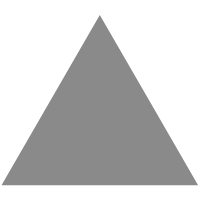
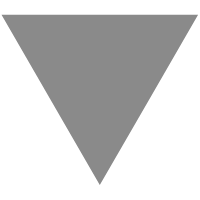
Qt-数据库操作SQLite
source link: http://www.cnblogs.com/mrlayfolk/p/13334881.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
1 简介
参考视频: https://www.bilibili.com/video/BV1XW411x7NU?p=88
说明:本文对在Qt中操作SQLite做简要说明。
SQLite:SQLite 是一个软件库,实现了自给自足的、无服务器的、零配置的、事务性的 SQL 数据库引擎。具体的操作命令可参考: https://www.runoob.com/sqlite/sqlite-tutorial.html
在Qt中操作SQLite不需要我们单独先安装它,可以直接使用。
2 测试及说明
语法就不介绍了,这里说明功能:创建一个info.db数据库,插入一些数据,然后遍历输出。
代码步骤说明:
(1)添加sqlite数据库
1 db = QSqlDatabase::addDatabase("QSQLITE");
(2)创建数据库
语法和之前操作MySql一样:
1 QSqlQuery query; 2 query.exec("create table student(id int primary key, name varchar(255), age int, score int);");
(3)批量插入条目
1 //批量插入:odbc风格 2 //预处理语句 3 query.prepare("insert into student(name, age, score) values(?, ?, ?);"); 4 // 给字段设置内容 5 QVariantList nameList; 6 nameList << "xiaoming" << "xiaokong" << "xiaojiang"; 7 QVariantList ageList; 8 ageList << 22 << 21 << 24; 9 QVariantList scoreList; 10 scoreList << 89 << 99 << 78; 11 //给字段绑定相应的值,必须按顺序绑定 12 query.addBindValue(nameList); 13 query.addBindValue(ageList); 14 query.addBindValue(scoreList); 15 //执行预处理命令 16 query.execBatch();
(4)遍历输出
1 query.exec("select * from student"); 2 while (true == query.next()) { //一行一行遍历 3 //取出当前行的内容,以列为单位 4 qDebug() << query.value(0).toInt() //取第一列 5 << query.value(1).toString() //取第二列 6 << query.value("age").toInt() 7 << query.value("score").toInt(); 8 }
完整代码:
1 #include "widget.h" 2 #include "ui_widget.h" 3 #include <QDebug> 4 #include <QSqlDatabase> 5 #include <QMessageBox> 6 #include <QSqlError> 7 #include <QSqlQuery> 8 9 10 Widget::Widget(QWidget *parent) : 11 QWidget(parent), 12 ui(new Ui::Widget) 13 { 14 ui->setupUi(this); 15 16 //打印qt支持的数据库驱动 17 qDebug() << QSqlDatabase::drivers(); 18 19 //添加sqlite数据库 20 db = QSqlDatabase::addDatabase("QSQLITE"); 21 //设置数据库 22 db.setDatabaseName("../info.db"); 23 //打开数据库 24 if (db.open() == false) { 25 QMessageBox::warning(this, "错误", db.lastError().text()); 26 return; 27 } 28 //操作sql语句 29 QSqlQuery query; 30 query.exec("create table student(id int primary key, name varchar(255), age int, score int);"); 31 //批量插入:odbc风格 32 //预处理语句 33 query.prepare("insert into student(name, age, score) values(?, ?, ?);"); 34 // 给字段设置内容 35 QVariantList nameList; 36 nameList << "xiaoming" << "xiaokong" << "xiaojiang"; 37 QVariantList ageList; 38 ageList << 22 << 21 << 24; 39 QVariantList scoreList; 40 scoreList << 89 << 99 << 78; 41 //给字段绑定相应的值,必须按顺序绑定 42 query.addBindValue(nameList); 43 query.addBindValue(ageList); 44 query.addBindValue(scoreList); 45 //执行预处理命令 46 query.execBatch(); 47 48 query.exec("select * from student"); 49 while (true == query.next()) { //一行一行遍历 50 //取出当前行的内容,以列为单位 51 qDebug() << query.value(0).toInt() //取第一列 52 << query.value(1).toString() //取第二列 53 << query.value("age").toInt() 54 << query.value("score").toInt(); 55 } 56 } 57 58 Widget::~Widget() 59 { 60 delete ui; 61 } View Code
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK