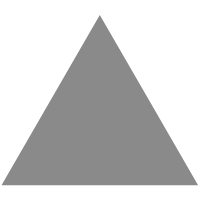
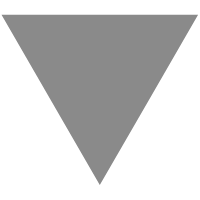
spring-boot-route(八)整合mybatis操作数据库
source link: http://www.cnblogs.com/zhixie/p/13779876.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
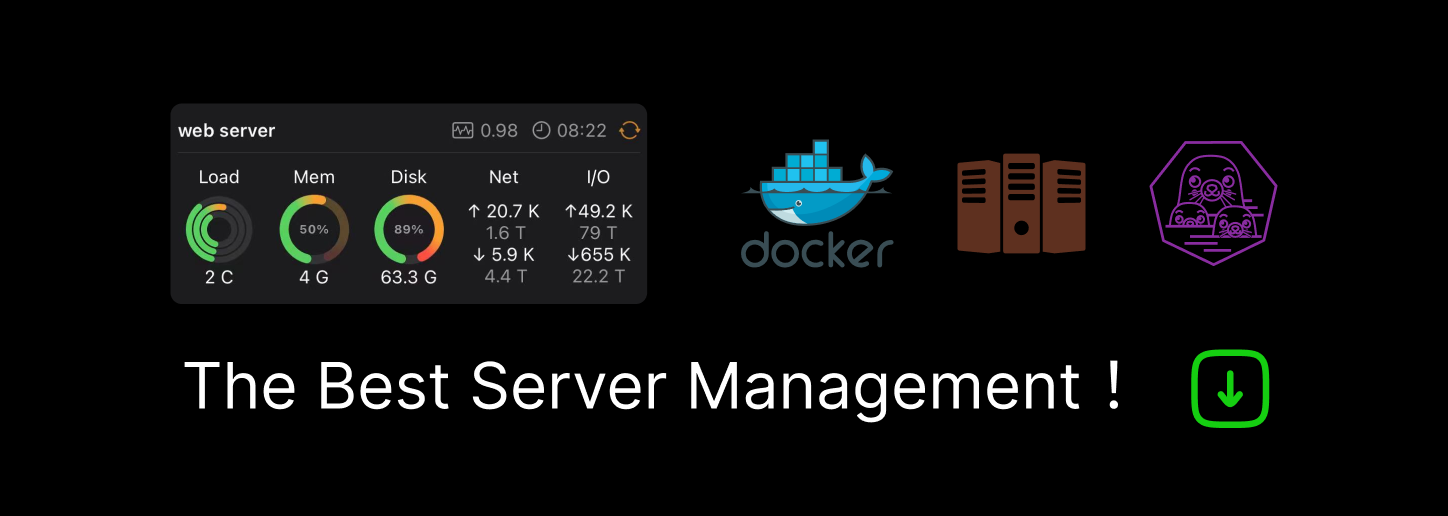
MyBatis 是一款优秀的持久层框架,它支持定制化 SQL、存储过程以及高级映射。MyBatis 避免了几乎所有的 JDBC 代码和手动设置参数以及获取结果集。MyBatis 可以使用简单的 XML 或注解来配置和映射原生信息,将接口和 Java 的 POJOs(Plain Ordinary Java Object,普通的 Java对象)映射成数据库中的记录。
通过注解完成数据操作
第一步:引入mysql依赖和mybatis依赖
<dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> </dependency> <dependency> <groupId>org.mybatis.spring.boot</groupId> <artifactId>mybatis-spring-boot-starter</artifactId> <version>LATEST</version> </dependency>
第二步:新建学生表及对应的实体类
CREATE TABLE `student` ( `student_id` int(30) NOT NULL AUTO_INCREMENT, `age` int(1) DEFAULT NULL COMMENT '年龄', `name` varchar(45) DEFAULT NULL COMMENT '姓名', `sex` int(1) DEFAULT NULL COMMENT '性别:1:男,2:女,0:未知', `create_time` datetime DEFAULT NULL COMMENT '创建时间', `status` int(1) DEFAULT NULL COMMENT '状态:1:正常,-1:删除', PRIMARY KEY (`student_id`) ) ENGINE=InnoDB AUTO_INCREMENT=617354 DEFAULT CHARSET=utf8mb4 CHECKSUM=1 DELAY_KEY_WRITE=1 ROW_FORMAT=DYNAMIC COMMENT='学生表'
@Data @NoArgsConstructor @AllArgsConstructor public class Student implements Serializable { private static final long serialVersionUID = 6712540741269055064L; private Integer studentId; private Integer age; private String name; private Integer sex; private Date createTime; private Integer status; }
第三步:配置数据库连接信息
spring: datasource: driver-class-name: com.mysql.cj.jdbc.Driver url: jdbc:mysql://localhost:3306/simple_fast username: root password: root
增删改查
@Mapper public interface StudentMapper { @Select("select * from student where student_id = #{studentId}") Student findById(@Param("studentId") Integer studentId); @Insert("insert into student(age,name) values(#{age},#{name})") int addStudent(@Param("name") String name,@Param("age") Integer age); @Update("update student set name = #{name} where student_id = #{studentId}") int updateStudent(@Param("studentId") Integer studentId,@Param("name") String name); @Delete("delete from student where student_id = #{studentId}") int deleteStudent(@Param("studentId") Integer studentId); }
上面演示的传参方式是通过单个参数传递的, 如果想通过Map或实体类传参数,就不需要使用@Param来绑定参数了,将map中的key或者实体类中的属性与sql中的参数值对应上就可以了 。
通过XML配置完成数据操作
@Mapper和@MapperScan
@Mapper加在数据层接口上,将其注册到ioc容器上,@MapperScan加在启动类上,需要指定扫描的数据层接口包。如下:
@Mapper public interface StudentMapper {}
@SpringBootApplication @MapperScan("com.javatrip.mybatis.mapper") public class MybatisApplication { public static void main(String[] args) { SpringApplication.run(MybatisApplication.class, args); } }
两个注解的作用一样,在开发中为了方便,通常我们会使用@MapperScan。
指定mapper.xml的位置
mybatis: mapper-locations: classpath:mybatis/*.xml
开启数据实体映射驼峰命名
mybatis: configuration: map-underscore-to-camel-case: true
编写xml和与之对应的mapper接口
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <mapper namespace="com.javatrip.mybatis.mapper.StudentXMapper"> <select id="findById" resultType="com.javatrip.mybatis.entity.Student"> select * from student where student_id = #{studentId} </select> <insert id="addStudent" parameterType="com.javatrip.mybatis.entity.Student"> insert into student(name,age) values(#{name},#{age}) </insert> <update id="updateStudent" parameterType="com.javatrip.mybatis.entity.Student"> update student set name = #{name} where student_id = #{studentId} </update> <delete id="deleteStudent" parameterType="Integer"> delete from student where student_id = #{studentId} </delete> </mapper>
@Mapper public interface StudentXMapper { Student findById(@Param("studentId") Integer studentId); int addStudent(Student student); int updateStudent(@Param("studentId") Integer studentId,@Param("name") String name); int deleteStudent(@Param("studentId") Integer studentId); }
编写测试类
@SpringBootTest class MybatisApplicationTests { @Autowired StudentMapper mapper; @Autowired StudentXMapper xMapper; @Test void testMapper() { Student student = mapper.findById(10); mapper.addStudent("Java旅途",19); mapper.deleteStudent(31); mapper.updateStudent(10,"Java旅途"); } @Test void contextLoads() { Student student = xMapper.findById(10); Student studentDo = new Student(); studentDo.setAge(18); studentDo.setName("Java旅途呀"); xMapper.addStudent(studentDo); xMapper.deleteStudent(32); xMapper.updateStudent(31,"Java旅途"); } }
这里有几个需要注意的点:mapper标签中namespace属性对应的是mapper接口;select标签的id对应mapper接口中的方法名字;select标签的resultType对应查询的实体类,使用全路径。
此是spring-boot-route系列的第八篇文章,这个系列的文章都比较简单,主要目的就是为了帮助初次接触Spring Boot 的同学有一个系统的认识。本文已收录至我的 github
,欢迎各位小伙伴 star
!
github: https://github.com/binzh303/spring-boot-route
点关注、不迷路
如果觉得文章不错,欢迎 关注 、 点赞 、 收藏 ,你们的支持是我创作的动力,感谢大家。
如果文章写的有问题,请不要吝啬,欢迎留言指出,我会及时核查修改。
如果你还想更加深入的了解我,可以微信搜索「 Java旅途 」进行关注。回复「 1024 」即可获得学习视频及精美电子书。每天7:30准时推送技术文章,让你的上班路不在孤独,而且每月还有送书活动,助你提升硬实力!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK