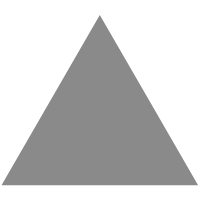
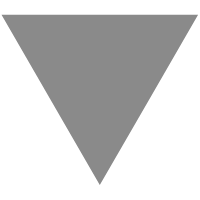
Replacing React Lifecycle Methods with Hooks: A Comprehensive Guide
source link: https://blog.bitsrc.io/replacing-react-lifecycle-methods-with-hooks-a-comprehensive-guide-dfd5cbe1f274
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
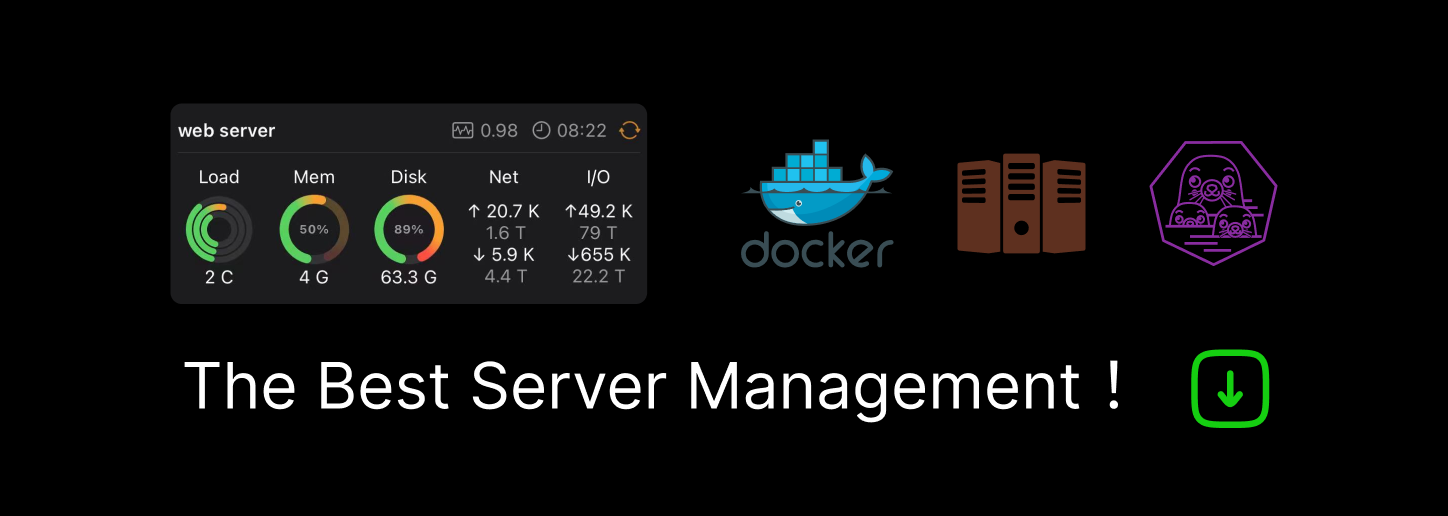
Using Hooks to replace componentDidMount, componentWillUnmount, componentWillReceiveProps, componentDidUpdate.
May 4 ·4min read
Why Hooks? / Why not Classes?
Class components are verbose and cumbersome. In many cases, we are forced to duplicate our logic in different lifecycle methods to implement an ‘effect logic’.
Class components do not offer an elegant solution to sharing logic between components (HOC and friends are not an elegant solution) — React Hooks, on the other hand, give us the ability to build custom hooks, a much simpler solution.
And the topping of the cake — we’ve had enough dealing with this
.
Sharing Components: Classes vs Functions
We share and reuse components with our team through cloud component hubs like Bit.dev . The logic of that is pretty simple: we maximize code reuse, keep a consistent UI and collaborate across repositories.
To make the most out of tools like Bit.dev and guarantee we make it easy and simple to reuse and maintain other’s components, it’s best to go for function components with hooks. They’re simply more ‘lightweight’, elegant, and easy to understand. They are, IMHO, what components should have been all along.

componentDidMount
componentDidMount
's equivalent in function components is the useEffect
hook with an empty array as its second argument.
For instance, we can use componentDidMount
to load some data on component load as follows:
(The component is at https://bit.dev/jauyeunggithub/componentdidmount )
We loaded some data from an API with the componentDidMount
hook, which is rendered in the render
method.
To do the same thing with the useEffect
hook in function components, we write:
We have the fetchData
function to get the data.
Then we call it in the useEffect
callback.
We passed in an empty array to useEffect
as the 2nd argument so that the callback only runs on component's first load.
We can’t have async functions as a callback. Otherwise, we’ll get an error.
componentWillUnmount
componentWillUnmount
is a lifecycle method for class-based components that's loaded when the component is being removed from React virtual DOM.
We can run some code when the component unmounts by writing:
We call clearInterval
to remove the timer in the componentWillUnmount
hook so that we remove the timer when the component unmounts.
To do the same thing with hooks, we can use the useEffect
hook again.
We return a callback that runs code before the component unloads.
To function component of the example above is:
We have the date
and timer
states to hold the current date and the timer object respectively.
The timer is created by setInterval
when the component loads as indicated by the empty array in the 2nd argument of useEffect
.
In the callback, we passed into useEffect
, we return a function that runs cleanup code.
Therefore, we have clearInterval
in that function to remove the timer from when the component unmounts.
componentWillReceiveProps / componentDidUpdate
The useEffect
hook is also the equivalent of the componentWillReceiveProps
or componentDidUpdate
hooks.
All we have to do is to pass in an array with the value that we want to watch for changes.
For instance, the following code has a SuperCounter
component with the componentDidUpdate
hook:
componentDidUpdate
takes a prevProps
parameter.
We check if this.props.count
is different from prevProps.count
.
If it is, then we update the superCount
state with this.props.count * 2
.
We can write the hooks equivalent as follows:
We also have a SuperCounter
component that has the destructed count
prop in the parameter.
Then in the useEffect
hook, we called setSuperCount
in the callback.
The 2nd argument is an array with the count
variable.
This means that we’re watching for changes in the value of the count
variable.
If the value changes, the callback runs.
As we can see, this is much simpler than the old componentDidUpdate
life cycle method.
Bonus: DOM refs
To access DOM elements, we need to use refs.
In a class component, we can create a ref and access it by writing:
We set the this.input
in a callback that we pass into the ref
prop of the input element.
Then we can call DOM methods on inputRef
as we did in the onClick
callback.
To do the same thing with function components, we use the useRef
hook:
All we had to do is call the useRef
hook to return a ref object.
Then we can call it in our onClick
handler as we did in the button element.
The difference is that the DOM methods are under the current
property instead of being at the top level.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK