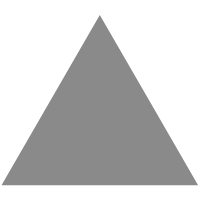
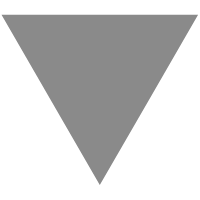
Blazor WebAssembly Rest Client
source link: https://christianfindlay.com/2020/02/14/blazor-webassembly-rest-client/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
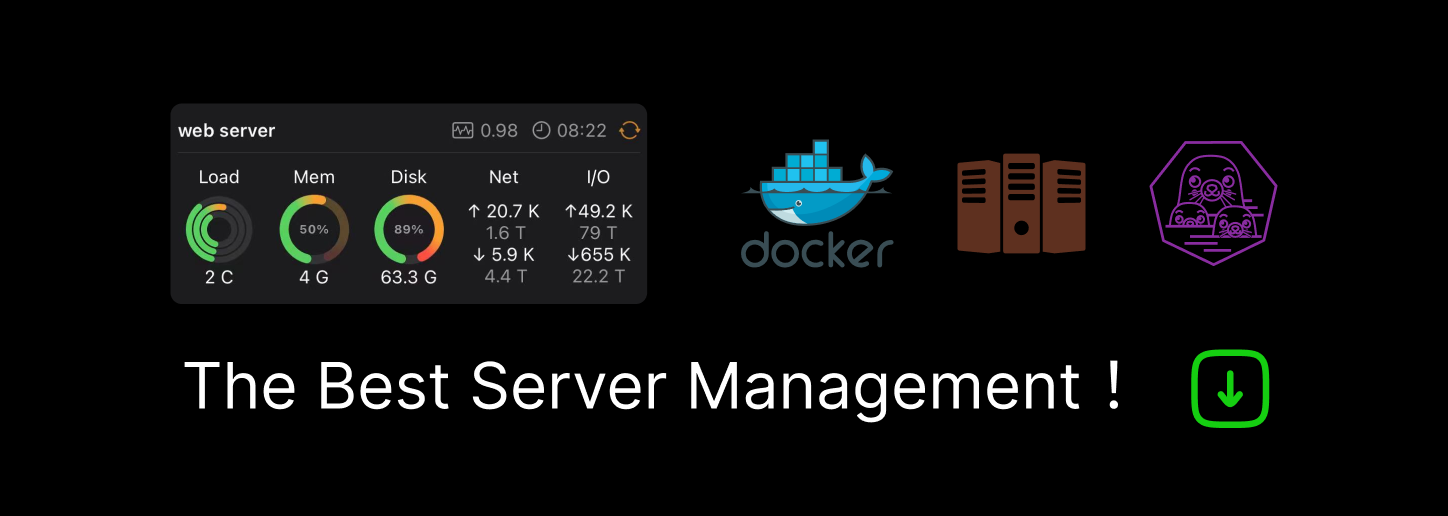
Blazor is Microsoft’s latest Single Page Application (SPA) framework, which is C# based and renders to the browser HTML DOM. Blazor comes in two flavors: server-side and client-side rendering. This article focuses on client-side rendering and explains how to use RestClient.Net to make calls to a RESTful API. Blazor WebAssembly uses C# compiled for WebAssembly (Wasm).
Blazor lets you build interactive web UIs using C# instead of JavaScript. Blazor apps are composed of reusable web UI components implemented using C#, HTML, and CSS. Both client and server code is written in C#, allowing you to share code and libraries.
https://dotnet.microsoft.com/apps/aspnet/web-apps/blazorIf you haven’t heard of Blazor yet, now would be a good time to start doing some research. Front-end development has been primarily dominated by JavaScript and related technologies like TypeScript for a long time. C# developers often need to switch between JavaScript and C#, even though working in a single language can provide significant benefits for software development. Blazor offers an opportunity to write browser-based applications that are written purely in C#.
I previously wrote about using RestClient.Net on Uno Platform. Uno is another Wasm based technology that allows developers to build C# apps for browsers. Uno provides developers with a XAML based platform that is familiar to Windows desktop developers. Blazor allows a mixture of HTML and C# in a single page, so it is more similar to ASP.NET Core Razor scripting. It offers a pathway to migrate away from MVC apps.
The RestClient.Net NuGet package can be added to any server-side or client-side Blazor app. The Client class can be used directly on Blazor razor pages like so:
page "/countrydata" @using BlazorApp1.Data @using RestClient.Net; <h1>Countries</h1> <p>This page shows data about countries.</p> @if (countries == null) { <p><em>Loading...</em></p> } else { <table class="table"> <thead> <tr> <th>Name</th> <th>Flag</th> <th>Capital</th> <th>Borders</th> <th>Population</th> </tr> </thead> <tbody> @foreach (var country in countries) { <tr> <td>@country.name</td> <td><img src="@country.flag" width="320" height="200" /></td> <td>@country.capital</td> <td>@string.Join(", ", country.borders)</td> <td>@country.population</td> </tr> } </tbody> </table> } @code { private List<RestCountry> countries; protected override async Task OnInitializedAsync() { countries = await new Client(new NewtonsoftSerializationAdapter(), baseUri: new Uri("https://restcountries.eu/rest/v2/")).GetAsync<List<RestCountry>>(); } }
The easiest way to get started is to clone the repo and then check out this project. Open the solution RestClient.Net.Samples.sln in the latest version of Visual Studio 2019. The project is configured for client-side rendering on WebAssembly. It targets .NET Standard 2.0. Debugging is not available in this mode. To switch to server-side rendering, change the target framework to .NET Core 3.1. You can do this by editing the .csproj file directly.
<Project Sdk="Microsoft.NET.Sdk.Web"> <PropertyGroup> <TargetFramework>netstandard2.1</TargetFramework> <RootNamespace>BlazorApp1</RootNamespace> </PropertyGroup> <PropertyGroup Condition=" '$(TargetFramework)'!='netcoreapp3.1' "> <RazorLangVersion>3.0</RazorLangVersion> </PropertyGroup> <ItemGroup Condition=" '$(TargetFramework)'!='netcoreapp3.1' "> <Compile Remove="Startup.cs" /> <Content Remove="Pages\_Host.cshtml" /> <PackageReference Include="Microsoft.AspNetCore.Blazor" Version="3.2.0-preview1.20073.1" /> <PackageReference Include="Microsoft.AspNetCore.Blazor.Build" Version="3.2.0-preview1.20073.1" PrivateAssets="all" /> <PackageReference Include="Microsoft.AspNetCore.Blazor.DevServer" Version="3.2.0-preview1.20073.1" PrivateAssets="all" /> <PackageReference Include="Microsoft.AspNetCore.Blazor.HttpClient" Version="3.2.0-preview1.20073.1" /> </ItemGroup> <ItemGroup> <Compile Include="..\RestClient.Net.Samples.Uno\RestClient.Net.Samples.Uno.Shared\NewtonsoftSerializationAdapter.cs" Link="NewtonsoftSerializationAdapter.cs" /> <PackageReference Include="Newtonsoft.Json" Version="12.0.3" /> <ProjectReference Include="..\RestClient.Net\RestClient.Net.csproj" /> </ItemGroup> </Project>
The RestClient.Net page has documentation for different use cases. It can be used with JSON, and also Protobuffer. If you face any issues with RestClient.Net on Blazor, feel free to reach out on the issues section.
Wrap Up
Check out Blazor and Uno Platform. These platforms both offer new approaches to building web apps and provide familiar territory for .NET developers. Consuming RESTful Apis on Blazor is straight forward with RestClient.Net, so try it out with your next SPA project!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK