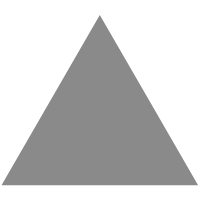
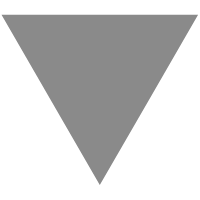
彻底解决Spring mvc中时间类型的转换和序列化问题
source link: http://mp.weixin.qq.com/s?__biz=Mzg3MjA4MTExMw%3D%3D&%3Bmid=2247487403&%3Bidx=2&%3Bsn=d3a4b4fd9a05a1b338970828129049b1
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
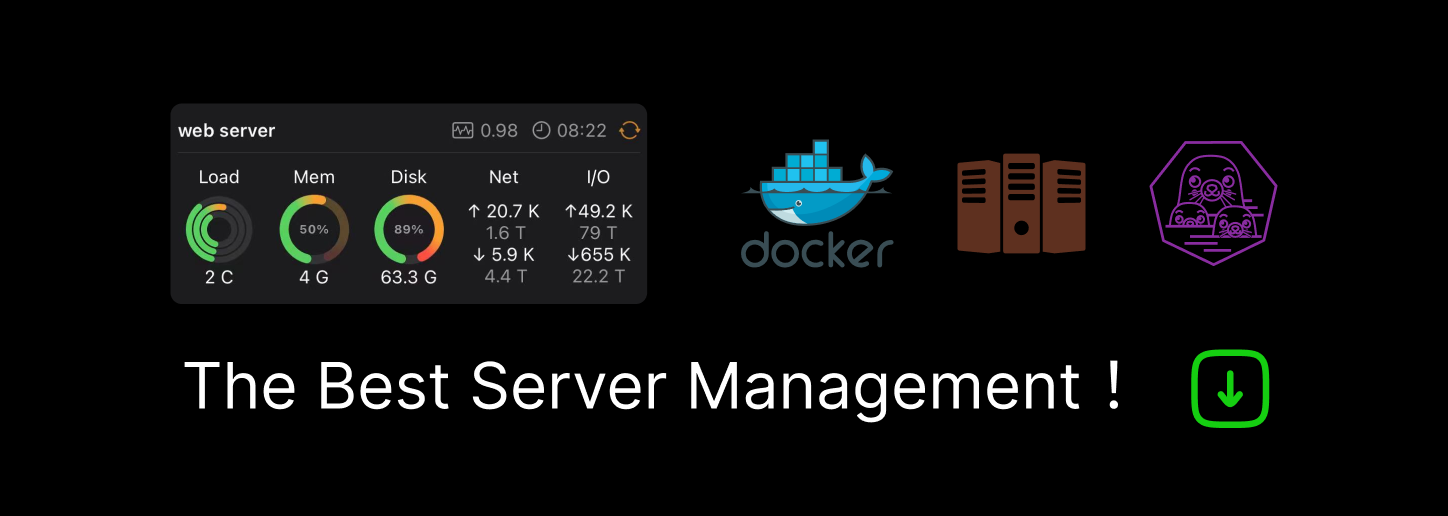
痛点
在使用Spring mvc 进行开发时我们经常遇到前端传来的某种格式的时间字符串无法用java8时间包下的具体类型参数来直接接收。同时还有一系列的序列化 、反序列化问题,在返回前端带时间类型的同样会出现一些格式化的问题。今天我们来彻底解决他们。
建议
其实最科学的建议统一使用时间戳来代表时间。这个是最完美的,避免了前端浏览器的兼容性问题,同时也避免了其它一些中间件的序列化/反序列化问题。但是用时间表达可能更清晰语义化。两种方式各有千秋,如果我们坚持使用java8的时间类库也不是没有办法。下面我们会以`java.time.LocalDateTime` 为例逐一解决这些问题。
局部注解
网上有很多文章说该注解是前端指向后端的,也就是前端向后端传递时间参数格式化使用的,这没有错!但是有一个小问题,该方式只能适用于不涉及反序列化的情况下。也就是以下场景才适用:
@GetMapping("/local")
public Map<String, String> data(@DateTimeFormat(pattern = "yyyy-MM-dd HH:mm:ss") LocalDateTime localDateTime) {
Map<String, String> map = new HashMap<>(1);
map.put("data", localDateTime.format(DateTimeFormatter.ofPattern("yyyy-MM-dd")));
return map;
}
如果你在下面这个场景使用就不行了:
@Data
public class UserInfo {
@DateTimeFormat(pattern = "yyyy-MM-dd HH:mm:ss")
private LocalDateTime birthday;
private String name;
private Integer age;
}
@PostMapping("/user")
public Object postData(@RequestBody UserInfo userInfo) {
System.out.println("userInfo = " + userInfo);
return userInfo;
}
原因是Post请求参数在body中,需要反序列化成对象。默认是jackson类库来进行反序列化,并不触发`@DateTimeFormat`注解机制。
这时我们就需要使用jackson的格式化注解`@JsonFormat`。我们将实体类`UserInfo`改造成下面的就可以了:
@Data
public class UserInfo {
@DateTimeFormat(pattern = "yyyy-MM-dd HH:mm:ss")
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss", timezone = "GMT+8")
private LocalDateTime birthday;
private String name;
private Integer age;
}
以上两个注解可以并存,但是一定要清楚各自的使用场景。这里还有一个小细节:格式一定要对应好时间类型。比如`yyyy-MM-dd` 对应`java.time.LocalDate` 。如果再个性化一些`@JsonFormat` 可以被`@JsonDeserialize`和`@JsonSerialize` 代替。但是它们的`using`参数需要你自己实现为你对应的时间类型类型。如果`@JsonFormat`、`@JsonDeserialize`和`@JsonSerialize`同时存在`@JsonFormat`的优先级要更高。
局部注解的好处
局部处理的好处在于八个字:百花齐放,百家争鸣 。可以保持多样性、个性化 。但是局部带来了一个新的问题 :没有共同的标准 、不兼容。进而不方便维护。所以有时候基于业务需要我们全局化可以统一管理。下面我们将讲解如何进行全局化配置。
全局配置
全局化其实也是基于 `@DateTimeFormat` 和`@JsonFormat` 两种场景来进行配置。对于`@DateTimeFormat`的场景我们通过实现Spring提供的接口:
DateTimeFormatter :
// 时间格式化
private static final DateTimeFormatter FORMATTER = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss", Locale.CHINA);
类型转换接口:
org.springframework.core.convert.converter.Converter<S,T>
实现:
@Bean
public Converter<String, LocalDateTime> localDateConverter() {
return new Converter<String, LocalDateTime>() {
@Override
public LocalDateTime convert(String source) {
return LocalDateTime.parse(source, FORMATTER);
}
};
}
或者格式化接口:
org.springframework.format.Formatter<T>
实现 :
@Bean
public Formatter<LocalDateTime> localDateFormatter() {
return new Formatter<LocalDateTime>() {
@Override
public LocalDateTime parse(String text, Locale locale) throws ParseException {
return LocalDateTime.parse(text, FORMATTER);
}
@Override
public String print(LocalDateTime object, Locale locale) {
return object.format(FORMATTER);
}
};
}
以上两个接口的实现都要注册为Spring Bean,配置的时候二者选其一即可,其中S即Source也就是来源,其实就是前端的时间字符串。T即Target也就是目标,代表你需要转化或者格式化的时间java类型。 那么对于时间序列化和反序列化我们进行如下配置就行了(基于默认jackson,以LocalDateTime 为例):
@Bean
public Jackson2ObjectMapperBuilderCustomizer jackson2ObjectMapperBuilderCustomizer() {
return jacksonObjectMapperBuilder -> jacksonObjectMapperBuilder
// 反序列化
.deserializerByType(LocalDateTime.class, new LocalDateTimeDeserializer(FORMATTER))
// 序列化
.serializerByType(LocalDateTime.class, new LocalDateTimeSerializer(FORMATTER));
}
同样该jsonMapper自定义构建器要注册成Spring Bean才行。
全局配置的要点
全局配置的一些优缺点上面已经阐述了,这里我还是要啰嗦一下要点避免你踩坑。全局配置跟局部配置一样。同样要约定pattern。这就要求我们全局保持一致。我们可以实现多个以上的全局配置来对其他诸如`LocalDate`、`OffsetDateTime` 的适配。同时如果我们接入了其它一些需要用到序列化/反序列化的中间件,比如redis、rabbitmq,我们也要注意进行适配。
总结
总结通过以上对时间格式的局部和全局处理方式的介绍,相信困扰你的Spring mvc 时间问题不会再存在了。如果感觉写的可以请转发告诉其他同学,点个赞,关注一下。
demo 地址: https://gitee.com/felord/datetime-convert
喜欢我可以给我设为星标哦
好文章,我 在看

Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK