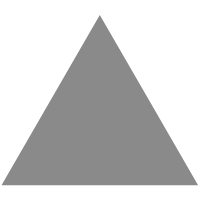
39
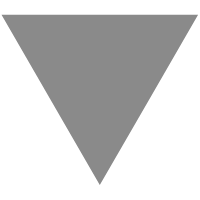
设计模式-装饰器模式
source link: http://www.hi-roy.com/2020/01/02/设计模式-装饰器模式/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
在python中有一个非常常用的语法糖 @
,本质上就是装饰器。这种模式可以不改变原文件的情况下动态的扩展一个对象的功能而不用创建子类,避免了类膨胀,是一种比较常用的模式,有个细节需要注意的就是装饰的顺序。
这个模式核心有4个角色:
- 被装饰者抽象Component:一个接口或者抽象类,定义最核心对象。
- 被装饰者具体实现ConcreteComponent:Component的实现类,装饰的就是这个实现类。
- 装饰者抽象Decorator:一般是个抽象类,实现接口或者抽象方法,里面必须有一个Component变量引用。
- 装饰者实现ConcreteDecorator:具体的装饰者类。
当只有一个被装饰者时,也可以吧Component和ConcreateComponent合并成一个,灵活运用而不要死板。
示例代码如下:
package main import ( "fmt" ) type Component interface { show() } type ConcreteComponent struct { name string } func (c ConcreteComponent) show() { fmt.Printf("This is %s\n", c.name) } type Decorator struct { c Component } func (d *Decorator) show() { d.c.show() } func (d *Decorator) setComponent(com Component) { d.c = com } type ConcreteDecorator struct { d Decorator age int } func (c *ConcreteDecorator) show() { fmt.Printf("In ConcreteDecorator show func! im %d old!\n", c.age) c.d.show() } func (c *ConcreteDecorator) setComponent(com Component) { c.d.setComponent(com) } func main() { c := ConcreteComponent{name: "tom"} cd := ConcreteDecorator{age: 10} cd.setComponent(c) cd.show() }
程序输出:
In ConcreteDecorator show func! im 10 old! This is concrete_component
使用场景
- 当需要动态的对某个对象添加功能时。
- 当某个对象的功能经常发生变化时,又想避免过多的继承造成类膨胀。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK