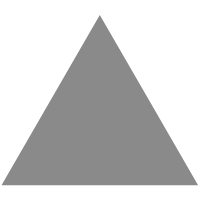
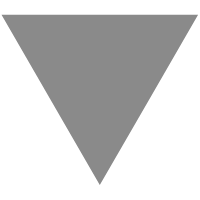
mybatis入门学习
source link: http://www.cnblogs.com/shuimutong/p/13246474.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
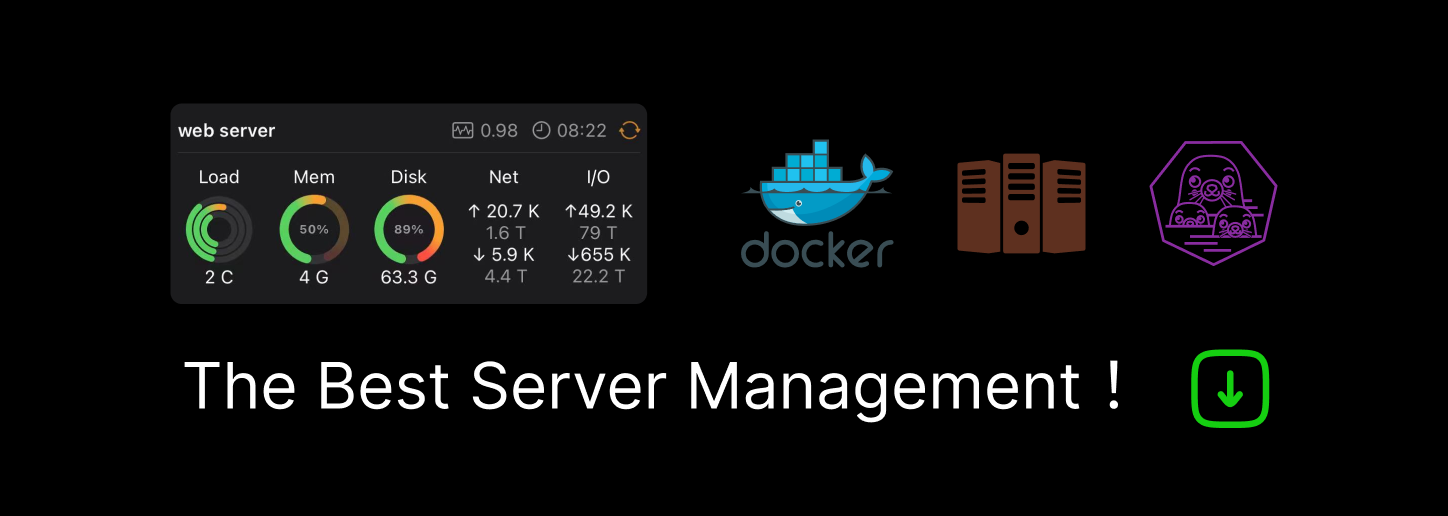
一、背景
很久以前听说过ibatis,后来知道ibatis改名mybatis了,之前只是简单的接触过mybatis,没有使用太多,不是太了解。所以趁着周末看了看。
本文主要参考网站:
https://mybatis.org/mybatis-3/zh/index.html
https://www.cnblogs.com/benjieqiang/p/11183580.html
http://www.mybatis.cn/679.html
网上关于mybatis的入门文章很多,但是经过实践,发现许多写的都不全。有些代码根本就只是一个示例,根本就无法执行。
写这篇文章,一方面是记录自己的学习,另一方面是给像我这样的小白参考。能够真正执行的代码。
(文章是有目录的,在右下角。。可能需要滚动一下屏幕才出来)
二、mybatis简单使用
1、引入依赖
<dependency> <groupId>org.mybatis</groupId> <artifactId>mybatis</artifactId> <version>${mybatis.version}</version> </dependency> <dependency> <groupId>org.mybatis.spring.boot</groupId> <artifactId>mybatis-spring-boot-starter</artifactId> <version>1.3.0</version> </dependency> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <scope>runtime</scope> </dependency>
全部依赖请查看文末的git源码。
2、对象类
@Data //使用了lombok,自动生成get/set方法 public class User { private Long id; private String name; private Integer age; }
3、对应的数据库表语句
CREATE TABLE `t_user` ( `id` bigint(20) unsigned NOT NULL AUTO_INCREMENT COMMENT '主键', `name` varchar(255) NOT NULL DEFAULT '' COMMENT '名称', `age` int(15) unsigned NOT NULL DEFAULT 0 COMMENT '年龄', PRIMARY KEY (`id`) ) ENGINE=InnoDB AUTO_INCREMENT=1 DEFAULT CHARSET=utf8 COMMENT='用户表';
4、在resources目录下创建Mapper
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <!--namespace:用来区别不同的类的名字 --> <mapper namespace="test"> <!-- 通过Id查询一个用户 --> <select id="findUserById" parameterType="Integer" resultType="com.shuimutong.learn.mybatis.domain.User"> select * from t_user where id = #{v} </select> </mapper>
5、在resources下创建mybatis连接数据库的配置文件
1)mybatis-config.xml
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd"> <configuration> <properties resource="mysql.properties"></properties> <environments default="mysql"> <environment id="mysql"> <transactionManager type="JDBC"/> <dataSource type="POOLED"> <property name="driver" value="${jdbc.driver}"/> <property name="url" value="${jdbc.url}"/> <property name="username" value="${jdbc.username}"/> <property name="password" value="${jdbc.password}"/> </dataSource> </environment> </environments> <mappers> <mapper resource="mapper/UserMapper.xml"/> <mapper resource="mapper/User2Mapper.xml"/> </mappers> </configuration>
2)相同目录下创建对应的mysql.properties
jdbc.driver=com.mysql.jdbc.Driver jdbc.url=jdbc:mysql://localhost:3306/simple?useUnicode=ture&characterEncoding=UTF-8&serverTimezone=GMT%2B8 jdbc.username=simple jdbc.password=123456
这一步主要是想把经常变动的部分和变的部分分开,也可以选择不要这个文件,直接写在mybatis-config.xml中。如果直接在xml文件中,需要主要“&”的转义,应该使用“&”(大概是这个,我是根据IDEA提示改的),不然文件会报错。
6、代码调用
public class UserTest { public static void main(String[] args) throws IOException { //1.读取配置文件 InputStream in = Resources.getResourceAsStream("mybatis-config.xml"); //2.创建SqlSessionFactory工厂 SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(in); //3.使用工厂生产SqlSession对象 SqlSession session = sqlSessionFactory.openSession(); //4.执行Sql语句 User user = session.selectOne("test.findUserById", 2); //5. 打印结果 System.out.println(JSON.toJSONString(user)); //6.释放资源 session.close(); in.close(); } }
小结:
代码写到这里,你应该已经学会了mybatis的基本使用。
但是工作中如果用到,许多情况下会跟其他框架一起使用,比如说springboot。
三、mybatis+springboot使用
1、引入依赖
因为我引入的依赖较多,所以请直接通过git查看,那里最全。
2、User对象和sql
和(二)中的一样。
3、创建User3Mapper接口
已经使用spring了,不能再通过Session工厂找方法了,要通过定义的接口去访问。(工作中要保证少出错,虽然代码会一些)
package com.shuimutong.learn.mybatis.dao; import com.shuimutong.learn.mybatis.domain.User; import org.apache.ibatis.annotations.Mapper; @Mapper public interface User3Mapper { User selectUser(long id); }
4、创建User3Mapper对应的xml
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <!--和定义的接口对应上--> <mapper namespace="com.shuimutong.learn.mybatis.dao.User3Mapper"> <!--java对象和数据库中表字段的映射关系--> <resultMap id="User3Map" type="com.shuimutong.learn.mybatis.domain.User"> <id column="id" property="id" jdbcType="BIGINT"/> <result column="name" property="name" jdbcType="VARCHAR"/> <result column="age" property="age" jdbcType="INTEGER"/> </resultMap> <sql id="BaseColumn"> id, name, age </sql> <select id="selectUser" parameterType="java.lang.Long" resultMap="User3Map"> SELECT <include refid="BaseColumn" /> FROM t_user WHERE id = #{id, jdbcType=BIGINT} </select> </mapper>
5、创建application.yml
在resources目录下创建此文件。
server: port: 8080 spring: #数据库连接 datasource: driver-class-name: com.mysql.cj.jdbc.Driver url: jdbc:mysql://localhost:3306/simple?useUnicode=ture&characterEncoding=UTF-8&serverTimezone=GMT%2B8 username: simple password: 123456 mybatis: type-aliases-package: com.shuimutong.learn.mybatis.domain mapperLocations: classpath:mapper/*.xml
6、创建UserService方法
前面的User3Mapper接口相当于是UserDao,User3Mapper.xml相当于UserDao的实现。
DAO层写了,所以这里就是service层了。
package com.shuimutong.learn.mybatis.service.impl; import com.alibaba.fastjson.JSON; import com.shuimutong.learn.mybatis.dao.User3Mapper; import com.shuimutong.learn.mybatis.domain.User; import com.shuimutong.learn.mybatis.service.UserService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; @Service public class UserServiceImpl implements UserService { @Autowired private User3Mapper user3Mapper; @Override public User findById(long id) { System.out.println("user3MapperIsNull?"); System.out.println(JSON.toJSONString(user3Mapper)); return user3Mapper.selectUser(id); } }
UserService代码这里就不贴了。
7、创建UserController
创建controller代码,咱这可是springboot项目。
package com.shuimutong.learn.mybatis.controller; import com.shuimutong.learn.mybatis.domain.User; import com.shuimutong.learn.mybatis.service.UserService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController; @RestController public class UserController { @Autowired private UserService userService; @GetMapping("/getUser") public User getUser(long id) { return userService.findById(id); } }
8、最后是spring的启动类MybatisApplication
package com.shuimutong.learn.mybatis; import org.mybatis.spring.annotation.MapperScan; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication @MapperScan("com.shuimutong.learn.mybatis.dao") //这个注解从运行结果看:加不加都行 //如果不加,只扫描和主类处于同包下的Class https://blog.csdn.net/lisheng19870305/article/details/102816358 public class MybatisApplication { public static void main(String[] args) { SpringApplication.run(MybatisApplication.class, args); } }
关于MapperScan注解,我在项目里试了,不加也能运行。具体说明参考对应的链接文章。
9、启动项目请求连接
http://localhost:8080/getUser?id=1
{"id":1,"name":"trans-634541697","age":6}
最后,git地址:https://github.com/shuimutong/spring_learn/tree/master/mybatis(项目较多,需要点开后才能clone)
祝大家生活开心、工作顺利。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK