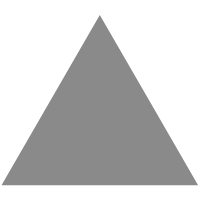
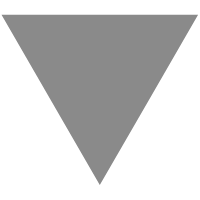
Get Deeper Look into Looping Concept with Python
source link: https://mc.ai/get-deeper-look-into-looping-concept-with-python/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
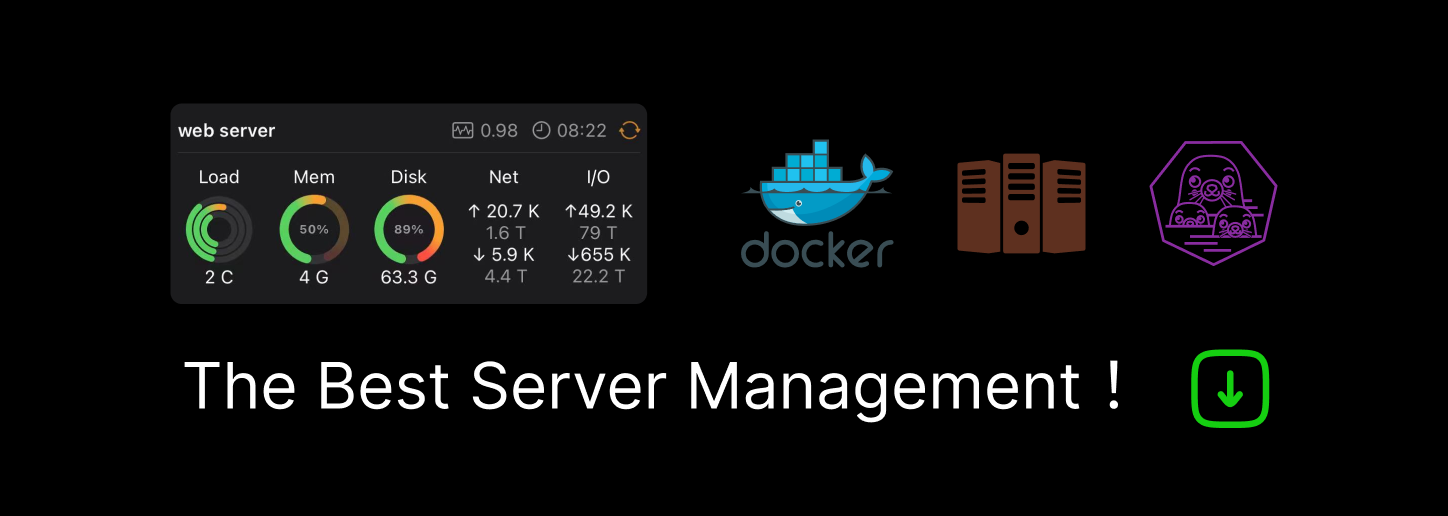
Repeating the instruction in a sequence is an important concept used by a programmer to reduce the repetition of the same code for multiple times. The concept of looping makes a simple way to perform a certain task in a program for multiple times. As I always saying, we have to look at the nature to grasp the logic for any kind of problem solving through computer programs. In nature we are surrounded by many kinds of repeated tasks. From the revolution of earth around the sun to synodic cycles of moon, they follows some same kind of activities in a loop. In programming, it is essential to have such a concept to avoid the complexity of code. In this article you will learn about the creation of loops in Python using different ways.
Why need a loop ?
Let us try to understand the concept with some logical scenarios. Consider that you are working as a professor in a college. At the very first day of the college you have an introductory session in a class. At beginning the no of students in the class is three. You should ask for their name one by one. In programming perspective the equivalent code for this scenario will be like the following snippet.
student_1 = input("Your Name?") student_2 = input("Your Name?") student_3 = input("Your Name?")
After some more time the count of the students in that class get increased by 100. Now you are going to ask the same question to the all students. But writing the scenario as a computer program in the above format will not be a best solution as the program will have lots of lines of code. Looping made this very simple. In programming, you have to write the instruction for only one time which can be executed for multiple times.
Executing the same instructions for multiple time is great. But we have to stop the execution somewhere. The logic for the above discussed scenario will be something like this.
LOOP UNTIL MEET LAST PERSON { student_id = input("Your Name?") }
Here meeting the last student in the classroom is a condition that drives the loop perfectly. Each loop should follow some conditions to stop somewhere when the condition is failed to meet. We will discuss what will happen when there is no condition available in the loop later.
“Repetition of practice makes the master”
Basic loops in Python
The looping statements in python can be classified into two major statements. The statements are for and while loops. Both of them has their uniqueness towards their working. We will discuss about the for loop for now.
The for loop
The for loop is a loop which mostly runs based on the collection of data provided to it. The loop will stop at somewhere at which the value of corresponding data in a collection becomes false. It is a very useful type of tool in which we have a clear idea about how many times the loop should be executed. The syntax for the for loop is given below.
Syntax: for value in sequence:
Here the sequence is a collection of data which drives the for loop from the start to end. The for loop will check for the stating value of any collection of data and store the value to the looping variable. In the syntax the term ‘value’ is called looping variable. After successful execution of all the instructions under the loop for first time. The next value of the collection will stored in the value then the instructions will be executed again. This cycle happens continuously when there is no element available in the collection. The basic collection data types used in for loop is listed below.
Range function
List data type
Tuple data type
Dictionary data type
Set data type
String data type
Let us discuss about each collective data with some examples using for loop statement.
Range function
Range is a function which is used to generate a numerical sequence of data with continuous values. The range function can be used in three different ways based on the arguments passed through it.
range(end) range(start,end) range(start,end,step)
range(end)
This function will generate the values from zero to one lesser than the passed value. If the argument passed is 5 then it will generate 0,1,2,3,4. Look at the following code.
for value in range(8): print(value,end=" ")
Output
range(start,end)
It will generate a continuous numbers in sequence from a particular start instead of zero. The list will start from the first argument to second – 1.
for value in range(3,9): print(value,end=" ")
Output
range(start,end,step)
This function will generate the numbers with a particular gap between them. If the first value is start then the next value will be start+step.
for value in range(2,20,2): print(value,end=" ")
Output
List data type
A python list can be used to run a for loop. The looping variable will take the value of current position of the list as its value. Consider a list list = [0,1,2,3]. The for loop can be created like the following.
for value in list: print(value)
Output
Tuple data type
Tuple data types works in the same manner as the list in python. The for loop created using a tuple is given below.
tuple = (1,2,3,4,5) for value in tuple: print(value,end=" ")
Output
String data type
String data type will create a loop with iterations equal to the number of characters present in the string. Each character is stored in the looping value one by one.
string = "Hello World" for x in string: print(x)
Output
H e l l oW o r l d
Do the same things for dictionary and set data types.
Count vs Value
Make some more exercises with all the data types. You can see that the two collections of same length will produce the iterations equal to the same length. So the major purpose of for loop is to create a loop with certain number of iterations.
Then, why a Variable?
You may wonder why we are using a looping variable to generate iterations. The looping variables are mainly used to check whether the condition is true or not. But using the value inside the scope of the loop is an additional advantage provided by the language which gives higher flexibility over the logic. Let me try to explain this concept with two different examples. Consider a problem statement in which you have to print numbers between 2 to 6. We can do the thing with following code.
a = 2 for x in range(5): print(a,end=" ") a++
Output
The same thing will be done using the following.
for x in range(2,7): print(x,end=" ")
Output
As I mentioned earlier the above example shows that the looping variable provides an additional advantage to use the value itself instead of external variable for some tasks.
The while loop
While is an another looping concept which uses a condition to check whether the loop should run again or not. In most cases the variable used to check the condition will have dynamic value assignments. That means the value of the variable will change often.
Syntax: while condition:
If the condition is true the statements will executed. When the condition becomes false the loop will stop iterating. The while loop is used when the no of iterations are not defined.
a=5 while a!=0: print(a,end=" ") a--
Output
After five iterations the value of a will become 0. Which gives False for the expression a!=0
. So the loop will stop there.
Infinite loops
Infinite loops are created if the condition becomes true continuously. Look at the following code.
a=1 while True: print(a)
It will print the value for infinite times. To prevent your code from this kind of loops we can use two different kinds of keywords. The keywords are break
and continue
.
Break Statement
Break statement is used to stop the loop by giving a certain condition as statement in the program.
a=1 while(True): print("Hi") a++ if a==5: break
This will break the loop when the value of a become 5. The output will be.
Hi Hi Hi Hi Hi
Continue Statement
Continue statement is used to skip some particular iterations in the loop. Consider the following loop generated using range 1 to 10. To skip wherever the value is even we can use continue.
for x in range(1,10): if(x%2==0): continue print(x,end=" ")
Output
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK