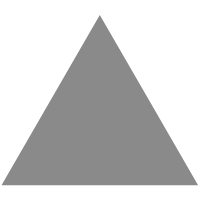
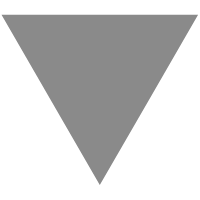
Face Detection in just 5 lines of code
source link: https://towardsdatascience.com/face-detection-in-just-5-lines-of-code-5cc6087cb1a9?gi=890d6c17762
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
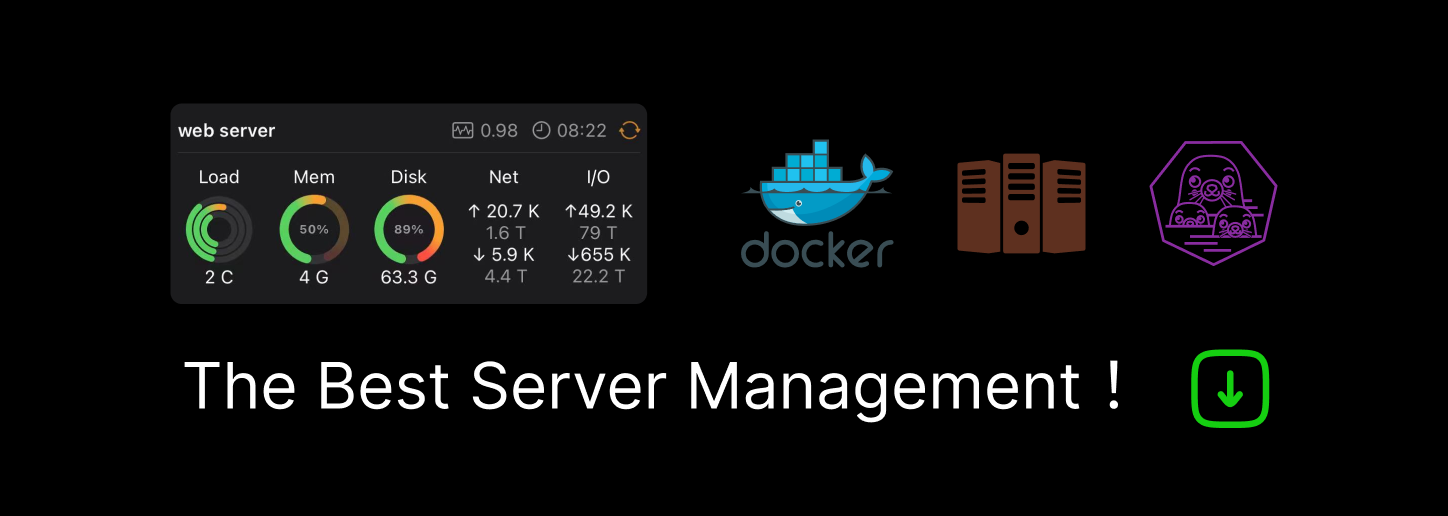
Face Detection in just 5 lines of code
Recognize and Manipulate faces with the world’s simplest face recognition python library.

Jun 13 ·5min read
F ace detection is a computer technology part of deep learning. It identifies human faces in digital images or video scenes. It is used in a variety of applications such as Facial Recognition, Unlock smartphones, Smart advertising, Emotional inference , and many more.
Deep learningis a subset of machine learning in artificial intelligence (AI) that has networks capable of learning unsupervised from data that is unstructured or unlabeled.
“COMPUTERS ARE NOW ABLE TO SEE, HEAR AND LEARN. WELCOME TO THE FUTURE!“ — Dave Waters
In this beginner’s tutorial, we are going to learn how to install and configure python environment, use Integrated Development Environments (IDEs) to debug and run our python code. Then we will use widely popular face detection and manipulation library face-recognition by Adam Geitgey . We will customize the images with different colors and border shapes around the detected faces . It is built using dlib ’s state-of-the-art face recognition with deep learning. The model has an accuracy of 99.38% on the Labeled Faces in the Wild benchmark.
TL;DRThis tutorial is for absolute beginners , even if you are a fresh graduate or someone looking forward to starting machine learning using python. Developers across the technology stack like Web and Mobile developers or Backend developers can also follow this article with ease. So, Let’s begin our new brief code journey.
Environment Configuration and Installation
At first, we need to download the latest stable version of Python .
There are usually 2 famous ways to configure python environments and install packages in our computers — pip or condo.
A python package contains all the files you need for a module. Modules are Python code libraries you can include in your project.
- PIP - a package manager for Python packages.
- Anaconda - a complete python environment solution to run different versions and manage all python packages. Using Anaconda Navigator, you can install and manage all packages in your computers.
You can follow anyone or both among these.
Code Editor and IDE
Apart from basic python REPL (command-line interface), we can run our python program in any of these two professional editors-
- PyCharm - Download popular Python IDE for Professional Developers and start. It’s like other famous IDE out there and simple to start and use.
- Jupyter Notebooks - Widely used web-based code editor by programmers and professional trainers.
To install Jupyter Notebook using conda-
conda install -c conda-forge notebook
or install using pip and then run Jupiter notebook to run it-
pip install notebook jupyter notebook
That's enough for us to start writing our first Python program and run it. You can use any one of the above editors. We are going to use PyCharm to write code with the pip package manager to install packages in our tutorial.
So, let’s commence detecting faces in any given images and photos. Ain't you excited?:grinning:
Firstly, let’s install the required libraries for our code to work. We will install and import only 2 libraries.
- Pillow - The friendly PIL fork (Python Imaging Library).
- Face_recognition - To detect faces in the image.
pip install Pillowpip install face-recognition
You can create a new file of type python (with the extension .py ) or a new notebook in Jupyter Notebooks to start coding — face_detection.py . In case, it requires other dependent libraries, you can simply install them too using pip or conda.
Let’s import above libraries in our code -
import PIL.Image
import PIL.ImageDraw
import face_recognition
Line 1
Let’s pick one image name GroupYoga.jpg (you can pick any image) in the same folder as our code file or else give the proper path of the image. We should load our image to the load_image_file() method from the face_recognition library which will convert it into a NumPy array of that image. Let’s assign it to the variable name given_image .
given_image = face_recognition.load_image_file('GroupYoga.jpg')
Line 2
Using face_locations() method from the same library, we will count the number of faces in the given_image and will print the length of total faces found in the image.
face_locations = face_recognition.face_locations(given_image)
number_of_faces = len(face_locations)
print("We found {} face(s) in this image.".format(number_of_faces))
Line 3
Now, to draw any shape on the image, we will convert the image to the Pillow library object using fromarray() method from PIL.Image.
pil_image = PIL.Image.fromarray(given_image)
Line 4
Now, we will run a for-in loop to print four pixel locations such as top, left, bottom & right of the detected faces.
for face_location in face_locations:
top, left, bottom, right = face_location
print("A face is detected at pixel location Top: {}, Left: {}, Bottom: {}, Right: {}".format(top, left, bottom, right))
Line 5
We will draw a green color rectangle box with width 10 around the faces. You can change the shape to polygon or ellipse also. So, in the same above loop write below 2 draw methods.
draw = PIL.ImageDraw.Draw(pil_image)
draw.rectangle([left, top, right, bottom], outline="green", width=10)
Now, just use the variable pil_image to display our new image with detected faces using a rectangular border around them.
pil_image.show()
The new temporary image will be open automatically on your computer (if everything is set up correctly). It should be something like below image -
Bingo! :tada:
In case, you get stuck with any compile time or run time issues and environment configuration issues, you can write in the response section below or connect with me.
Now, let’s recollect all the above small code snippets into one file conjointly which is ready to run in any python environment. Let’s use the school kids image(as featured in the cover of the article)-
If you run the above file(run button in PyCharm or Notebook), if everything goes fine (I mean compile or runtime errors), you are going to get the below output at the console of your editor -
That’s it. Our simplest face detection :performing_arts: code is ready to recognize faces from any given photos. You can customize the above code by changing colors, border types, and width. I recommend to try customization and explore other methods and options.
Face_recognitionlibrary can also be used to put digital makeup on the faces, check the similar faces among a group of people and develop some games around these detected faces.
I am sure you will find it as one of the simplest face detection tutorial among all the tech posts available on the internet. you can go through the below resources for more details of this library. For other applications of this library, I shall write some other articles next time.
Resources:
Follow me on Medium and let’s be connected on LinkedIn ( @kapilraghuwansh i) and Twitter ( @techygeeek y) for more such interesting tech articles.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK