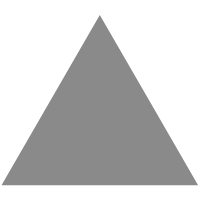
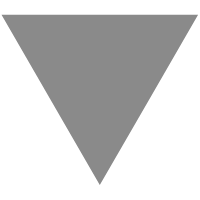
Vue项目(电商后台管理)优化推荐
source link: https://segmentfault.com/a/1190000023767727
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
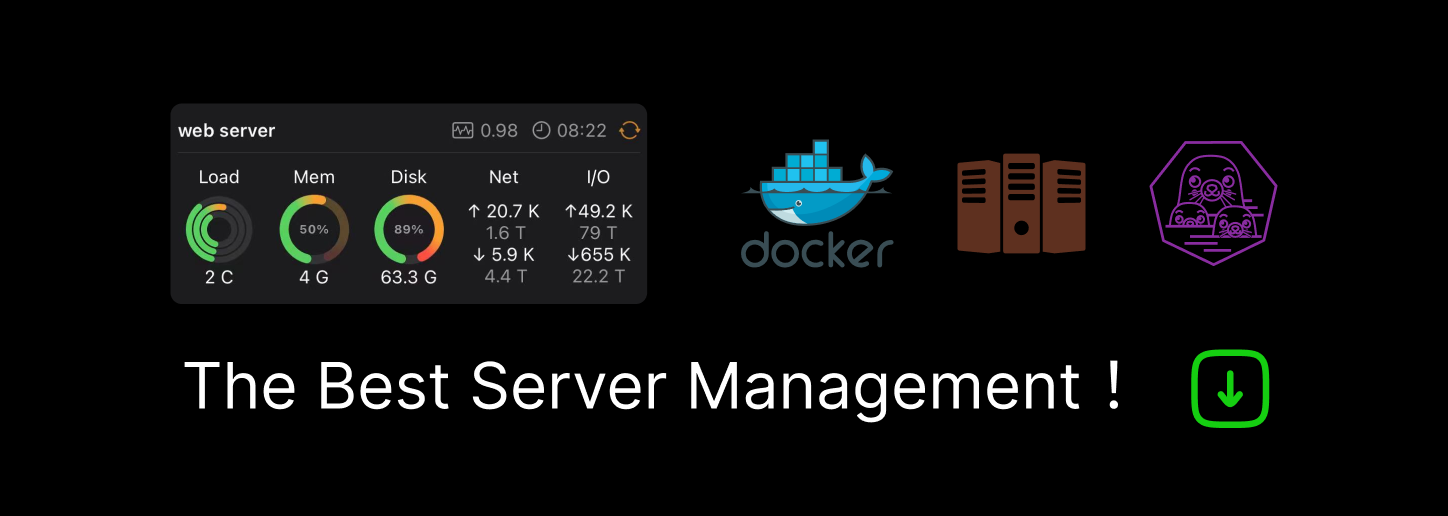
项目优化--进度条添加
- 安装nprogress,ui界面依赖安装依赖,搜索nprogress进行安装即可
- 也可以在终端中安装 npm i nprogress -S
-
在main.js中导入进度条组件
//导入进度条插件 import NProgress from 'nprogress' //导入进度条样式 import 'nprogress/nprogress.css'
-
利用NProgress.start()显示进度条(利用请求拦截器)
NProgress.start()
-
利用NProgress.done()隐藏进度条(利用响应拦截器)
NProgress.done()
移除打包之后的console (babel.config.js 中设置)
- 安装babel-plugin-transform-remove-console
- 在终端中下载 npm i babel-plugin-transform-remove-console -S
-
在plugins节点下新增"transform-remove-console" ()
"plugins": [ [ "component", { "libraryName": "element-ui", "styleLibraryName": "theme-chalk" } ], // 这个是对开发和发布环境的下的所有console都是禁止输出的 "transform-remove-console" ]
只在发布阶段移除console
-
利用process.env.NODE_ENV判断当前是发布是开发阶段
var prodPlugins = [] if(process.env.NODE_ENV == 'production'){ prodPlugins.push('transform-remove-console') }
-
利用展开运算符将数组里面的插件交给plugins
"plugins": [ [ "component", { "libraryName": "element-ui", "styleLibraryName": "theme-chalk" } ], ...prodPlugins ]
利用vue.config.js修改webpack的默认配置
- 创建vue.config.js文件
-
js内部暴露出一个配置对象
module.exports = { // 打包文件后会产生一个dist文件夹里面的index.html可以直接双击运行 publicPath: './', }
自定义入口文件
-
利用chainWebpack配置不同环境设置不同的入口文件
module.exports = { // 打包文件后会产生一个dist文件夹里面的index.html可以直接双击运行 publicPath: './', chainWebpack:config=>{ // 当前环境为 发布阶段 config.when(process.env.NODE_ENV == 'production',config=>{ config.entry('app').clear().add('./src/main-prod.js') }) // 当前环境为 开发阶段 config.when(process.env.NODE_ENV == 'development',config=>{ config.entry('app').clear().add('./src/main-dev.js') }) } }
通过cdn加载外部资源(就近的获取服务器资源)
- 因为入口文件中通过import导入包的方式,最终都会打包到同一个js文件中,所以导致chunk-vendors文件体积过大
-
既然这些文件都打包到同一个js文件里体积过大,所以忽略一些js文件不打包(只需要配置发布阶段即可)
// 使用externals设置排除项 // 在vue.config.js 中的发布模式下填写 config.set('externals',{ vue:'Vue', 'vue-router':'VueRouter', axios:'axios', echarts:'echarts', nprogress:'NProgress', 'vue-quill-editor':'VueQuillEditor' })
-
虽然忽略了一些js文件,但是在入口文件中还有一些css文件体积也比较大,所以直接将引入的css删除
import VueQuillEditor from 'vue-quill-editor' // 富文本编辑器 import 'quill/dist/quill.core.css' import 'quill/dist/quill.snow.css' import 'quill/dist/quill.bubble.css'
-
将上面css删除后不打包,最终的样式肯定会有问题,所以我们在public中index.html中引入cdn的资源文件
<!-- nprogress 的样式表文件 --> <link rel="stylesheet" href="https://cdn.staticfile.org/nprogress/0.2.0/nprogress.min.css"/> <!-- 富文本编辑器 的样式表文件 --> <link rel="stylesheet" href="https://cdn.staticfile.org/quill/1.3.4/quill.core.min.css" /> <link rel="stylesheet" href="https://cdn.staticfile.org/quill/1.3.4/quill.snow.min.css" /> <link rel="stylesheet" href="https://cdn.staticfile.org/quill/1.3.4/quill.bubble.min.css" />
-
对应的js文件我们也忽略了没有打包,所以也是直接在public中index.html中引入cdn的资源
<script src="https://cdn.staticfile.org/vue/2.5.22/vue.min.js"></script> <script src="https://cdn.staticfile.org/vue-router/3.0.1/vue-router.min.js"></script> <script src="https://cdn.staticfile.org/axios/0.18.0/axios.min.js"></script> <script src="https://cdn.staticfile.org/echarts/4.1.0/echarts.min.js"></script> <script src="https://cdn.staticfile.org/nprogress/0.2.0/nprogress.min.js"></script> <!-- 富文本编辑器的 js 文件 --> <script src="https://cdn.staticfile.org/quill/1.3.4/quill.min.js"></script> <script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/vue-quill-editor.js"></script>
配置element-ui的cdn资源
- 删除入口文件中的按需导入的elemen.js
-
导入element的cdn资源
<!-- element-ui 的样式表文件 --> <link rel="stylesheet" href="https://cdn.staticfile.org/element-ui/2.13.0/theme-chalk/index.css" /> <!-- element-ui 的 js 文件 --> <script src="https://cdn.staticfile.org/element-ui/2.13.0/index.js"></script>
根据不同环境定制不同的首页内容
我们要在index.html中来判断当前环境为开发阶段还是发布阶段,但是在index.html无法获取到process.env.NODE_ENV,但是index.html中可以获取到htmlWebpackPlugin该插件的配置数据。
为什么?
- 第一个作用获取到index.html,在生成一个新的index.html
- 会自动引入打包的js和css文件
-
如果是发布阶段,我们给htmlWebpackPlugin添加一个标识为true
//使用插件 config.plugin('html').tap(args=>{ //添加参数isProd args[0].isProd = true return args })
-
如果是开发阶段也添加一个标识,为flase
//使用插件 config.plugin('html').tap(args=>{ //添加参数isProd args[0].isProd = false return args })
-
在页面通过htmlWebpackPlugin获取到标识,判断该标识为true还是false,如果是true代表发布阶段,应该添加cdn资源,如果是false不需要添加
<% if(htmlWebpackPlugin.options.isProd){ %> cdn资源文件 <% } %>
实现路由懒加载
又名:路由按需加载
当匹配某个路由时,才去加载对应的资源文件
- 安装 @babel/plugin-syntax-dynamic-import
-
配置babel-plugins
"plugins": [ [ "component", { "libraryName": "element-ui", "styleLibraryName": "theme-chalk" } ], ...prodPlugins, "@babel/plugin-syntax-dynamic-import" ]
-
修改引入方式
const Login = () => import(/* webpackChunkName: "login_home_welcome" */ '../views/Login.vue') const Home = () => import(/* webpackChunkName: "login_home_welcome" */ '../views/Home.vue') const Welcome = () => import(/* webpackChunkName: "login_home_welcome" */ '../views/Welcome.vue') const Users = () => import(/* webpackChunkName: "user" */ '../views/users/Users.vue') const Rights = () => import(/* webpackChunkName: "right_roles" */ '../views/power/Rights.vue') const Roles = () => import(/* webpackChunkName: "right_roles" */ '../views/power/Roles.vue') const GoodCate = () => import(/* webpackChunkName: "goods" */ '../views/goods/cate.vue') const GoddsParams = () => import(/* webpackChunkName: "goods" */ '../views/goods/params.vue') const GoddsList = () => import(/* webpackChunkName: "goods" */ '../views/goods/list.vue') const GoodsAdd = () => import(/* webpackChunkName: "goods" */ '../views/goods/add.vue') const Order = () => import(/* webpackChunkName: "order" */ '../views/Order.vue') const Reports = () => import(/* webpackChunkName: "report" */ '../views/Reports.vue')
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK