供了一种新的颜色,该颜色在图像中根本看不到。
方法二:最高像素频率
第二种方法将比第一种更加准确。我们的工作就是计算每个像素值出现的次数。numpy给我们提供了一个函数可以完成这个任务。但是首先,我们必须调整图像数据结构的形状,以仅提供3个值的列表(每个R,G和B通道强度一个)。
我们可以使用numpy的reshape函数来获取像素值列表。
现在我们已经有了正确结构的数据,可以开始计算像素值的频率了,使用numpy中的unique函数即可。
img_temp = img.copy() unique, counts = np.unique(img_temp.reshape(-1, 3), axis=0, return_counts=True) img_temp[:,:,0], img_temp[:,:,1], img_temp[:,:,2] = unique[np.argmax(counts)] img_temp_2 = img_2.copy() unique, counts = np.unique(img_temp_2.reshape(-1, 3), axis=0, return_counts=True) img_temp_2[:,:,0], img_temp_2[:,:,1], img_temp_2[:,:,2] = unique[np.argmax(counts)] show_img_compar(img, img_temp) show_img_compar(img_2, img_temp_2)
比第一个更有意义吗?最常见的颜色是黑色区域。但是如果我们不仅采用一种最常见的颜色,还要采用更多的颜色怎么办?使用相同的概念,我们可以采用N种最常见的颜色。换句话说,我们要采用最常见的不同颜色群集该怎么办。
方法三:使用K均值聚类
我们可以使用著名的K均值聚类将颜色组聚类在一起。
def palette(clusters): width=300 palette = np.zeros((50, width, 3), np.uint8) steps = width/clusters.cluster_centers_.shape[0] for idx, centers in enumerate(clusters.cluster_centers_): palette[:, int(idx*steps):(int((idx+1)*steps)), :] = centers return palette clt_1 = clt.fit(img.reshape(-1, 3)) show_img_compar(img, palette(clt_1)) clt_2 = clt.fit(img_2.reshape(-1, 3)) show_img_compar(img_2, palette(clt_2))
容易吧!现在,我们需要的是一个显示上面的颜色簇并立即显示的功能。我们只需要创建一个高度为50,宽度为300像素的图像来显示颜色组/调色板。对于每个颜色簇,我们将其分配给我们的调色板。
是不是很漂亮?就图像中最常见的颜色而言,K均值聚类给出了出色的结果。在第二张图像中,我们可以看到调色板中有太多的棕色阴影。这很可能是因为我们选择了太多的群集。让我们看看是否可以通过选择较小的k值来对其进行修复。
def palette(clusters): width=300 palette = np.zeros((50, width, 3), np.uint8) steps = width/clusters.cluster_centers_.shape[0] for idx, centers in enumerate(clusters.cluster_centers_): palette[:, int(idx*steps):(int((idx+1)*steps)), :] = centers return palette clt_3 = KMeans(n_clusters=3) clt_3.fit(img_2.reshape(-1, 3)) show_img_compar(img_2, palette(clt_3))
由于我们使用K均值聚类,因此我们仍然必须自己确定适当数量的聚类。三个集群似乎是一个不错的选择。但是我们仍然可以改善这些结果,并且仍然可以解决集群问题。我们还如何显示群集在整个图像中所占的比例?
方法四:K均值+比例显示
我们需要做的就是修改我们的palette功能。代替使用固定步骤,我们将每个群集的宽度更改为与该群集中的像素数成比例。
from collections import Counter def palette_perc(k_cluster): width = 300 palette = np.zeros((50, width, 3), np.uint8) n_pixels = len(k_cluster.labels_) counter = Counter(k_cluster.labels_) # count how many pixels per cluster perc = {} for i in counter: perc[i] = np.round(counter[i]/n_pixels, 2) perc = dict(sorted(perc.items())) #for logging purposes print(perc) print(k_cluster.cluster_centers_) step = 0 for idx, centers in enumerate(k_cluster.cluster_centers_): palette[:, step:int(step + perc[idx]*width+1), :] = centers step += int(perc[idx]*width+1) return palette clt_1 = clt.fit(img.reshape(-1, 3)) show_img_compar(img, palette_perc(clt_1)) clt_2 = clt.fit(img_2.reshape(-1, 3)) show_img_compar(img_2, palette_perc(clt_2))
它不仅为我们提供了图像中最常见的颜色。这也给了我们每个像素出现的比例。
03. 结论
我们介绍了几种使用Python以及最知名的库来获取图像中最常见颜色的技术。另外,我们还看到了这些技术的优缺点。到目前为止,使用k> 1的K均值找到最常见的颜色是找到图像中最频繁的颜色的最佳解决方案之一。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK