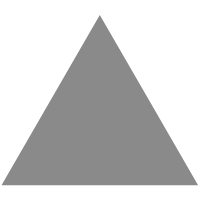
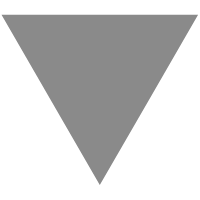
Kotlin’s Elvis better than Swift’s Guard - Elye - Medium
source link: https://medium.com/@elye.project/kotlins-elvis-better-than-swift-s-guard-53030d403c3f?source=friends_link&%3Bsk=bf7fced446cc161095a9d281054fb927
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
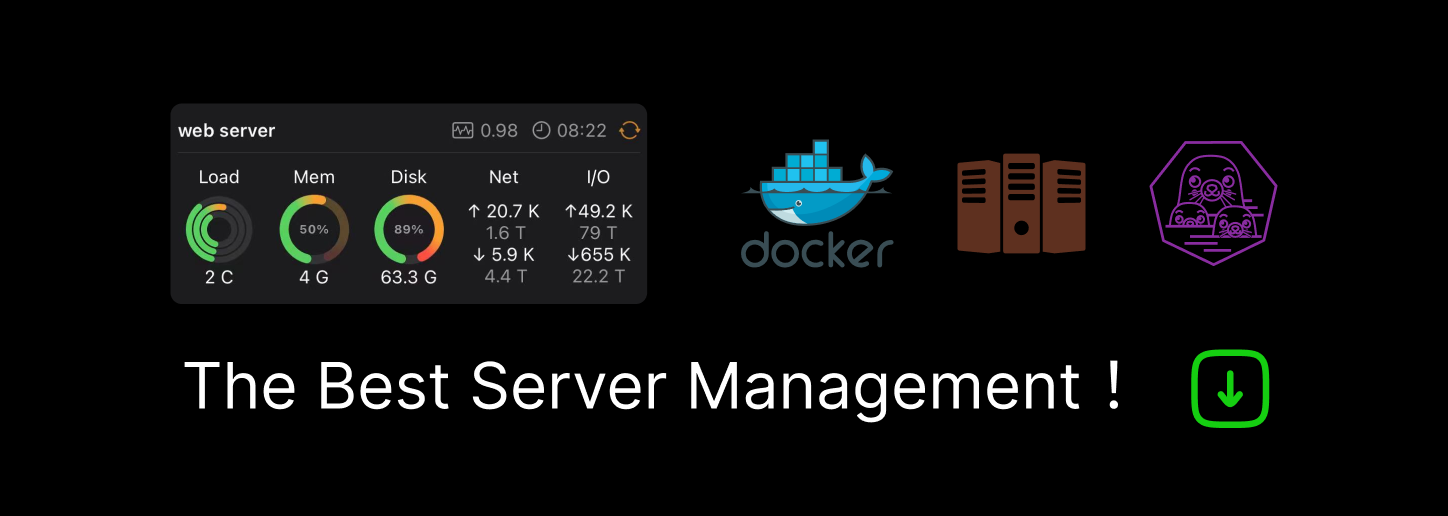
You have 2 free member-only stories left this month.
Why Kotlin’s Elvis Operator is Better Than Swift’s Guard Statement
It’s similar but better


If you’ve programmed for iOS Swift before, you’ll find this interesting keyword, guard
, that helps to guard and ensure some condition is met or some variable is not null before proceeding.
func process(couldBeNullMesage: String?) {
guard let notNullMessage = couldBeNullMesage else { return } printMessage(notNullMessage)
// ... and a lot more
// things to do...
}func printMessage(message: String) {
print(message)
}
Something like the above is common, where guard
is used to prevent any subsequent code from being executed if a null
value is received.
Coming from the Android and Kotlin world, I find guard
fascinating. I gave a quick think about what the equivalent in Kotlin might be.
Four Different Ways in Kotlin (Getting Better Each Time)
If you’re short on patience, you can go straight to the fourth approach — which amazes me.
The popular let
approach
Everybody who first learns Kotlin loves let
.
fun process(couldBeNullMesage: String?) {
couldBeNullMesage?.let {
printMessage(it)
// ... and a lot more
// things to do...
}
}fun printMessage(message: String) {
println(message)
}
This definitely does the work. However, all the code in the function will need to have an extra indentation.
As mentioned in this article, let
isn’t always a good option.
2. The Elvis-operator approach
In Kotlin, the Elvis operator is similar to Swift coalescing — but a little smarter. It can assign a non-null
value to another variable, and if it is null
, it can execute return
.
fun process(couldBeNullMesage: String?) { val notNullMessage = couldBeNullMesage ?: return printMessage(notNullMessage) // this is autocast to non-null.
// ... and a lot more
// things to do...
}fun printMessage(message: String) {
println(message)
}
This is almost like the guard
equivalent in the Swift language. It’s already better than guard
, as it doesn’t need a parenthesis to wrap the return
.
In Swift, we can’t do the following, as it expects a valid string on the right side of coalescing — i.e., ??
.
val notNullMessage = couldBeNullMesage ?? return
Nonetheless, I’m still not content with the Elvis-operator approach above. The conventional approach below looks even better.
3. Conventional if-else approach
Let’s use what the Java convention teaches us: the old, faithful if-else
.
fun process(couldBeNullMesage: String?) { if (couldBeNullMesage == null) return printMessage(couldBeNullMesage) // smartcast to non-null.
// ... and a lot more
// things to do...
}fun printMessage(message: String) {
println(message)
}
In Kotlin, the smart casting of a null
-checked variable to a non-null
variable eliminates the need for a temporary variable.
But wait! There’s an even better way.
4. The smart cast–Elvis operator approach
What if we combine the Elvis operator and the smart-cast capability of Kotlin together — how would it look like?
fun process(couldBeNullMesage: String?) { couldBeNullMesage ?: return printMessage(couldBeNullMesage) // smartcast to non-null.
// ... and a lot more
// things to do...
}fun printMessage(message: String) {
println(message)
}
Hallelujah! This is just genius. Concise and to the point.
A Quick Comparison
// Swift
guard let notNullMessage = couldBeNullMesage else { return }// Kotlin
couldBeNullMesage ?: return
Sorry if I started a battle between iOS and Android. Some credit should go to iOS, as states below:
- I have to thank iOS Swift for letting me ponder this by learning about
guard
- To be fair,
guard
isn’t just for the sole purpose of checking thenull
value. It’s used in other contexts (e.g., boolean variables, check for more variabless), which can’t be done with a single Kotlin Elvis operation.
It’s just in this context purely that, in my opinion, Kotlin does better.
Thanks for reading.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK