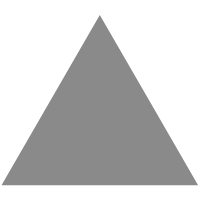
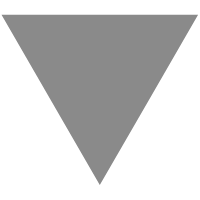
Formatting Strings with JavaScript padStart() and padEnd()
source link: https://scotch.io/tutorials/formattings-string-with-javascript-padstart-and-padend
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
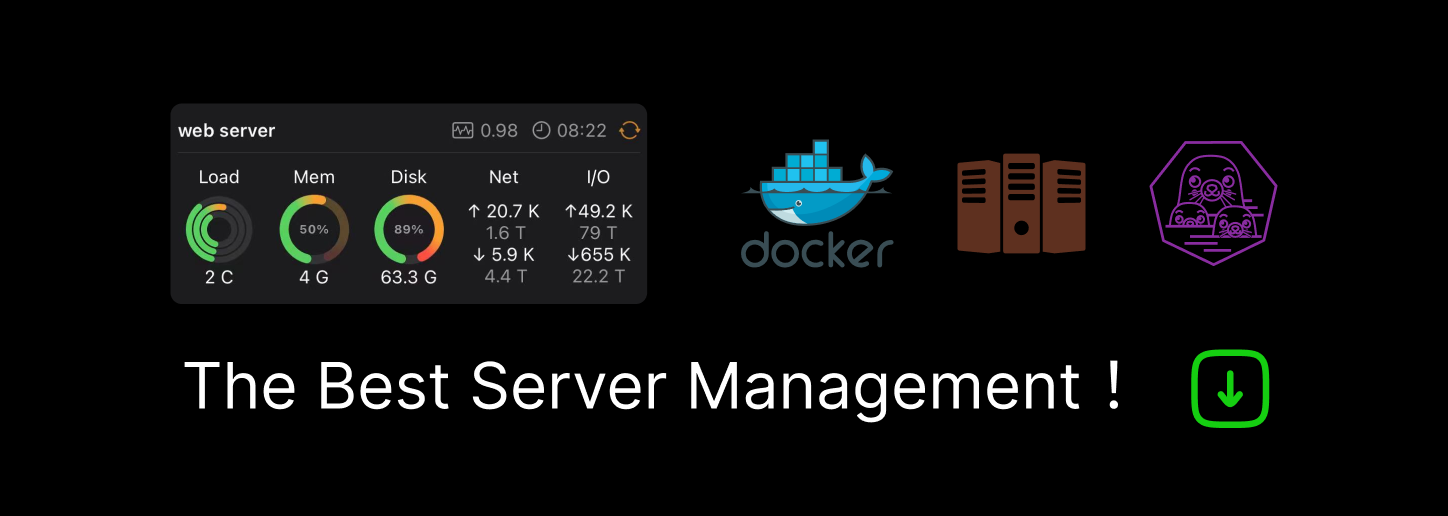
A few days ago, I was working on building a countdown timer in JavaScript, and I needed to format my seconds and milliseconds. I wanted the seconds to always be a length of 2 digits and the milliseconds to always be a length of 3 digits. In other words I wanted 1
second to display as 01
and 1
millisecond to display as 001
.
Table of Contents
I ended up writing my own function to "pad" these numbers, but I found out there are built in functions in JavaScript, padStart() and padEnd() to do just that. In this article, let's look at how to leverage these built-in functions in JavaScript!
Let's start by covering a few different use cases for padding.
Labels and Values
Let's say you have labels and values on the same line, something like Name: James
and Phone Number: (555)-555-1234
. If you stack these lines of different lengths on top of each other, it looks a bit odd. So instead of this...
Name: James Phone Number: (555)-555-1234
You might want this.
Name: James Phone Number: (555)555-1234
or this...
Name: James Phone Number: (555)555-1234
Money
In America, it's customary to display two digits for the cents when displaying price. So instead of this...
$10.1
You would want this.
Our New React Course! Make 20 React Apps$10.01
Dates
For dates, you want 2 digits for both the day and the month. So instead of this...
2020-5-4
You would want this.
2020-05-04
Timer
Similar to dates above, for a ms timer, you would want 2 digits for the seconds and 3 digits for the ms. So instead of this...
You would want this.
Let's start with padStart()
(get it?!?) and with the label and value example. Let's say we want our labels to be right aligned with each other so that the values start at the same place.
Name: James Phone Number: (555)555-1234
Since Phone Number
is the longer of the two labels we want to pad the start of the Name
label with spaces. For future sake though, let's not pad it specifically to the length of Phone Number,
let's pad it to something longer, say 20 characters. This way, if you ever use longer labels in the future this trick will still work.
Before we pad, here's our starter code for displaying this info.
const label1 = "Name"; const label2 = "Phone Number"; const name = "James" const phoneNumber = "(555)-555-1234"; console.log(label1 + ": " + name); console.log(label2 + ": " + phoneNumber); //Name: James //Phone Number: (555)-555-1234
Now, let's pad the first label. For calling padStart()
you need to pass two parameters: one for the target length of the padded string, and the second for the character you want to pad with
. In this case, we want the length to be 20, and the padded character to be a space. It will look like.
const label1 = "Name"; const label2 = "Phone Number"; const name = "James" const phoneNumber = "(555)-555-1234"; console.log(label1.padStart(20, " ") + ": " + name); console.log(label2 + ": " + phoneNumber); // Name: James ////Phone Number: (555)-555-1234
And now to pad the second line.
const label1 = "Name"; const label2 = "Phone Number"; const name = "James" const phoneNumber = "(555)-555-1234"; console.log(label1.padStart(20, " ") + ": " + name); console.log(label2.padStart(20, " ") + ": " + phoneNumber); // Name: James //// Phone Number: (555)-555-1234
With the same label and value example, let's change the way we pad the labels. Let's align the labels to the left so that we are adding padding at the end.
Starter code.
const label1 = "Name"; const label2 = "Phone Number"; const name = "James" const phoneNumber = "(555)-555-1234"; console.log(label1 + ": " + name); console.log(label2 + ": " + phoneNumber); //Name: James //Phone Number: (555)-555-1234
Now, let's pad the first label. Similar to what we did before with two small differences. Now, we are using padEnd()
instead of padStart()
and we need to concatenate the colon with the label before padding. This way we make sure the colon ends up in the right place.
const label1 = "Name"; const label2 = "Phone Number"; const name = "James" const phoneNumber = "(555)-555-1234"; console.log((label1 + ': ').padEnd(20, ' ') + name); console.log(label2 + ": " + phoneNumber); //Name: James //Phone Number: (555)-555-1234
And now with both lines padded.
const label1 = "Name"; const label2 = "Phone Number"; const name = "James" const phoneNumber = "(555)-555-1234"; console.log((label1 + ': ').padEnd(20, ' ') + name); console.log((label2 + ': ').padEnd(20, ' ') + phoneNumber); //Name: James //Phone Number: (555)-555-1234
What About Numbers (prices, dates, timers,etc)
We haven't specified this yet (although it may be obvious), but the padding functions are specifically associated with strings
not numbers.
So, to pad a number, we need to first convert it to a string.
Price
Let's look at the starter code for displaying a price.
const dollars = 10; const cents = 1; console.log("$" + dollars + "." + cents); //$10.1
To pad our cents, we need to first convert it to a string, then call the padStart()
function specifying a length of 1 and a pad character of '0';
const dollars = 10; const cents = 1; console.log("$" + dollars + "." + cents.toString().padStart(2,0)); //$10.01
Dates
Here's the starter code for a displaying a date.
const month = 2; const year = 2020; console.log(year + "-" + month); //2020-2
Now, let's pad the month to make sure it's two digits.
const month = 2; const year = 2020; console.log(year + "-" + month.toString().padStart(2,"0")); // 2020-02
Timer
And finally our timer. We have two different numbers we want to format, the seconds and the milliseconds. Same principle applies though. Here's the starter code.
const seconds = 1; const ms = 1; console.log(seconds + ":" + ms); //1:1
And now to pad. I'm going to do the padding on separate lines so that it is easier to read.
const seconds = 1; const formattedSeconds = seconds.toString().padStart(2,0); const ms = 1; const formattedMs = ms.toString().padStart(3,0); console.log(formattedSeconds + ":" + formattedMs); //01:001
Although writing your own padding function wouldn't be difficult, why do it yourself when it's built in to JavaScript? There's lots of nifty functions that are already built in. It's probably worth doing a quick search before you build something yourself.
Question for you. What are other useful functions in JavaScript that not many people know about? Let me know in the comments below or on twitter, @jamesqquick .
Like this article? Follow @jamesqquick on Twitter
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK