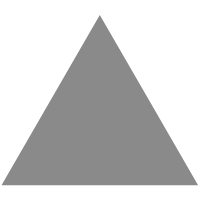
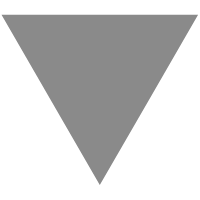
使用Java代码配置MyBatis Generator
source link: http://www.cnblogs.com/xuruiming/p/13124609.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
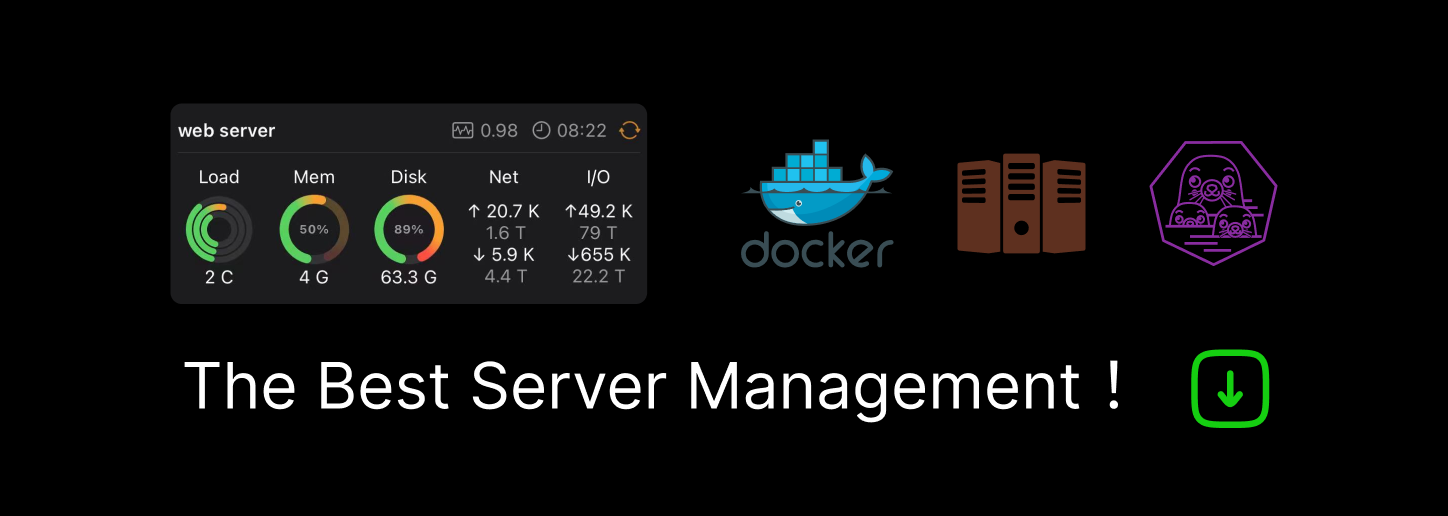
使用MyBatis Generator生成器时,有时候没办法使用xml型的配置文件,比如将Maven项目设置成pom打包方式(<packaging> pom </packaging>)!由于Maven的工作机制对于打包方式为pom的项目是不会输出jar包或war和resources内容,所以放在resources目录下或放在源码目录下的xml文件就没法读取了,就算你在pom.xml文件中明确有如下配置也没有用的:
<build> <resources> <resource> <directory>src/main/java</directory> <includes> <include>**/*.properties</include> <include>**/*.yml</include> <include>**/*.xml</include> </includes> </resource> <resource> <directory>src/main/resources</directory> <includes> <include>**/*.properties</include> <include>**/*.yml</include> <include>**/*.xml</include> </includes> </resource> </resources> </build>
这个时候就会用到纯Java代码的MyBatis Generator配置,直接贴配置代码:
import org.mybatis.generator.config.*; /** * 基础Java代码的MBG配置 * Maven打包方式为POM的项目或模块(<packaging>pom</packaging>),resources目录的内容不会输出到类路径下,所以可以选择直接使用Java代码配置! * * @author [email protected] * @since 2020-06-13 */ public class GeneratorConfig { public static Configuration getGeneratorConfig() { Context context = new Context(ModelType.CONDITIONAL); context.setId("simple"); context.setTargetRuntime("MyBatis3Simple"); /*添加属性*/ context.addProperty("javaFileEncoding", "UTF-8"); /*插件配置,这个是我自己的插件,没有自定义插件的同学可以不配这一节,删除即可*/ PluginConfiguration pluginConfig = new PluginConfiguration(); pluginConfig.setConfigurationType("com.xgclassroom.generator.GeneratorPlugin"); context.addPluginConfiguration(pluginConfig); /*注释生成器配置*/ CommentGeneratorConfiguration commentGeneratorConfig = new CommentGeneratorConfiguration(); commentGeneratorConfig.addProperty("suppressAllComments", "true"); context.setCommentGeneratorConfiguration(commentGeneratorConfig); /*JDBC连接信息配置*/ JDBCConnectionConfiguration jdbcConnectionConfig = new JDBCConnectionConfiguration(); jdbcConnectionConfig.setDriverClass("com.mysql.cj.jdbc.Driver"); //注意代码配置中JDBC连接字符串中的参数分隔符不需要再像xml配置文件中那样使用转义符 jdbcConnectionConfig.setConnectionURL("jdbc:mysql://localhost:3306/permission_center?useUnicode=true&characterEncoding=utf8&serverTimezone=GMT%2B8&useSSL=false"); jdbcConnectionConfig.setUserId("xurm"); jdbcConnectionConfig.setPassword("1qaz@WSX"); jdbcConnectionConfig.addProperty("nullCatalogMeansCurrent", "true");//MySQL无法识别table标签中schema类的配置,所以在URL上指明目标数据库,并追加nullCatalogMeansCurrent属性为true jdbcConnectionConfig.addProperty("remarksReporting", "true");//针对oracle数据库无法读取表和字段备注 jdbcConnectionConfig.addProperty("useInformationSchema", "true");//针对mysql数据库无法读取表和字段备注 context.setJdbcConnectionConfiguration(jdbcConnectionConfig); /*Model生成器配置*/ JavaModelGeneratorConfiguration javaModelGeneratorConfig = new JavaModelGeneratorConfiguration(); javaModelGeneratorConfig.setTargetProject("permission/src/main/java");//目标项目(源码主路径) javaModelGeneratorConfig.setTargetPackage("com.xgclassroom.model");//目标包(Model类文件存放包) context.setJavaModelGeneratorConfiguration(javaModelGeneratorConfig); /*SqlMapper生成器配置(*Mapper.xml类文件),要javaClient生成器类型配合*/ SqlMapGeneratorConfiguration sqlMapGeneratorConfig = new SqlMapGeneratorConfiguration(); sqlMapGeneratorConfig.setTargetProject("permission/src/main/java");//目标项目(源码主路径) sqlMapGeneratorConfig.setTargetPackage("com.xgclassroom.mapper");//目标包(*Mapper.xml类文件存放包) context.setSqlMapGeneratorConfiguration(sqlMapGeneratorConfig); /*JavaClient生成器配置(*Mapper.java类文件)*/ JavaClientGeneratorConfiguration javaClientGeneratorConfig = new JavaClientGeneratorConfiguration(); javaClientGeneratorConfig.setConfigurationType("XMLMAPPER");//JavaClient生成器类型(主要有ANNOTATEDMAPPER、MIXEDMAPPER、XMLMAPPER,要Context的TargetRuntime配合) javaClientGeneratorConfig.setTargetProject("permission/src/main/java");//目标项目(源码主路径) javaClientGeneratorConfig.setTargetPackage("com.xgclassroom.mapper");//目标包(*Mapper.java类文件存放包) context.setJavaClientGeneratorConfiguration(javaClientGeneratorConfig); /*表生成配置*/ TableConfiguration tableConfig = new TableConfiguration(context); tableConfig.setTableName("%"); GeneratedKey generatedKey = new GeneratedKey("id", "JDBC", true, null);//设置主键列和生成方式 tableConfig.setGeneratedKey(generatedKey); context.addTableConfiguration(tableConfig); Configuration config = new Configuration(); config.addContext(context); return config; } }
然后就是把MyBatis Generator调用过程中原本读取xml配置文件的地方换掉就可以了:
import org.mybatis.generator.api.MyBatisGenerator; import org.mybatis.generator.config.Configuration; import org.mybatis.generator.internal.DefaultShellCallback; import java.util.ArrayList; import java.util.List; /** * MyBatisGenerator代码生成器Java调用程序 * * @author [email protected] * @since 2020-06-13 */ public class GeneratorRunner { public static void main(String[] args) { try { List<String> warnings = new ArrayList<String>(); Configuration config; //使用xml配置文件的方式 /*File configFile = new File(GeneratorRunner.class.getClassLoader().getResource("generatorConfig.xml").getPath()); ConfigurationParser cp = new ConfigurationParser(warnings); config = cp.parseConfiguration(configFile);*/ //使用纯Java代码配置的方式 config = GeneratorConfig.getGeneratorConfig(); DefaultShellCallback callback = new DefaultShellCallback(true); MyBatisGenerator myBatisGenerator = new MyBatisGenerator(config, callback, warnings); myBatisGenerator.generate(null); } catch (Exception e) { System.out.println(e.getMessage()); e.printStackTrace(); } } }
如果对你有帮助,请记得点个【推荐】!
Talk is cheap, show me the code! Bye.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK